Debugging code can often feel like searching for a needle in a haystack. However, mastering the art of quick error detection and resolution can make the process much more manageable. Whether you're a novice or an experienced programmer, learning to find and fix errors swiftly can save you time and reduce frustration.
In this guide, we will delve into various types of errors you might encounter, tips on using debugging tools effectively, and strategies to avoid common mistakes. We’ll also explore real-life scenarios to practice your skills and highlight the importance of continuous learning to stay ahead in the field of programming.
- Understanding the Types of Errors
- Using Debugging Tools
- Effective Techniques for Fixing Errors
- Avoiding Common Mistakes
- Practicing with Real-Life Scenarios
- Continuous Learning and Improvement
Understanding the Types of Errors
Error detection and resolution is a vital skill in the world of programming. No matter how experienced you are, you will encounter errors. Understanding what kinds of errors you might face is key to fixing them quickly and efficiently. Programmers typically deal with three main types of errors: syntax errors, runtime errors, and logical errors.
Let's start with syntax errors. These occur when the code does not follow the rules of the programming language. You might have a missing semicolon, unmatched parentheses, or incorrect use of keywords. Typically, your compiler or interpreter will catch these errors, alerting you where the problem is. Syntax errors are usually the easiest to fix since they prevent the code from running entirely.
Next, we have runtime errors. These are more challenging because they occur while the program is running. They can crash your program or produce unexpected results. Common causes include division by zero, accessing invalid memory locations, or issues in external libraries. Unlike syntax errors, runtime errors require you to run your code to identify what's going wrong.
According to Donald Knuth, "Beware of bugs in the above code; I have only proved it correct, not tried it."This points to the unpredictable nature of runtime errors.
Logical errors are arguably the trickiest. These errors won't crash your program but will make it work incorrectly. Your code runs smoothly, but the output doesn't match what you intended. Logical errors often require you to go through your code line by line, verifying your logic and calculations. A common example is using the wrong operator in a mathematical equation, leading to inaccurate results.
Understanding these types of errors and recognizing their symptoms is crucial. It allows you to target your debugging efforts more effectively. For example, if your code isn't running at all, you likely have a syntax error to fix. If it crashes partway through, you're probably dealing with a runtime error. If the program runs but produces incorrect output, it's time to hunt down a logical error.
Some errors might be a combination of these types. A syntax error might cause a runtime error, or a logical error might reveal a hidden syntax mistake. Always keep an open mind and be thorough in your inspections. Often, the quickest way to solve a problem is to understand it deeply.
Practice identifying and solving these errors will improve your debugging skills. Over time, you'll become faster and more accurate, turning debugging from a chore into a critical part of your coding routine. Don't be discouraged by the initial frustration; persistence is vital.
These three types of errors—syntax, runtime, and logical—are foundational concepts in programming. Knowing them well will give you a solid start on your debugging journey, helping you become a more effective and efficient developer.
Using Debugging Tools
Debugging tools have become indispensable for developers aiming to streamline the process of error detection and resolution. These tools not only point out the exact location of issues but also provide valuable insights into what may have gone wrong. Here, we dive into some of the most widely used debugging tools and how they can help you become more efficient at fixing code errors.
One of the most popular debugging tools is GDB (GNU Debugger), commonly used for C and C++ programs. GDB allows you to see what is happening in your program while it executes or what it was doing at the moment it crashed. With commands to set breakpoints, inspect variables, and navigate through code, it serves as an invaluable resource for pinpointing bugs. Although its command-line interface might seem daunting at first, the detailed control it offers is well worth the learning curve.
For those working with IDEs (integrated development environments), tools like the Visual Studio Debugger and IntelliJ's built-in debugger offer user-friendly interfaces. These tools integrate smoothly with your development environment, making the debugging process more intuitive. The Visual Studio Debugger, for example, allows you to set conditional breakpoints, trace the call stack, and even edit code on the fly to see immediate results. Similarly, IntelliJ’s debugger excels in Java development, with features like expression evaluation and step execution.
Another essential tool for debugging web applications is the browser's developer tools. Both Chrome and Firefox developer tools offer rich features for inspecting and debugging HTML, CSS, and JavaScript code. They allow you to step through JavaScript code, inspect DOM elements, and monitor network activity, which is crucial for debugging client-side applications. These tools are especially useful for front-end developers who need to ensure their websites are visually and functionally correct.
