Debugging can be a challenging task, but it's a crucial part of coding. Errors are bound to arise, and knowing how to tackle them effectively can make a world of difference. Whether you're a newbie dipping your toes into programming or someone who's been in the game for a while, mastering debugging skills is essential.
Let's dive into five practical tips to help you debug your code more efficiently. These strategies will not only save you time but also reduce the headaches that come along with finding and fixing errors.
- Understand the Problem
- Use a Debugger Tool
- Print Statements Wisely
- Keep Your Code Clean
- Learn from Insights
Understand the Problem
Grappling with a thorny bug often starts with pinpointing what's actually going wrong. Before diving into code alterations, take a moment to clearly understand the issue. This means reproducing the problem consistently. If you can reproduce it, you're halfway to solving it. Sometimes, the error messages and logs can provide clues. Read them carefully. They often direct you toward the root of the problem.
Accurate reproduction of a bug may seem basic, but countless hours can be wasted on elusive or intermittent issues. Document the exact steps leading to the bug and try breaking the process into smaller parts. By isolating each part, you can identify whether the problem is caused by a particular segment. Don’t guess; rely on data and observations to guide your debugging efforts.
Moreover, try to understand the intended behavior of your code. What should be happening versus what is happening? This context is essential. Sometimes, you might find that the problem isn’t the code itself but an unmet condition or a missing dependency. Debugging isn’t just a technical challenge; it’s a logical puzzle.
Consider sharing your problem with a colleague or a community. A fresh set of eyes can offer a different perspective. Explaining the problem to someone else can also clarify your own understanding. As Albert Einstein once said, "If you can't explain it simply, you don't understand it well enough."
Finally, patience is key. Debugging is often more time-consuming than writing code. Don’t rush; take your time to methodically understand the problem. With a clear grasp of the issue, you can apply your skills and tools more effectively.
Use a Debugger Tool
Diving into the world of debugging can be intimidating, but using a proper debugger tool can ease much of this stress. Debugger tools are designed to help you step through your code, observe variables, and understand the flow of your program in a clear, organized manner. To put it simply, they act like a magnifying glass, helping you see every intricate detail of your code's execution.
One of the primary benefits of a debugger tool is the ability to set breakpoints. Breakpoints allow you to pause the execution of your code at specific lines. When your code hits a breakpoint, it stops, giving you the chance to inspect the current state of the application. This can be extremely helpful for identifying where something has gone wrong. Debuggers also let you step through the code line-by-line, watch variable changes, and evaluate expressions.
Consider using popular debugger tools such as the ones integrated into IDEs like Visual Studio Code, PyCharm, or Eclipse. These tools come equipped with powerful debugging features. For instance, in Visual Studio Code, developers can hover over variables to see their current values or use the console to execute commands and inspect states. This can be especially handy for complex applications where understanding the context is necessary.
“Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.” – Brian Kernighan
Another interesting feature offered by debugger tools is the ability to view the call stack. This shows you the sequence of function calls that led to the current point in execution. Understanding the call stack can be critical when you’re dealing with deep or nested function calls. Additionally, some debugger tools provide memory analysis features where you can track memory usage and detect leaks.
To make the most out of a debugger tool, it’s essential to familiarize yourself with its interface and functionalities. Spend some time exploring the different features, setting various types of breakpoints, and navigating through the controls. The investment in learning how to use your debugger tool efficiently will pay off when you’re faced with complex debugging tasks.
Remember, using a debugger is not just about finding errors; it’s also an excellent way to learn more about how your code operates. It allows you to trace execution paths and visualize data flows, which can be invaluable for refining your problem-solving skills and improving the overall quality of your code.
Key Features of Debugger Tools
- Breakpoints: Pause code execution at specific points.
- Step-through: Move through code one line at a time.
- Variable Inspection: Examine changes in variable values.
- Call Stack View: See the sequence of function calls.
- Memory Analysis: Monitor memory usage and detect leaks.
Embrace debugger tools as your ally in the battle against bugs. By leveraging their features and understanding their capabilities, you’ll become more adept at navigating through errors and sharpening your programming skills.
