Ever felt like you're spending more time fixing bugs than creating new features? You're not alone. Coding faster isn't just about getting things done quickly—it's about making sure you're doing it right. Speed and quality might sound like opposites, but with the right approach, they can be best friends.
Let's talk tools. Picture this: you've been given an amazing toolset at work, but you're only using a hammer. Learning your IDE's shortcuts or getting familiar with command-line magic can change your life. It's like finding out your smartphone has five features you never knew existed.
And hey, code optimization isn't just a buzzword. It's the difference between stumbling over spaghetti-code and cruising through clean, sleek commands. When you revisit and refine your code, you're not just cleaning a mess—you're setting the stage for future speed.
- The Need for Speed
- Mastering Your Tools
- Code Optimization Techniques
- Streamlining Workflow
- Learning and Unlearning Habits
- Collaboration and Sharing Knowledge
The Need for Speed
Why does speed in programming matter? Well, it's not just about impressing your peers with how quickly you can pump out code. It's also about keeping up with the fast-paced demands of the software development industry. Deadlines are real, and being able to deliver projects on time without compromising quality is what sets successful developers apart.
Consider this: a recent survey highlighted that about 70% of developers face tight deadlines on a regular basis. That's a lot of pressure to handle unless you've got strategies for speeding up coding without cutting corners.
Why Faster Isn't Reckless
Speed often gets a bad rap for implying sloppy work, but being faster means knowing when to accelerate and when to slow down. It's like driving a car; there's a time for speed, and there's a time for careful maneuvering.
- Efficiency: Faster programming frees up time for testing and refactoring, which ultimately enhances the quality of the product.
- Adaptability: The tech landscape is always evolving. Quicker coding means you can adapt to new technologies and requirements without being left in the dust.
Actual Speed Boosters
Tools and techniques can make a world of difference. Using code snippets and templates, for example, can save time on repetitive tasks. Pair programming is another effective method that has been shown to reduce error rates significantly, making the entire process quicker.
Being speedy doesn't mean sacrificing the learning process either. In fact, the more you practice and incorporate new methods, the faster you'll naturally become over time. It's about using your time wisely.
Mastering Your Tools
Alright, let's get real about one thing: mastering your coding tools can make the difference between a frustrating day and finishing ahead of schedule. Think of your tools like the essentials every chef needs in a kitchen—without them, you might be able to boil water, but you sure aren't making a soufflé.
Know Your IDE Inside Out
Your Integrated Development Environment (IDE) is your best buddy. Whether you're using Visual Studio Code, IntelliJ IDEA, or something else, knowing the shortcuts and extensions can save you loads of time. Picture jumping to a function definition without tediously scrolling through pages of code—it's possible if you leverage these built-in features. Plus, with extensions like Prettier or ESLint, you can automate style checks and corrections so you don't have to worry about messy code.
Real Magic: Command Line
The command line is more than just a black screen; it’s magic waiting to happen. Once you get the hang of it, you can navigate your projects way faster than point-and-clicking through folders. Commands like git
, grep
, and find
can be incredibly efficient when fetching data or managing files across projects. It may feel daunting at first but stick with it, and you'll soon wonder how you ever managed without it.
Automation: Why Do It If You Can Script It?
If you find yourself doing the same task over and over, it's time to script it. Automation tools like Jenkins for continuous integration and deployment, or Selenium for automated testing, can drastically cut down on grunt work. Not only does this boost your productivity, but it also reduces human error. Plus, scripting repetitive tasks means you have more time to focus on creative problem-solving and writing better code.
Version Control Like a Pro
We can't talk about tools without mentioning version control systems like Git. Understanding how to branch, merge, and roll back changes can save your skin. Branching allows you to experiment freely; merging ensures smooth collaboration. If you’re not using version control effectively, you’re not just leaving productivity on the table—you’re inviting chaos into your codebase.
One handy trick? Start using pull requests and code reviews through platforms like GitHub or Bitbucket. They aren’t just for show—they help catch issues before they turn into big problems, enhancing your coding workflow in more ways than one.
Code Optimization Techniques
So, you're ready to dive into the world of code optimization to speed up your programming? Great choice! Let's break down a few killer techniques that can turn your code from clunky to slick.
1. Identify and Remove Bottlenecks
Start by figuring out where your program is taking its sweet time. Use profilers to get insights into your application's performance. Tools like PyCharm or Visual Studio's profiling features are spot-on for this. Once you've identified the slow bits, you can dig in and fix them.