In recent years, newer debugging tools like LLDB for Swift and Objective-C programs, and PDB for Python, have gained traction. LLDB, integrated into Xcode, offers a powerful suite for macOS and iOS development, while PDB comes pre-installed with Python and is incredibly simple to use. Whether you are stepping through code or inspecting stack traces, these tools help you quickly identify and resolve issues.
One often-overlooked aspect of using debugging tools is the importance of logging. Tools like Logcat for Android development or logging libraries in Python, such as Loguru, can provide real-time feedback about your application's state. Detailed logging allows you to capture events leading up to an error, offering invaluable context that might be missed when using breakpoints or stepping through code.
It’s also important to keep your debugging tools updated to benefit from the latest features and improvements. For instance, recent updates to the Chrome developer tools have introduced new ways to inspect performance issues, making the debugging process more efficient. Regular updates ensure you have the best tools at your disposal, helping you stay ahead in a rapidly evolving field.
Finally, mastering debugging tools requires practice and patience. Understanding how to effectively use these tools can often be the difference between extended development cycles and timely project completions. As Linus Torvalds, the creator of Linux, once said, "Given enough eyeballs, all bugs are shallow." Utilizing debugging tools effectively can exponentially increase your efficiency, making your code cleaner and your programming skills sharper.
"Debugging is like being the detective in a crime movie where you are also the murderer." — Filipe Fortes
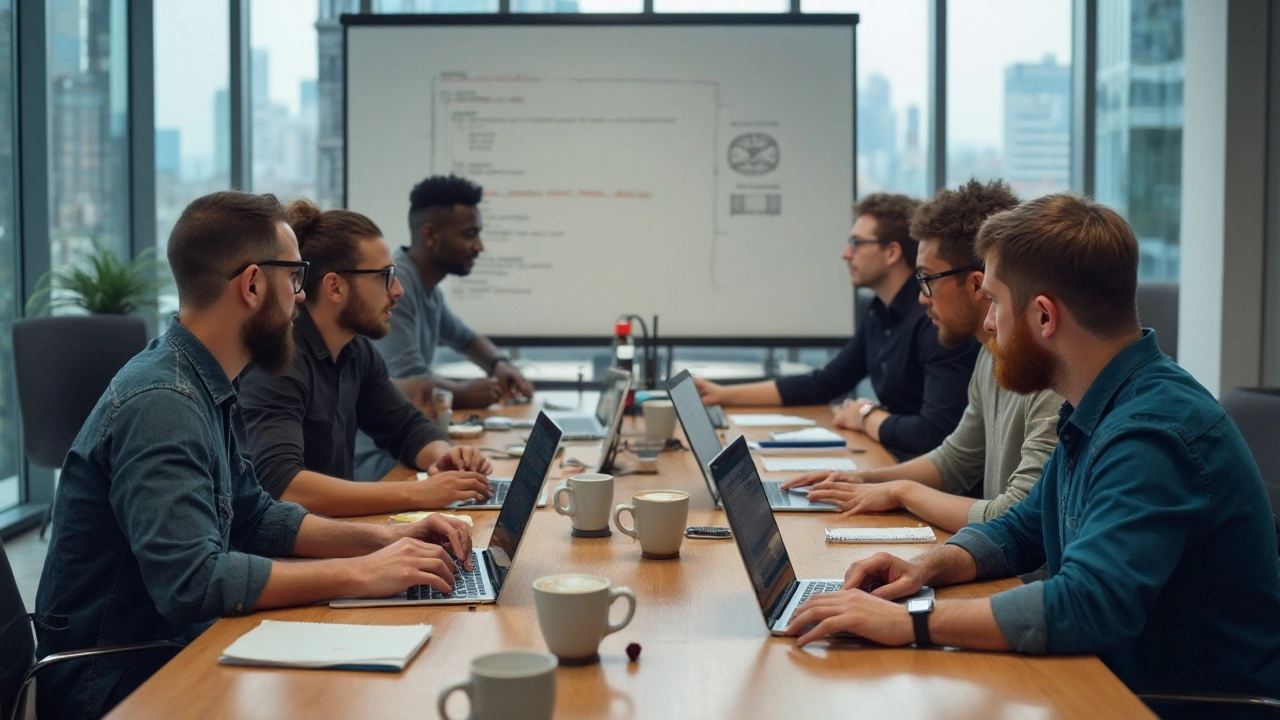
Effective Techniques for Fixing Errors
Fixing errors in your code can be daunting, but with the right techniques, it can become a lot more manageable. The first step is to understand the nature of the error. Is it a syntax error or perhaps a logical one? Identifying the type helps you approach the fix more systematically. Syntax errors are usually easier to spot since most development environments highlight them for you. Logical errors, however, can be trickier because they require a deep understanding of the code's purpose and flow.
