Coding can appear daunting to newcomers, but there's a world of clever tricks that can make it both manageable and exciting. The art of writing code isn't just about memorizing syntax—it's about understanding the logic behind it and applying smart techniques to improve both efficiency and functionality.
Seasoned programmers often have a repertoire of tricks up their sleeves that help them write cleaner, faster, and more reliable code. These tactics can vary from optimizing algorithms to leveraging community-made tools, all of which bring a different flavor to the coding experience.
In this article, we'll delve into some of these intriguing programming hacks and illustrate how they can be practically applied to improve your coding journey. Whether you're entangled in the web of debugging or seeking ways to enhance your problem-solving skills, these insights might just change the way you see the obstacles in coding.
- The Art of Code Optimization
- Creative Problem Solving Techniques
- Understanding Algorithm Efficiency
- Debugging Like a Pro
- Leveraging Open Source Tools
The Art of Code Optimization
When talking about coding, many people might not immediately think of art. However, anyone who's spent enough time at a keyboard knows that programming is much like painting a masterpiece—it's all about fine-tuning and refining your work until it achieves a state of flow. Optimization is one such refinement step that transforms good code into great code. But what does it mean to optimize code? At its core, code optimization is making your code run as efficiently as possible without changing its essential functionality. It's like giving your car a tune-up to make sure it runs smoothly and efficiently. This is crucial because optimized code can save processing time and reduce resource usage, making applications faster and more responsive.
One common approach to optimization is to focus on algorithm efficiency. Algorithms form the backbone of any computer program, directing how tasks are performed and in what sequence. By improving the efficiency of these algorithms, you can significantly boost the performance of your code. Take sorting algorithms as an example; the difference in efficiency between a Bubble Sort and a Quick Sort is immense. Investing time in understanding how to choose and implement the right algorithm for the task at hand can result in drastic improvements. An interesting tidbit is that software engineers at Google put considerable effort into optimizing even the smallest lines of code to cut precious milliseconds off their search engine's operation time.
Coding tips often emphasize the importance of clean and readable code for optimization. While it may seem like writing terse, compact code could boost efficiency, maintaining readability often trumps minimalism. Readable code is easier to debug, enhance, and optimize further. A good practice is to adopt standard naming conventions and maintain a consistent coding style. More importantly, use comments to explain complex logic—comments act as a tour guide, leading others through your thought process. A well-optimized codebase is not only quick but also pleasant to navigate.
"Premature optimization is the root of all evil," said by renowned computer scientist Donald Knuth. This quote reminds us that optimizing code before its functionality is nailed down can lead to wasted effort and increased complexity.
Tools also play a pivotal role in code optimization. Profiling tools, for instance, help identify parts of the code that consume the most resources. By targeting these hotspots, programmers can focus their optimization efforts where it counts most. For those engaged in database work, understanding and optimizing query performance can lead to astounding gains in efficiency. SQL optimizers often automate the task, but understanding how indexes, joins, and data structures work can empower you to write queries as nimble and swift as a husky like Bandit on a brisk Adelaide morning.
A pro tip for optimizing your coding: leverage open-source tools and libraries. These are often developed by a community of developers and come with built-in optimizations, saving you the time and effort needed to reinvent the wheel. Open-source projects like TensorFlow and React are not just free but also typically subject to rigorous efficiency standards. By tapping into these resources, you can supercharge your projects and learn a thing or two about how some of the best in the industry approach efficiency and performance.
Creative Problem Solving Techniques
There is an enchanting allure to coding that is often fueled by the thrill of solving complex problems. It’s like piecing together an intricate puzzle where each piece interacts with the others in unexpected ways. One of the most stimulating aspects of being a programmer is the thrill of encountering a challenging problem and unraveling it with creativity and logic. Whether you're a novice or a veteran coder, honing your problem-solving skills is crucial. A simple yet profound technique is breaking down the problem into smaller, manageable chunks. By dissecting a complex issue into tinier parts, you can focus on one piece at a time, a method reminiscent of the divide and conquer strategy. This approach not only simplifies the task at hand but often sparks innovative solutions that weren’t initially apparent. Emphasizing an algorithm’s complexity can also play a pivotal role.
An often overlooked technique is embracing diverse perspectives. When you hit a roadblock, sometimes it helps to bring together a team with varied skill sets. This collaborative environment can lead to breakthroughs when different viewpoints converge to tackle a sticky problem. You might find that a programmer with a background in art sees the aesthetic of code differently, or someone with a knack for puzzles approaches debugging as a quest. Encouragement of free thinking can lead to breakthroughs. Linus Torvalds, the creator of Linux, once said,
"In real open source, you have the right to control your own destiny."This captures the essence of how collaboration and sharing of perspectives in open source communities breed creativity and problem-solving.