2. Efficient Data Structures
Pick the right data structures based on what you need. Using a hash table or dictionary where appropriate can greatly reduce time complexity. If searching is crucial, maybe give binary search trees a go!
3. Avoid Redundancy
Why repeat code when you can loop? Loops and functions aren't just neat—they make your coding life easier. Spot areas where you're doing the same thing over and over, and simplify.
4. Keep It Simple
Simplicity is your friend! Write code that's straightforward and easy to understand. Less complexity means fewer bugs and faster problem-solving.
5. Optimize Loops
Loops are often a major pain point. Check if you can reduce the number of iterations or move calculations outside of loops. Little tweaks can make a big difference.
6. Minimize I/O Operations
Input/output operations can slow things down big time. If you can, read and write in bulk rather than bit by bit.
Here's a sneak peek into a time it pays off:
Operation | Standard Time (ms) | Optimized Time (ms) |
---|---|---|
Read Small File | 120 | 30 |
Write Large File | 300 | 80 |
7. Use Built-in Libraries
Why reinvent the wheel? Languages like Python and Java have tons of built-in libraries that can fast-track your solutions. They’re pre-optimized and ready to go, which will help you program faster.
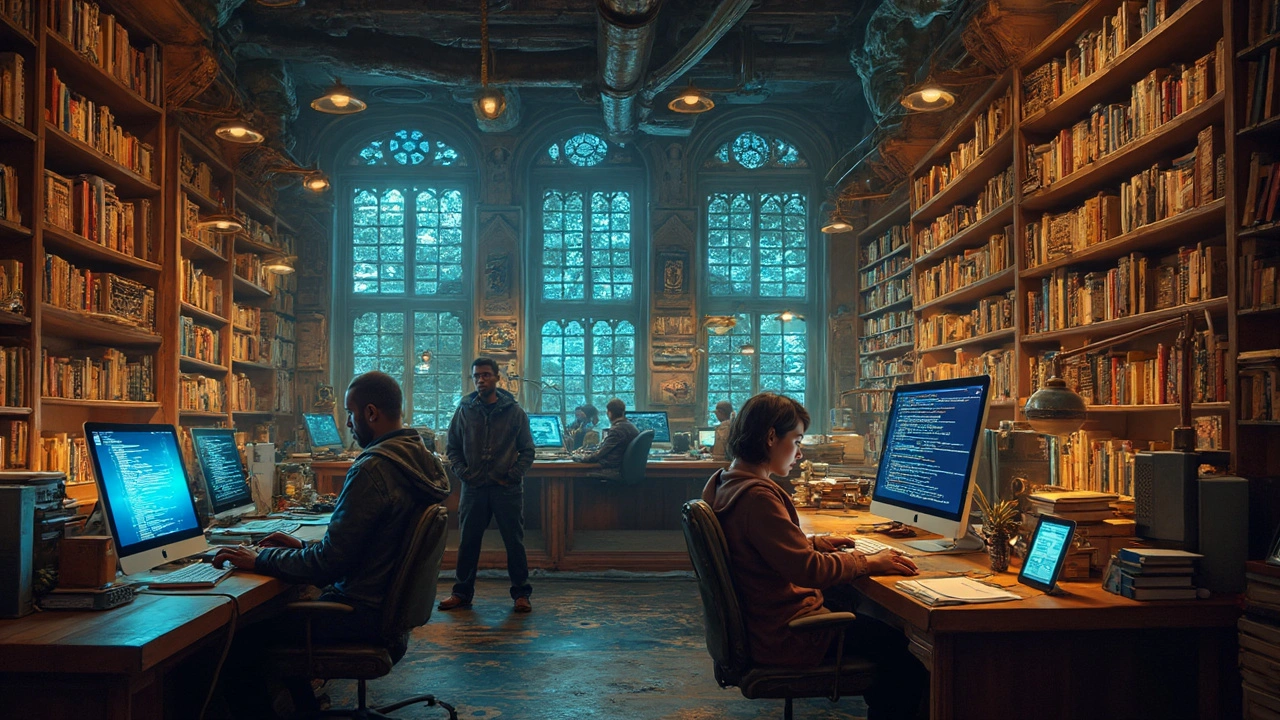
Streamlining Workflow
Who doesn't love saving time and effort? Streamlining your workflow can make all the difference when you're aiming to program faster. The secret sauce? Efficiency!