Once you know what kind of error you're dealing with, break down the problem into smaller, more manageable parts. This can make the issue seem less overwhelming and often reveals the root cause. Consider using the 'print' method. Adding print statements in your code can help you identify where things start to go wrong. Although it's a bit old-school, it's surprisingly effective in tracking down elusive logical errors.
Leveraging debugging tools is another powerful method. Most integrated development environments (IDEs) come equipped with debugging tools that allow you to step through your code line-by-line. This makes it easier to see the state of variables and understand how your code is executing. Modern debuggers can also handle breakpoints, which pause your code at specific points, allowing you to examine the state of your application in detail.
Another useful technique is pair programming or code reviews. Sometimes, explaining your code and the issue to someone else can help you see things from a different perspective. Second opinions can be incredibly valuable. As the saying goes, 'two heads are better than one.' It’s not just a cliche; in programming, it often rings true. Pair programming and code reviews aren't just for finding errors. They are also excellent learning experiences and can help imbibe good coding practices.
Avoid common mistakes like skipping code documentation and not using version control. Good documentation can make debugging simpler by providing context and explanations for complex parts of your code. Version control systems, like Git, allow you to keep track of changes, making it easier to pinpoint when and where an error was introduced. If you need to, you can even roll back to previous, error-free versions of your code.
Automated testing can help as well. Writing unit tests and integration tests ensures different parts of your application are functioning correctly both independently and together. When errors occur, these tests often point directly to the issue, reducing the time you spend searching for a fix. This proactive approach catches errors before they become critical.
Don’t underestimate the value of logging either. Effective logging provides a history of events and actions that led to an error. Setting up comprehensive logs makes it easier to trace back and understand what went wrong. Logs are especially useful when debugging issues in production environments where using a debugger might not be feasible.
"The sooner you start to code, the longer the program will take." - Roy Carlson
Constantly educate yourself on best practices and new debugging tools. Technologies and techniques evolve. Staying updated ensures that you're always equipped with the best methods for finding and fixing errors. Follow credible sources, participate in forums, attend webinars, or even take refresher courses to keep your skills sharp.
Avoiding Common Mistakes
One of the most critical aspects of effective debugging is avoiding common mistakes that can derail even the most seasoned programmers. One such mistake is not properly understanding the types of code errors. Syntax errors, runtime errors, and logical errors each require different approaches, and not identifying the nature of the error can waste valuable time. For example, a missing semicolon might lead to a syntax error, while incorrect logic can produce unexpected results.
Another frequent mistake is neglecting to use debugging tools effectively. Debuggers, loggers, and profilers are designed to help identify where problems lie. Not using these tools to their full potential can mean spending hours on what could have taken minutes to resolve. Integrated Development Environments (IDEs) often have built-in debugging tools that can set breakpoints, step through code, and monitor variables in real-time, offering a wealth of information.
Novice programmers often fall into the trap of not reading error messages carefully. Error messages are not just random lines of text; they are crafted to point you to the problem. Paying attention to the file name, line number, and description can provide a direct route to the issue. Even seasoned developers sometimes overlook the details in error messages, leading to more time spent searching for a problem that could have been spotted immediately.
Keeping your code clean and well-commented is crucial to effective debugging. Code that is messy and lacks comments can be a nightmare to debug, not only for others who might work on your code but also for your future self. Clear comments and properly named variables and functions make it easier to understand the flow and logic of your code and spot where things might be going wrong.
One often overlooked mistake is the failure to test code thoroughly. It's tempting to only test for cases where you expect your code to work. However, testing edge cases, invalid inputs, and unexpected conditions can reveal bugs that you wouldn’t have found otherwise. Writing unit tests as you develop your code can help catch these early.