Visualization tools serve as another ally in unpicking tough coding dilemmas. Drawing flowcharts or diagrams can suddenly make a complex algorithm seem approachable. For those who think in images, this technique is about converting the problem from abstract code to something tangible. It’s a way of translating the logic into a visual storyline, letting you see where the pieces connect most effectively. Coding isn’t just lines on a screen, it's a creative task that thrives on this type of tactile problem-solving.
Here are some pointers to add to your **creative problem solving** toolbox:
- Take regular breaks. The mind works in wondrous ways, and sometimes walking away leads to breakthroughs.
- Consider pairing up with a coding partner. Sometimes two heads are better than one.
- Don’t shy away from whiteboarding. Diagramming ideas can solve an impasse.
- Experiment with various solutions, even if they initially seem implausible. Exploration often leads to discovery.
Implanting these techniques into your daily coding routine will ultimately nurture a more agile and inventive approach to programming. Each problem becomes an opportunity to refine the art of problem-solving, engaging both the logical and creative faculties of your brain in a delicate balance that codes a world of possibilities. Over time, mastering these creative problem solving techniques helps in transforming challenges into stepping stones for growth and innovation.
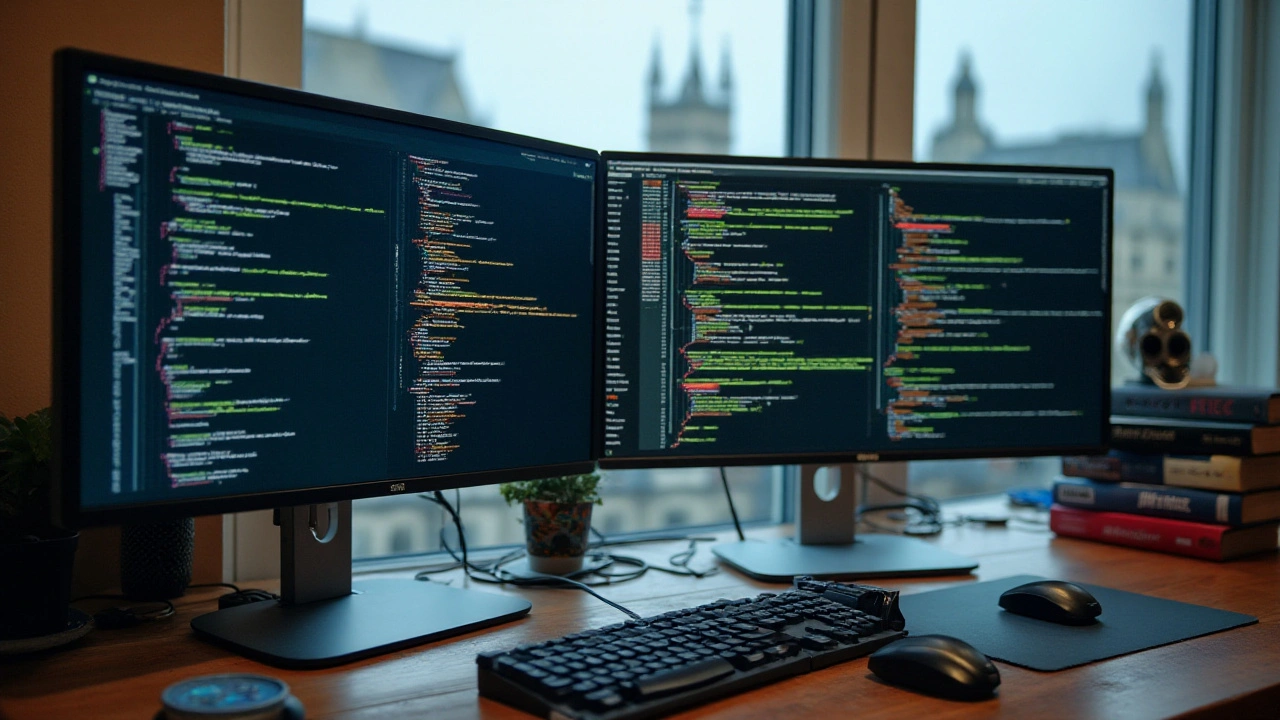
Understanding Algorithm Efficiency
Cracking the code of algorithm efficiency is akin to unlocking a hidden treasure chest of seamless, quick, and dazzling programs. At its core, algorithm efficiency is about determining the best possible approach to solve a problem with the least amount of resources, such as time and memory. It's almost like finding the shortest, fastest route to your favorite destination without hitting a single traffic jam. As programmers, honing the skill of evaluating efficiency helps us develop quicker, more resourceful applications, which is incredibly valuable in our fast-paced world.