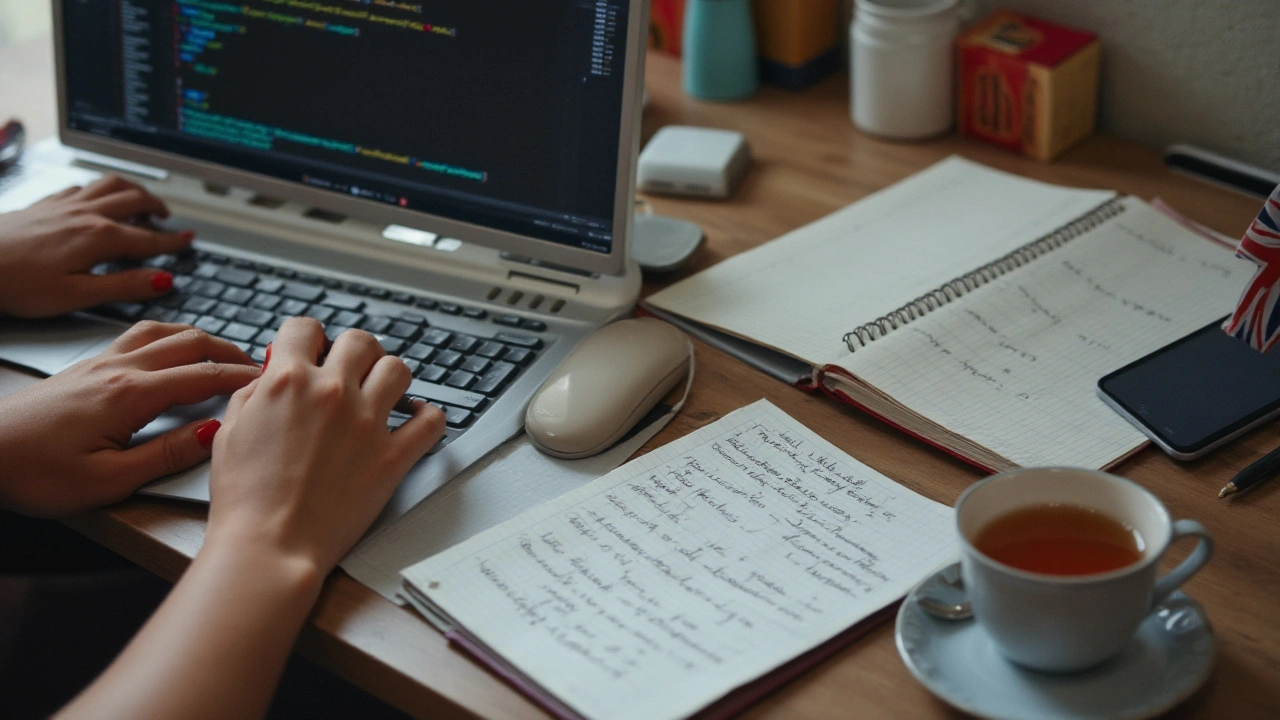
Print Statements Wisely
Using print statements is one of the simplest and oldest methods to debug code, yet it's still highly effective when done thoughtfully. Placing random print statements can clog your output and cause more confusion. Instead, you should be strategic about where and what you print. A well-placed print statement can help narrow down the problem by displaying what the code is doing at a specific point in time.
Firstly, ensure each print statement has a clear and descriptive message. Rather than just printing variable values, add context to what you are printing. For example, instead of print(x), use print('Value of x at this point:', x). This way, when you look at the output, you know exactly what variable is being displayed and at what point in the code.
Another good practice is to print statements in critical sections of your code, especially where logic branches occur. If you are dealing with loops, recursive functions, or conditional statements, print relevant information before and after these sections. These prints can give you insights into how the logic flows and where it might be going wrong.
Using temporary print statements is great, but remember to remove or comment them out once the debugging is complete, especially in production code. Leaving these statements can clutter the code and the console output, making it harder to understand and maintain. Some development environments even allow you to set conditional breakpoints, which can serve as sophisticated print statements without modifying your code.
Interestingly, a study by an IEEE research group found that most bugs are localized to a few lines of code. Using print statements wisely to focus on these areas can lead to quicker resolutions. They suggest starting by printing before and after suspect lines of code and then refining your focus based on the output.
"Make experiments and throw away the bad parts...Take good care of the good parts and use them again." - Linus Torvalds, Creator of Linux
Keep Your Code Clean
One of the simplest yet most effective tips for efficient debugging is maintaining clean code. Main reason is that code clarity allows you to easily spot mistakes and inconsistencies. Clean code follows a well-defined structure and is written in a way that anyone, including your future self, can understand.
Consider using meaningful variable names, which is a fundamental practice. For instance, if you're working on a function that calculates monthly expenses, name your variables to reflect their purpose, such as 'totalMonthlyExpenses' instead of just 't' or 'temp.' Meaningful names help you and anyone else reading the code understand its function without a single comment.
Another essential practice is to maintain proper indentation. Properly indented code ensures that the structure and flow are easily identifiable. Many modern integrated development environments (IDEs) have automatic indentation features, so there's no excuse for messy code structure. A well-indented block of code can help distinguish nested loops and conditionals clearly.
Removing commented-out code should also be a priority. Leftover snippets of code can clutter your script, making it difficult to read and maintain. It's better to completely delete them. If you think you might need it later, use a version control system like Git to track your changes instead of leaving commented-out lines scattered through your file.
Robert C. Martin once said, "Clean code is simple and direct. Clean code reads like well-written prose. Clean code never obscures the designer’s intent but rather is full of crisp abstractions and straightforward lines of control."
Organizing your code logically is another crucial aspect. Group related functions together and ensure that your file and folder structure makes sense. For example, if you have utility functions that you frequently use, put them in a separate file. This practice not only cleans up your main script but also makes your functions reusable.
Comments can also play a significant role in code clarity. But keep them short and to the point. Over-commenting can be just as bad as under-commenting. Aim to write comments that explain the 'why' behind particularly complicated or non-intuitive code sections, not the 'what' which should be evident from your method names and structure.