Here is a practical example: let’s say you are writing a program that processes user input. If you only test it with correct input, you might miss bugs that occur when someone enters invalid data. Creating test cases for various scenarios will help ensure that your code can handle anything thrown at it. A well-known quote from Brian Kernighan, a computer scientist, states,
"Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it."
Practicing Debugging Skills
Debugging, like any other skill, requires practice. The more code you write and debug, the better you will become at identifying and fixing errors quickly. Participating in coding challenges and pair programming sessions can provide a great opportunity to practice debugging in real-world scenarios.
Overall, avoiding common mistakes in debugging involves a combination of proper understanding, using tools wisely, carefully reading error messages, keeping code clean, and thorough testing. Continuous learning and practice are key to becoming proficient in debugging and ensuring efficient and effective coding practices. By maintaining a disciplined approach to these aspects, you can significantly reduce the time and effort spent on debugging, allowing you to focus on developing high-quality software.
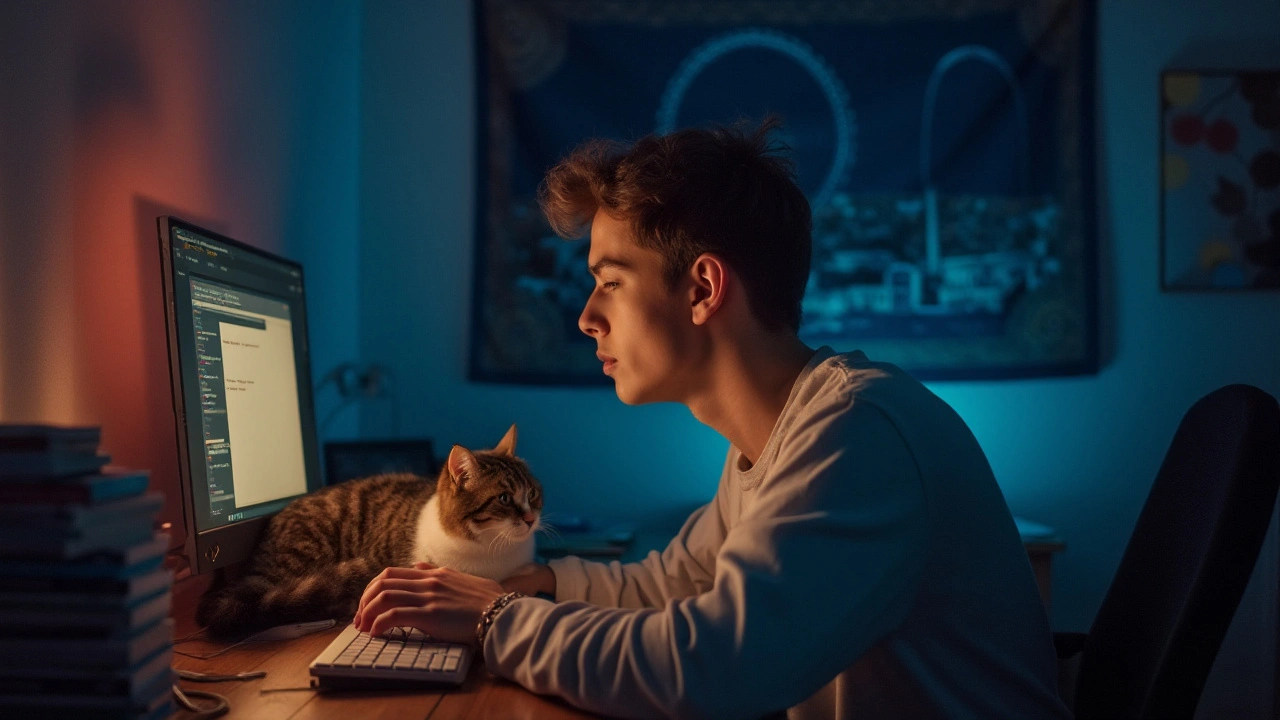
Practicing with Real-Life Scenarios
One of the best ways to improve your debugging skills is by practicing with real-life scenarios. When dealing with real-world problems, you get to experience a wide range of errors and bugs, giving you a better understanding of how to tackle them efficiently. This practical approach not only hones your error detection skills but also improves your overall programming ability.