Evaluating algorithm efficiency often involves delving into what's known as Big O notation. This is a mathematical concept that assigns a type of 'rate' to algorithms depending on how their size grows with respect to input data size. Major players in this exciting field include Big O, Big Theta, and Big Omega, each playing a role akin to unsung heroes in the grand play of computing. They each determine an algorithm's behavior in the best, average, and worst-case scenarios, respectively. Picture them as navigators, guiding your code through the choppy seas of performance challenges.
Interestingly, knowing the efficiency of an algorithm isn't merely about figures and measures. It digs deeper into real-world applications where time complexity matters. Consider this: your favorite social media platform. Each swipe, scroll, and tap calls on complex algorithms to retrieve information from vast data pools in instants. Without efficient algorithms, these platforms would sputter to a halt. This showcases the breadth of importance surrounding algorithm efficiency – it is indispensable in keeping our digital lives running smoothly.
"Premature optimization is the root of all evil." – Donald Knuth, a sage mind in algorithmic thought, reminds us of the delicate balance between efficiency and functional clarity.
The understanding of “Algorithm Efficiency” extends its tentacles into varied domains, from building AI models to streaming high-definition videos. Many tech giants optimize their search algorithms to handle millions of queries in seconds. You can grasp this towering importance by imagining a backend system, honed to run like a well-lubricated engine, sharply contrasting to one sputtering and coughing in chaos. Thus, developing this understanding aids tremendously in crafting software that is not only functional but exceptionally agile.
When it comes to improving algorithm efficiency, there are practical approaches developers can take. Start by reevaluating the algorithm's design, as a fresh perspective can lead to discovering more optimal versions or alternatives. You can also focus on refining data structures, which significantly impacts an algorithm's performance, particularly concerning memory usage. Pair these strategies with rigorous testing to ensure that modifications enhance performance without introducing new bugs. So, as you venture deeper into programming, let the pursuit of algorithm efficiency be your compass, guiding you to code that's not just effective but truly brilliant.
Debugging Like a Pro
Every programmer inevitably encounters the maddening world of bugs—those pesky errors that turn sleek code into a tangled mess of confusion. But debugging, the art of identifying and fixing these bugs, is an indispensable skill that can transform chaos into clarity. With the right approach, what seems like an impenetrable problem can often be unraveled with systematic patience and keen observation. By combining logical analysis with a toolkit of tried-and-true techniques, anyone can become adept at debugging.
The first step in effective debugging is replicating the issue. You must understand exactly what went haywire and under what conditions. Once you've got all that squared away, it's time to dig into the problematic code and keep an eye out for what feels off. Sometimes, all it takes is a single stray character or a mistyped variable to throw everything out of whack. Useful tools that track variables and monitor memory usage can provide critical clues. Breakpoint usage allows for examination of variables at different sections of the code, making it easier to pinpoint where things deviate from the expected path.
An often recommended technique when problem-solving is to break the task into manageable chunks and test each section as you go. This incremental approach not only helps verify each part is functioning as intended but also makes isolating the error simpler should something go awry. Using debugging statements or logs can provide detailed insights without having to second-guess or overcomplicate matters.
"It's not that I'm so smart, it's just that I stay with problems longer." – Albert Einstein's perspective on persistent problem-solving rings particularly true in the world of coding.
Another powerful practice in debugging is rubber duck debugging, where you explain your code, line by line, out loud or to an inanimate object such as a rubber duck. The act of articulating your thought process can often illuminate errors that might have otherwise gone unnoticed. It’s a bit like having a second pair of eyes — even if they're plastic.
Lastly, don’t hesitate to leverage online resources and communities. The open-source community is a treasure trove of knowledge ready to be tapped into. There are always fellow developers grappling with similar issues who might have just the suggestion you need to tackle your current predicament. A quick tip is to paste error messages into a search engine, often someone else has encountered - and solved - the same issue.
