Ever wondered why some software runs smoothly while others are annoyingly slow or buggy? The secret often lies in the art of code debugging. It's a critical practice that aims to identify and fix errors in the code, ensuring everything works as it should.
Let's take a closer look at why debugging is vital to software performance, explore some common techniques used by developers, introduce a few indispensable tools, share practical tips, and discuss how maintaining clean code can make a world of difference. By the end of this, you'll see how powerful effective debugging can be for your software projects.
- The Importance of Code Debugging
- Common Debugging Techniques
- Essential Debugging Tools
- Practical Tips for Debugging
- Benefits of Clean Code through Debugging
The Importance of Code Debugging
Code debugging is the unsung hero behind high-performing software. At its core, it's about finding and fixing errors in the code. These errors, known as bugs, can range from simple syntax mistakes to complex logical errors. By squashing these bugs, developers ensure that the software runs smoothly, providing a better experience for the users quite literally.
Debugging isn't just a one-time task during the development phase; it’s a continuous process. As new updates and features are added, the chances of new bugs slipping into the code increases. Regular debugging keeps the software healthy and reliable, reducing the frequency of crashes and performance lags. One interesting fact is that debugging can often consume up to 50% of the development time. This might seem like a lot, but it's a clear indication of how crucial it is for maintaining software quality.
Effective debugging also plays a crucial role in improving software performance. Unoptimized code with hidden bugs can slow down the execution speed. By identifying and resolving these issues, developers can significantly boost the efficiency of the software. Think of it like tuning an engine; a well-tuned engine runs faster and smoother than one with hidden problems.
Beyond performance, debugging helps with security. Many security vulnerabilities arise from errors in the code. By thoroughly debugging, developers can identify potential security holes and patch them before they are exploited. This is especially critical in today’s world where cyberattacks are more common than ever.
Moreover, debugging fosters better coding practices. As developers debug their code, they learn from their mistakes and gradually write cleaner, more efficient code. This not only improves the current project but also has long-term benefits as the same practices are applied to future projects. Encouraging a culture of thorough debugging can lead to a team of more competent and meticulous developers.
As Edsger Dijkstra, a renowned computer scientist, once said, "If debugging is the process of removing software bugs, then programming must be the process of putting them in." Understanding this humorous yet true insight emphasizes the importance of debugging in the software development cycle.
One dramatic case highlighting the importance of debugging was the Ariane 5 rocket failure in 1996. Just 37 seconds after liftoff, the rocket self-destructed due to a malfunction in its software—a bug caused by an arithmetic overflow. This incident cost $370 million, underscoring that even minor bugs can have huge repercussions.
Given the significant impact on performance, security, and overall software quality, it’s clear that debugging is not just an optional step but a vital one in software development. By prioritizing and integrating thorough debugging practices, developers can ensure their software is not only functional but also efficient and secure.
Common Debugging Techniques
Debugging is an essential stage in the software development lifecycle. It pinpoints errors, known as bugs, in the code, which can disrupt the functionality or slow down the application. Certain methods are universally practiced by software developers to effectively troubleshoot and fix these issues.
One popular technique is the use of print statements. By inserting print or log statements into the code, developers can see the values of variables at specific points during the program’s execution. This can help trace where something goes awry. Though it may seem rudimentary, it remains a staple in debugging.
Another well-known approach is utilizing a debugger tool. These tools allow you to run your code step-by-step, inspect variable states, and understand the control flow. Popular debug tools like GDB for C/C++ or the built-in debuggers in IDEs such as Visual Studio for .NET and PyCharm for Python are incredibly effective. Debugging tools can break the program execution at predetermined points (breakpoints), enabling detailed examination of the program's state.
"Given sufficient eyeballs, all bugs are shallow," noted Eric S. Raymond, emphasizing the importance of systematic code inspection.
Sometimes, bugs are not in the code itself but in the structure or logic. The method of code review can be extremely valuable here. Code review is a process where peers scrutinize each other’s code. A fresh set of eyes can often catch bugs that the original developer missed. It is also a great way to share knowledge and standardize coding practices.
Unit testing is another indispensable technique in a developer’s toolbox. Unit tests verify that individual units of source code, like functions or methods, work as expected. By using frameworks like JUnit for Java or pytest for Python, developers can run tests to ensure their code changes haven't introduced new bugs. These tests are automated, which means they can be repeatedly run with minimal effort.
When dealing with bugs that are difficult to reproduce, “rubber duck debugging” can be surprisingly effective. This method involves explaining your code, line-by-line, to an inanimate object like a rubber duck or even a colleague. This process often leads to self-discovery of the issue as it forces you to reconsider each step logically.