Finally, always be on the lookout for code smells. These are indicators that there's a deeper problem with your code. Examples include duplicated code, large classes, and long methods. Recognizing and refactoring code smells will help you keep your codebase clean, making it easier to debug in the future.
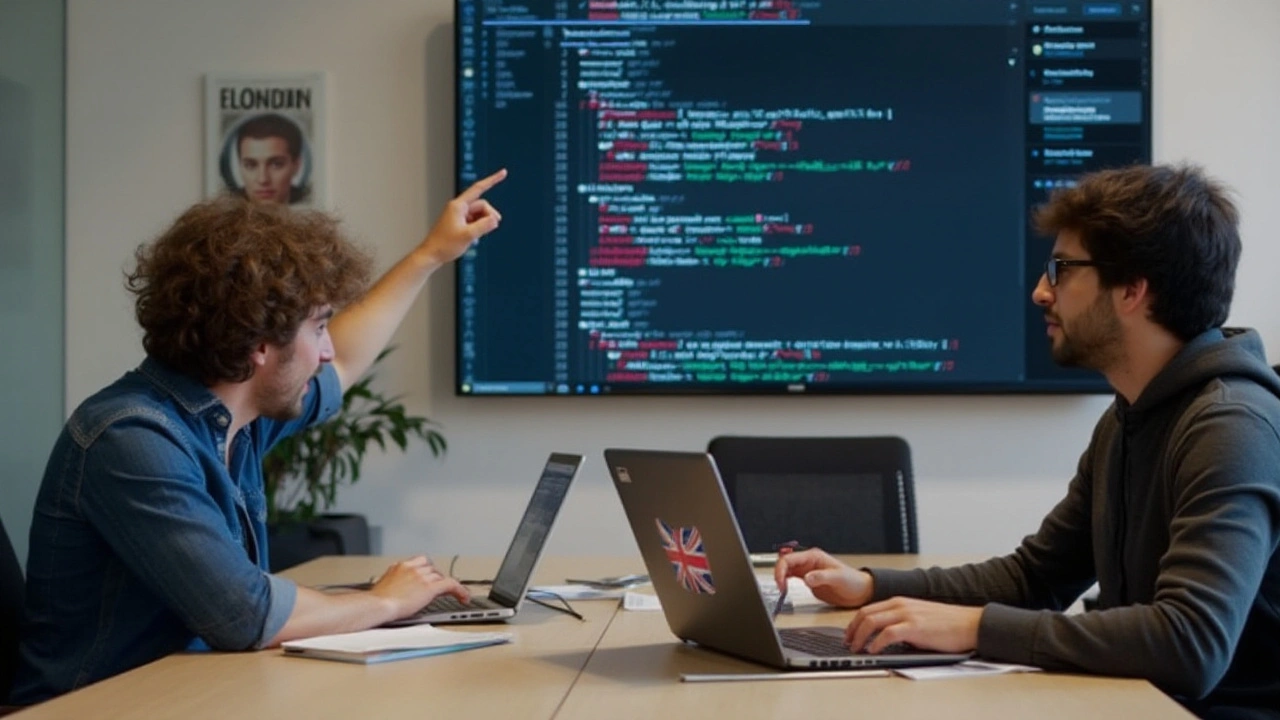
Learn from Insights
Every debugging session is not just an opportunity to fix errors but also a chance to understand your code better, improve it, and learn new strategies. Keeping a record of the issues you encounter and the solutions you discover can provide a valuable reference for future projects.
Reflecting on past mistakes can enhance your problem-solving skills and prevent similar errors in the future. Consider maintaining a debugging journal where you jot down the nature of the bugs, the steps you took to resolve them, and any patterns you notice. This practice helps in recognizing recurrent issues and refining your approach to debugging.
Learning from mistakes is essential for growth. As Mahatma Gandhi said, "Freedom is not worth having if it does not include the freedom to make mistakes."
Moreover, getting into the habit of conducting post-debugging reviews can highlight the areas of your code that need better design or documentation. Such reviews can involve discussing complex bugs with a senior developer or peer, as these discussions often present new perspectives and solutions.
Another effective method is participating in coding communities. Platforms like Stack Overflow, GitHub, and various developer forums are treasure troves of problems and solutions contributed by developers worldwide. Engaging with these communities allows you to learn from the experiences of others, which can be highly insightful.
Using debugging tools proficiently also equips you with insights into your code. For instance, modern IDEs like Visual Studio Code and PyCharm offer sophisticated debugging features such as variable watches, breakpoints, and stack traces, which can be game-changers when tracing the root cause of a bug.
Efficient debugging practices also include understanding the history of code changes. Using version control systems like Git allows you to track changes, identify when bugs were introduced, and roll back problematic updates. As Linus Torvalds, the creator of Git, stated, "Bad programmers worry about the code. Good programmers worry about data structures and their relationships." This emphasizes the importance of thinking about how changes in your code affect its functionality and debugging approach.
Finally, continuously educating yourself about new debugging techniques and tools fortifies your skills. Attend workshops, watch tutorials, and read articles to stay updated on the latest in code debugging. Knowledge about different strategies and tools makes you adaptable and efficient in handling various debugging scenarios.