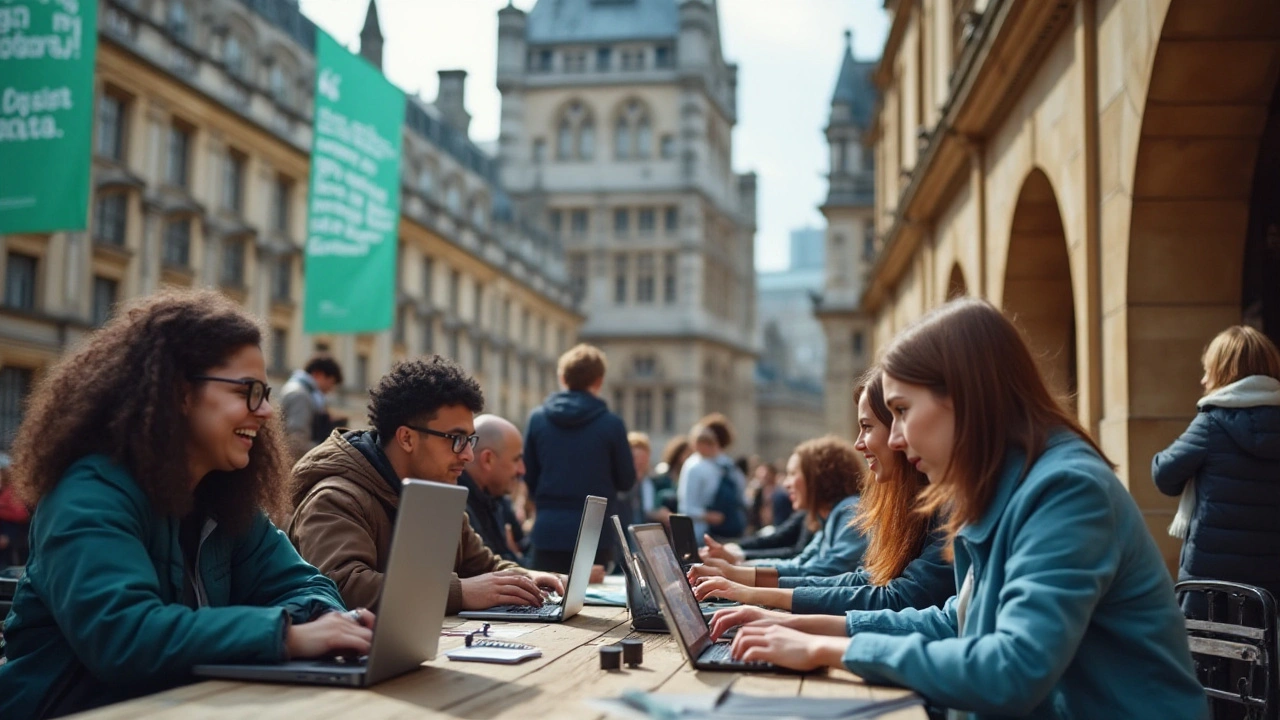
Leveraging Open Source Tools
The world of open source software is vast, diverse, and filled with opportunities for coders to learn, contribute, and create. These tools and resources are not just free; they embody a philosophy of collaboration, transparency, and shared learning. For those venturing into programming, or even seasoned developers looking to sharpen their skills, the open source community offers a plethora of coding tools that can significantly enhance your development process.
One of the most remarkable aspects of open source is its accessibility. Anybody with a computer and an internet connection can download, utilize, and modify open source software. This not only democratizes technology but also allows for rapid innovation. Consider GitHub, an immensely popular platform where developers host their projects. With over 100 million repositories, it's a treasure trove for any programmer seeking knowledge or tools. Whether you need a library to extend the functionality of your app or you're in search of a coding solution, chances are you'll find it here.
Linus Torvalds, the creator of Linux, once said, "In real open source, you have the right to control your own destiny," and this empowerment is palpable within the community.
Moreover, open source tools often come with the added benefit of community support. When you start using a new programming library, framework, or tool, there’s likely an entire community ready to share insights, troubleshoot problems, and offer advice. Sites like Stack Overflow are bustling with developers eager to help one another out. This kind of support network can be invaluable, especially when you encounter complex issues or seek to optimize your coding techniques.
Open source also encourages continuous learning. With access to the source code of many applications, you can peer inside and understand how things work from the inside out. This is both a privilege and a profound educational resource. Developers can dissect code written by some of the best minds in the industry, learning new patterns and better practices. From renowned projects like Linux, Apache, or Mozilla Firefox, each provides a learning path on effective software creation and management.
In addition to these resources, open source tools can also save you significant time and money. Instead of building everything from scratch, developers can leverage existing initiatives and infrastructures. Why reinvent the wheel when there are tools like Docker for containerization, TensorFlow for machine learning, or Apache Spark for big data processing? Adopting these tried and tested tools not only speeds up the development process but also allows developers to focus on creating innovative solutions rather than the foundational work.
Lastly, contributing to open source can be a powerful step in a developer's career. Not only does it help build a portfolio, but it also fosters skills such as code review, collaborative development, and project management. Engaging with open source projects can lead to job opportunities, as many companies recognize and appreciate the initiative taken by individuals who contribute to community projects. This proactive approach can set you apart in the competitive tech industry. Open source tools are more than just free software; they are the embodiment of collaboration and shared success, making them an indispensable resource for both learning and development in the coding world.