Profiling is a more advanced technique used to improve performance rather than fixing outright errors. Profilers can measure where your program spends the most time or consumes the most memory. Tools like Valgrind for C/C++ or py-spy for Python allow developers to identify and optimize bottlenecks. This is crucial for performance-intensive applications.
Using version control systems (VCS) like Git can also assist in debugging. By tracking changes over time, developers can pinpoint exactly when a bug was introduced. Branching and reverting features in VCS allow them to isolate problematic code without disrupting the main development workflow.
Each of these techniques plays a significant role in making code more reliable and efficient. By integrating multiple methods, developers can tackle almost any issue that arises, ensuring their software runs smoothly and efficiently. Consistent practice and refinement of these techniques lead to exceptional development skills and higher-quality software.
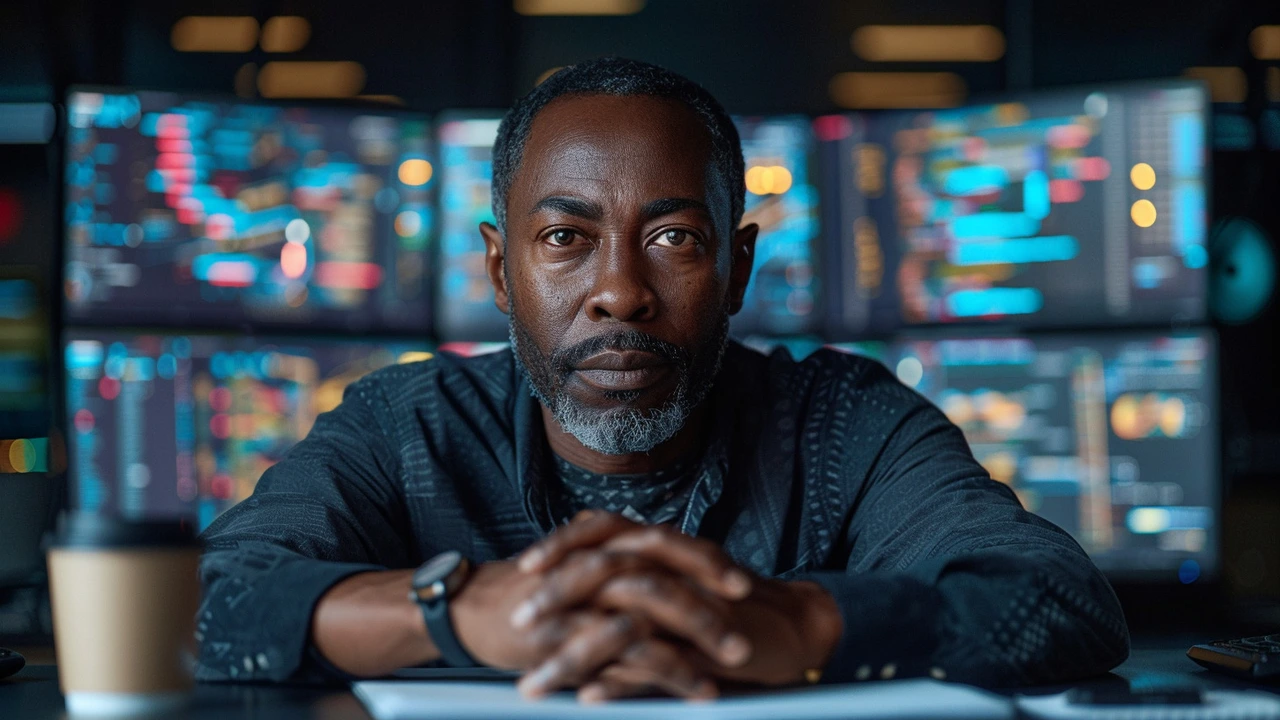
Essential Debugging Tools
Every developer knows the frustration of encountering bugs. To ease this process, a range of debugging tools is available, each designed to pinpoint and fix errors efficiently. These tools become indispensable as they help maintain software performance while ensuring user satisfaction.
Integrated Development Environments (IDEs) play a pivotal role in code debugging. These comprehensive tools offer various features like auto-completion, error detection, and debuggers. Popular examples include Visual Studio, Eclipse, and IntelliJ IDEA. They provide real-time feedback, which can significantly reduce the time spent on locating and fixing bugs.
Another crucial tool is the command line debugger. GDB (GNU Debugger) is a well-known example that supports various programming languages such as C, C++, and Fortran. GDB allows developers to see what is happening inside a program while it runs, or what the program was doing at the moment it crashed. This visibility helps in identifying the exact location of the error and understanding the flow of the program.
Static analysis tools like SonarQube and CodeClimate are also essential. These tools analyze the code without actually executing it, identifying potential bugs, code smells, and security vulnerabilities. They provide a comprehensive report that helps developers improve code quality and avoid potential issues before they become critical.
