So you've got your code, your baby, and it just won't behave the way you want. You scream, the neighbors think you're learning to yodel, and the debugger's giving you cold sweats. Welcome to debugging, the unsung hero of coding—the thing that transforms your Frankenstein-like code into a masterpiece of functionality.
Debugging isn't just about squashing bugs; it's like being a detective on the case of the erroneous code. You snoop around your own code lines for logical slip-ups, syntax errors, or the ever-dreaded typos. At times, it feels like looking for a needle in a haystack. But when that code finally runs the way you want it to, oh man, that’s the holy grail.
But why's it so crucial? Imagine launching a spaceship with a crossed wire; the same can apply to code. One wrong line, and boom, things go south. Software only shines when bugs are fixed, which keeps users from shouting at their screens and instead, makes them a fan of your app or program.
- The Importance of Debugging
- Common Debugging Techniques
- Tools of the Trade
- Tips for Effective Debugging
The Importance of Debugging
Debugging is the backbone of software development. Think of it like tuning a guitar before a big performance. Without it, your code is likely to sound off-key, frustrating users and tanking your app's reputation. Debugging is what takes our messy, raw code and whips it into a working, polished product.
The first thing to understand is that bug fixes aren't optional; they’re a necessity for delivering reliable software. A study by the Consortium for IT Software Quality found that poor software quality resulted in economic losses of over $2 trillion in the United States alone. A staggering number, right? That's where good debugging practices make a huge difference.
When you follow a routine of consistent debugging, you're not only enhancing the user experience but also preventing security risks. A missed bug can be an invite for cyber threats, something that no developer wants on their conscience.
Now, debugging is not just for emergencies. Regular checks help catch bugs early, saving time and resources. Think of it as the world's best insurance policy for your code. By identifying and repairing bugs as they appear, you build a more robust application that's less likely to crash or produce incorrect results during critical moments.
Moreover, debugging helps improve your problem-solving skills. By diving deep into the code to find solutions, your understanding of programming concepts and logic becomes sharper. With each bug you fix, you're sowing seeds of wisdom, slowly but surely turning into a coding ninja.
Common Debugging Techniques
Alright, let's talk about how you can turn that tangled mess of code into a smooth-running beauty. Debugging doesn't have to be a nightmare if you know what to look for and how to tackle it.
First up, the age-old tradition of print debugging. It's like scribbling notes in the margin of a textbook. You drop in print statements to figure out what's happening with your variables as the code runs. Seeing those values can give you the 'Aha!' moment you need, helping you pinpoint where things go wrong.
Then there's the nifty use of a debugger tool. Almost every modern IDE comes with an integrated debugger. They let you set breakpoints, step through your code line-by-line, and inspect variables on the fly. It's like having X-ray vision for your code's inner workings.
- Breakpoints: They let you pause the code execution so you can take a closer look at what's happening at a specific point. Press pause concerning the bugs!
- Step Over: This allows you to go over a line of code without diving into the functions or methods within it. A neat way to bypass complexity temporarily.
- Step Into: Use this to get deep into a function call and see what’s cooking beneath the surface. Useful for functions that act up.
Another trusty technique is the use of version control systems as a safety net. Ever made changes only to realize you’ve thrown a wrench in the whole system? With a tool like Git, you can compare your buggy version with older, working versions to spot what went south.
And don't forget about the power of rubber duck debugging. Sounds quacky, but explaining your code to a rubber duck (or any inanimate object) forces you to slow down and think about what each part is supposed to do. This often reveals things your brain might skim over when silently coding away.
If something feels off, Google’s there to back you up like a trusty sidekick. Chances are, someone else has run into the same issue you're having. Stack Overflow and forums are gold mines of information, offering insights from those who've been in your shoes and solved similar problems.
Finally, keeping a log file can be a lifesaver. As your application writes logs, you can trace through actions and see where things might be off. It's like following breadcrumbs back to the bug's lair.
Debugging isn't a one-size-fits-all process, but by mixing and matching these techniques, you’ll find the bugs and hammer them out with less headache.