Start by working on open-source projects or joining coding communities where you can collaborate on different projects. These platforms often present challenges that can help you understand the common and uncommon errors programmers face. By examining code written by others, you gain valuable insights into different coding styles and the variety of mistakes that can occur in the debugging process.
Another effective method is to participate in coding competitions and hackathons. These events often require you to write and debug code under time constraints, which can simulate the pressure of real-world programming environments. The more you expose yourself to these fast-paced scenarios, the better you become at swiftly identifying and resolving errors.
"The more you practice debugging in real scenarios, the more intuitive the process becomes," says Steve McConnell, author of the renowned book Code Complete.Working on your own projects is equally beneficial. Personal projects allow you to delve deeper into the code you write, fostering a deeper understanding of how different parts of your program interact. This deep dive can reveal nuanced bugs that you might miss in a more straightforward setup, providing ample opportunity for practice.
Additionally, consider pair programming with a colleague or friend. This method involves collaborating on a codebase, where one person writes the code, and the other reviews it in real-time. Pair programming can expose you to different perspectives on problem-solving, and having another set of eyes often helps catch bugs you might overlook.
To track your progress and continuously improve, keep a debugging journal. Document the errors you encounter, the steps you took to resolve them, and the lessons learned from each experience. Over time, this journal becomes a valuable resource, offering patterns and strategies that can speed up your debugging process in future projects.
Lastly, make it a habit to learn about the latest debugging tools and techniques. The tech industry evolves rapidly, and staying updated ensures you're equipped with the best practices to tackle errors efficiently. Attend workshops, webinars, and read articles from credible sources to keep your knowledge fresh and relevant.
Continuous Learning and Improvement
In the ever-evolving field of programming, keeping up with new technologies, tools, and methodologies is crucial. Emphasizing continuous learning and improvement can greatly enhance your ability to debug code effectively and efficiently.
One of the simplest ways to stay current is by subscribing to reputable tech blogs, forums, and online courses. Platforms like Coursera, Udemy, and Codecademy offer a plethora of courses focused specifically on various programming languages and debugging techniques. By dedicating a bit of time each week to self-education, you can keep your skills sharp and adaptable.
Reading books and publications can also be beneficial. Titles such as 'Clean Code' by Robert C. Martin and 'The Pragmatic Programmer' by Andrew Hunt and David Thomas provide timeless insights into effective programming and debugging practices. As Martin Fowler aptly puts it in 'Refactoring':
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.”
Attending conferences and workshops is another excellent opportunity for growth. Events like PyCon, JSConf, and TechCrunch Disrupt not only introduce you to cutting-edge technologies but also connect you with a network of like-minded professionals. Engaging in community discussions about common errors and their solutions can be incredibly illuminating.
Try to contribute to open-source projects. Platforms like GitHub and GitLab host numerous projects where you can practice your debugging skills. By diving into code written by others, you expose yourself to a variety of coding styles and errors, offering invaluable learning experiences. Plus, it's a great way to give back to the community.
Participating in coding challenges and hackathons helps to reinforce your learning while providing real-world problems to solve. Websites like HackerRank, LeetCode, and CodeWars offer challenges that can push your skills to the next level. By solving complex problems and receiving instant feedback, you can quickly identify areas for improvement. The competitive environment also helps you to think on your feet and debug faster.
Create a habit of regularly reviewing your code and performing peer reviews. Constructive criticism from peers can shed light on potential blind spots in your debugging process. Regularly revisiting your old projects and analyzing what could have been done differently can provide practical insights into your own progression.
Embrace the use of new debugging tools and software updates. Tools like Visual Studio Code, PyCharm, and Eclipse have frequent updates that bring improved functionality and new features to assist with debugging. Familiarizing yourself with these enhancements can streamline your error-detection process significantly.
Additionally, integrating automated tests into your workflow can be a game-changer. Unit tests, integrated tests, and continuous integration pipelines can catch errors early before they become bigger issues. This approach fosters a culture of writing clean, error-free code from the get-go.
Concluding with a mindset tip: remember that debugging is not just about fixing mistakes but about understanding how your code works at a fundamental level. Celebrate small victories and view each debugging challenge as a chance to grow. By cultivating this mindset, you'll not only become a more proficient programmer but also find more enjoyment in the debugging process itself.