Among the most modern solutions are real-time error tracking tools like Sentry and Rollbar. These tools work by tracking and reporting errors directly from live applications. They provide detailed insights and stack traces which help in quickly identifying the root cause of errors, leading to faster resolutions and minimized downtime.
To further enhance debugging, profiling tools like Apache JMeter and YourKit can be immensely useful. These tools help identify performance bottlenecks by monitoring the system’s behavior under different loads. They provide a detailed analysis of memory usage, CPU cycles, and other vital metrics, enabling developers to optimize their code for better performance.
As Martin Fowler, a renowned software developer, once said, "Any fool can write code that a computer can understand. Good programmers write code that humans can understand." This emphasizes the importance of tools that not only help in fixing errors but also in writing clean and maintainable code.
Browser developer tools are indispensable for debugging web applications. Chrome DevTools and Firefox Developer Tools provide a suite of utilities to inspect elements, debug JavaScript, and analyze the performance of web applications. These tools are built directly into the browsers, making them easily accessible and highly efficient.
Lastly, version control systems like Git are crucial in the debugging process. They allow developers to track changes made to the code, revert to previous states, and collaborate with team members. Tools like GitHub and GitLab offer additional features such as pull request reviews and issue tracking, which further streamline the debugging process.
Practical Tips for Debugging
Debugging might seem like a daunting task, but with the right approach, it can become a natural part of your development process. Here are some practical tips to help you tackle those elusive bugs more efficiently.
Break Down the Problem
When facing a bug, it's crucial to break down the problem into smaller, manageable parts. Start with identifying the symptoms and then work backward to locate the source. This methodical approach makes it easier to pinpoint the exact cause without feeling overwhelmed. A common strategy is to divide your code into segments and test each one individually.
Reproduce the Issue
If you can't reproduce a bug, you can't fix it. Consistently reproducing the issue is half the battle won. Make sure you document the steps required to trigger the bug. This will not only help you understand what went wrong but also enable anyone else working on the project to follow the same steps.
Use Debugging Tools
Don't underestimate the power of debugging tools such as debuggers, log analyzers, and profilers. Modern Integrated Development Environments (IDEs) come with built-in tools that can step through your code, watch variables, and visualize call stacks. Familiarize yourself with these tools to save time and effort. For instance, using breakpoints effectively allows you to pause code execution and inspect the state of your application at critical moments.
Read the Error Messages
It sounds simple, but always read the error messages carefully. Error messages and stack traces give valuable clues about what went wrong. Often, developers make the mistake of ignoring these messages, which can lead to unnecessary hours of guessing and trial and error. Pay close attention to the details provided; sometimes a slight variation in an error message can lead you to the solution.
Consult Documentation and Community
When you're stuck, don't hesitate to consult the documentation. It's there for a reason. Also, leverage the power of community forums like Stack Overflow, GitHub Issues, and even Reddit. These platforms are filled with experienced developers who might have encountered and resolved similar issues. Posting precise questions will often yield useful insights and save you a considerable amount of time.
“Programs must be written for people to read, and only incidentally for machines to execute.” – Harold Abelson
Keep a Bug Log
Maintaining a detailed bug log can be incredibly helpful, especially for larger projects. Documenting the bugs you encounter, how you fixed them, and any relevant notes can provide a valuable reference for the future. This practice also helps in keeping track of recurring issues and spotting patterns that might need a more significant refactor.
Stay Patient and Persistent
Lastly, debugging requires a good deal of patience and persistence. It's easy to get frustrated when a bug seems unsolvable. Take breaks when you need to, and approach the problem with a fresh perspective. Sometimes stepping away for a short while can help you see the problem in a new light.
By incorporating these practical tips into your routine, you'll find debugging to be a more manageable and even rewarding part of software development. With practice, you'll not only become more proficient at fixing bugs but also at writing cleaner and more efficient code, which will ultimately enhance the performance of your software.