First up, know your priorities. It's easy to get sidetracked by shiny new projects or endless code tweaks. Use a to-do list system, like Kanban or Trello, to keep track of what's really important. This way, you focus on critical tasks without getting lost in the noise.
Automate Routine Tasks
If you're doing a task repeatedly, it's time to automate. Use scripts or third-party tools to handle these repetitive jobs. Whether it's setting up development environments or deploying code, automation scripts can cut down hours of mundane work to mere seconds.
- Automate build processes with tools like Jenkins or Travis CI.
- Use automation scripts for database backups.
- Set up automated tests using tools like Selenium or Jest.
Stay Organized with Version Control
Ever lost work because of a coding mishap? Enter version control systems like Git. They allow you to experiment without the fear of making irreversible changes. A clean commit history is not just good practice—it's life-saving when you need to roll back or understand past decisions.
Effective Time Management
Time-tracking tools like RescueTime or Toggl can help you understand where your time goes and how you can optimize it. Consider allocating specific time slots for different coding activities, like bug fixes or new feature development. A structured schedule often keeps your creative juices flowing without burning out.
In a world where deadlines loom large, getting your workflow on point is crucial for advancing in your coding career. The more you streamline, the more you'll find your productivity—and your confidence—skyrocketing.
Learning and Unlearning Habits
Breaking habits isn't easy, especially the ones lurking in your software development routine. But if you want to speed up your programming game, embracing new habits and ditching old ones is crucial. Let's dive into what you might need to revamp.
Embrace Continuous Learning
Ever heard the phrase, 'Learn something new every day'? It couldn't be more accurate for coders. Technologies and tools evolve faster than most of us can keep up with. Set aside time each week dedicated to learning something new in tech—it could be a tool, a language feature, or a fresh coding tips tutorial. Imagine coding as cooking; new ingredients and techniques will always make your dish better.
Let Go of the Fear of Failure
Okay, so you tried something new and broke your code—big deal. Failure is just another step toward programming faster. Don’t cling to the safe and familiar. Push boundaries, make mistakes, and then learn from them. It’s only through trial and error that you can fine-tune your skills.
Practice the Art of Saying No
Taking on too much can turn any enthusiast into a burnout case. Learn to say no to unnecessary meetings, irrelevant projects, or requests that don’t align with your goals. Shield your focus and energy like your code depends on it—because it does.
Regularly Review Your Workflow
Have a habit of sticking to a given process just because it’s what you’ve always done? It’s time for a change. Conduct a review of your workflow at least once a quarter. This could mean swapping cumbersome practices for more efficient ones, which directly ties into coding more efficiently.
Feeling stuck or hesitant is normal, but think of these adjustments as upgrading from a bike to a motorbike in your coding career. You'll get the hang of it, and once the habits are reset and refreshed, there’ll be no looking back. Just ask any seasoned coder—they’ll tell you the same.
Collaboration and Sharing Knowledge
We all know that two heads are better than one, right? In the world of programming, this couldn't be more true. Successful developers understand that collaboration isn't just optional—it's essential. When coding tips and tricks get shared among team members, magic happens.
Why Collaboration is Key
You're not an island. When you work with others, you get a chance to see problems from different angles. Maybe you're stuck on a bug, and another developer has already faced it in the past. Sharing knowledge can save hours, even days, reducing frustration and increasing productivity. Plus, understanding how someone else approaches a problem can give you new tools for your own software development toolkit.
Tools That Make It Happen
Thanks to technology, collaborating has never been easier. Platforms like GitHub aren't just about storing code—they're powerful collaboration tools where developers can review each other's work and share insights instantly. Pair programming takes it a step further, allowing two people to work on the same codebase simultaneously. It's a great way to learn, to teach, and to keep programming faster as a team.
Building a Culture of Sharing
Think of workplaces where sharing knowledge is the norm. Companies that encourage open communication and regular code review sessions often see higher quality outcomes. In these environments, developers don't just hoard solutions—they pass them on, teaching their peers and boosting the whole team's capabilities.
On platforms like Stack Overflow, sharing extends beyond company walls. Developers worldwide solve each other's problems, creating an environment of global collaboration that feeds back into individual and team growth.
And when you share your insights, you're not just helping others—you reinforce your own understanding, too. It's a win-win that turns good programmers into great ones.
Platform | Users | Primary Use |
---|---|---|
GitHub | Over 100 million | Code collaboration, version control |
Stack Overflow | Over 14 million | Knowledge exchange, problem-solving |