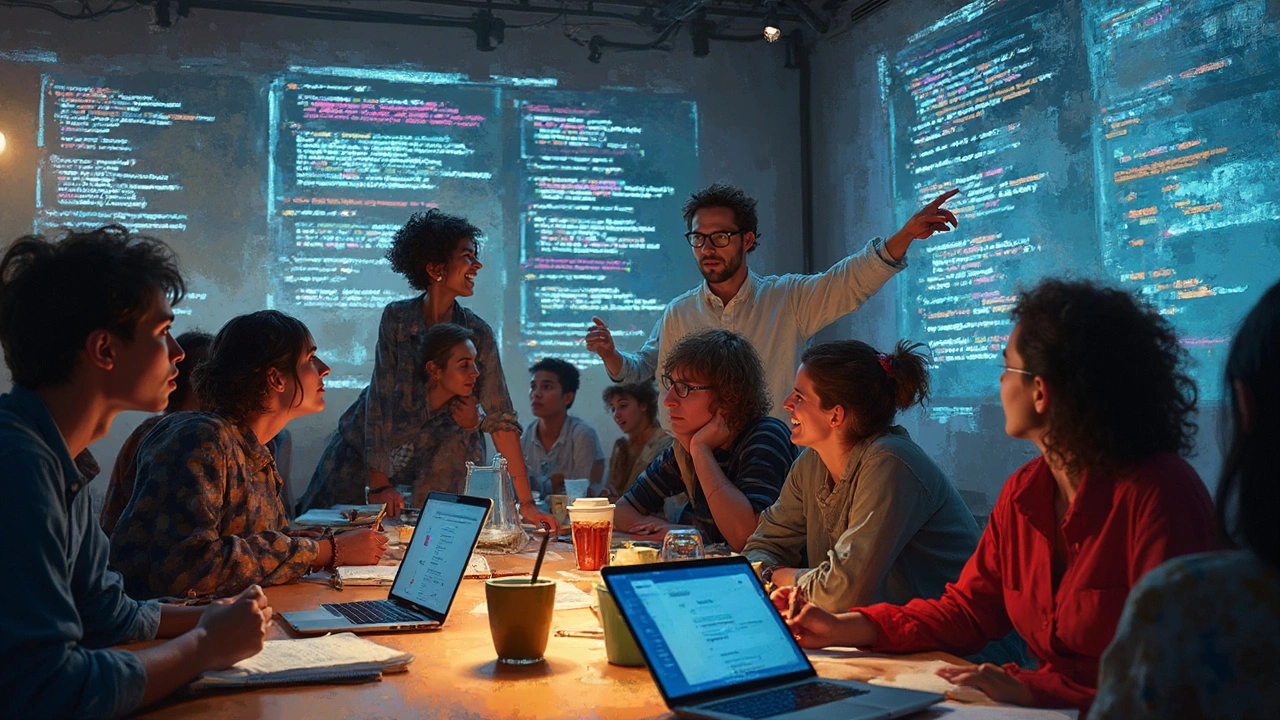
Tools of the Trade
When it comes to code debugging, having the right tools can make a world of difference. It's like having a trusty Swiss Army knife in your back pocket—handy for all kinds of situations. Let's dive into some essential tools that can turn every developer into a debugging wizard.
First up, we've got integrated development environments (IDEs) like Visual Studio Code, IntelliJ, or Eclipse. These trusty companions often come packed with built-in debugging features. You can set breakpoints, watch variables, and step through your code line by line, all in a sleek interface. They streamline the debugging process and are a staple in any coder's toolkit.
Following the heavyweights, there's the beloved debuggers like GDB (GNU Debugger) or LLDB. These are old-school but gold-school. They’re command-line tools that provide a deeper dive into the running code, especially useful when working with languages like C or C++.
Then, there are logging frameworks which might seem less glamorous but pack a punch when tracking down issues. Libraries like Log4j for Java or Winston for Node.js can help you trace what your code is up to by printing out logs at different levels—info, warning, error, you name it.
For web developers, browser developer tools are essential, too. Chrome DevTools and Firefox Developer Edition offer powerful built-in features for debugging JavaScript. They can catch those pesky frontend bugs and even optimize your CSS and HTML.
Static code analyzers are another valuable asset. Tools like SonarQube and ESLint can detect bugs and code smells even before you run the program, essentially halting some errors in their tracks. Best part? They help you keep your code clean and readable.
Last but not least, don't underestimate the power of the community-driven toolchains. Platforms like GitHub offer integrations such as CI/CD pipelines with error-logging capabilities, ensuring that each code commit is validated meticulously.
If you're serious about improving your debugging skills, getting familiar with these tools is a must. They are the knight's armor for every developer in the battlefield of software development.
Tips for Effective Debugging
Debugging isn’t some mystical art reserved for coding wizards. It's a skill you can get better at with practice and the right approach. Here’s the lowdown on making your debugging adventures a bit less wild and a lot more efficient.
First things first, embrace the power of breaking down your problem. If your program is acting up, isolate the troublesome section. By narrowing down, you’re slicing up the problem into bite-sized chunks instead of tackling a monstrous hydra of an error all at once.
Next, let your tools do the heavy lifting. Got a debugger? Use it! These tools can step through your code, showing you the soul of your script line by line, like having X-ray vision for your code. Seeing where things go sideways—made easy!
- Print Statements: Old but gold. Don’t underestimate the power of a well-placed print statement to see what’s going on in there.
- Rubber Duck Debugging: Yes, it sounds silly, but explaining your problem out loud (even to a toy duck) can help you think aloud and untangle your thoughts.
- Stay Calm and Debug On: Panicking won’t find that missing semicolon for you. Take a break, clear your head, and look at the issue with fresh eyes.
- Version Control is Your Friend: Mistakes happen, and when they do, having a snapshot of your working code in your version control can save you from hours of hair-pulling.
- Read the Error Messages: Before you dive into fixing, actually read those error messages. They often tell you exactly what the problem is—or at least, point you in the right direction.
Here's a quick peek at some common tools that can supercharge your debugging:
Tool | Purpose |
---|---|
GDB | Great for C/C++ debugging |
Chrome DevTools | Essential for web development |
PDB | Python’s handy debugger |
Visual Studio Debugger | Comes packed with features for .NET developers |
Every programmer hits walls; it's how you climb over them that sets the pros apart from the newbies. With these tips, your debugging skills can become the trusty sidekick that helps you nail problems without losing your sanity.