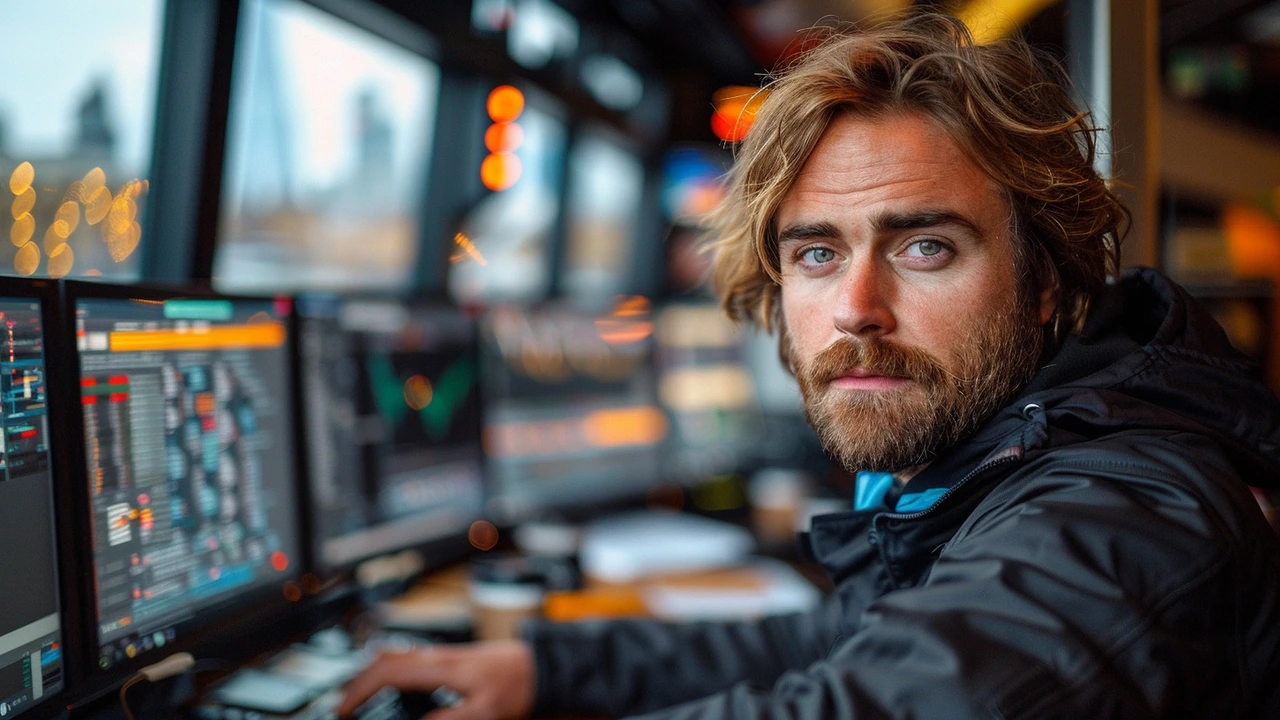
Benefits of Clean Code through Debugging
When you dive into the world of software development, you quickly realize the importance of clean code. It’s not just about making your programs run, but making sure they run well. Debugging plays an essential role in maintaining clean code, which in turn leads to numerous benefits. One of the primary advantages of keeping your code clean through regular debugging is improved software performance. When code is free of errors, it runs more efficiently and is less likely to encounter unexpected issues that can slow down or crash your application. Clean code is easier to understand, making it simpler for developers to identify and rectify potential performance bottlenecks.
Additionally, clean code is much easier to maintain. When you or another developer need to make changes or add new features, having well-organized and error-free code dramatically reduces the time and effort needed for these tasks. Debugging helps by systematically identifying and eliminating issues, preventing them from snowballing into larger problems. This leads to a more stable codebase that can be extended or modified with minimal disruption to existing functionality.
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” - Martin Fowler
A significant benefit of keeping your code clean through debugging is enhanced collaboration. In a team setting, having well-written, error-free code makes it easier for multiple developers to work on the same project. This is crucial for maintaining productivity and ensuring that everyone is on the same page. Poorly written code full of bugs can lead to miscommunication and a lot of wasted time trying to figure out what others have done. By prioritizing debugging and maintaining clean code, teams can work more cohesively and efficiently.
Another overlooked advantage of clean code is in the realm of security. Bugs and errors can sometimes lead to vulnerabilities that malicious users can exploit. Regular debugging helps identify these weak points and address them before they can be taken advantage of. Clean code is more robust and less likely to contain security flaws that can compromise the integrity of your application and the data it handles. The peace of mind from knowing your software is secure is invaluable.
Let’s not forget about scalability. As your project grows, having clean and well-debugged code makes it easier to scale your application. You can add new features, improve existing ones, and handle more extensive data without worrying about the underlying code collapsing under the weight. Clean code acts as a firm foundation upon which you can build more complex and powerful applications. It’s much easier to optimize and improve performance when the baseline code is in good shape. Additionally, clean code often results in fewer bugs reported by users, leading to higher satisfaction and better reviews for your software.
To summarize, the benefits of clean code through debugging are multi-faceted. From enhancing software performance and maintainability to improving collaboration, security, and scalability, regular debugging is indispensable. It's more than just a routine check; it’s an investment in the longevity and success of your software. Developers who prioritize debugging and maintaining clean code often find themselves with more reliable and efficient applications, smoother workflows, and happier users.