Python has captured the hearts of many in the coding world with its user-friendly syntax and powerful capabilities. But while many of us dabble with its basic structures, truly mastering Python requires diving deeper into its multitude of features.
In this journey of exploration, we will venture into some intriguing Python tricks that promise to redefine how you approach coding in this versatile language. From list comprehensions that can make your code cleaner, to built-in functions that can perform complex tasks in a snap, you're about to discover tools that seasoned developers use with finesse.
Whether you’re a beginner eager to expand your skillset or an experienced coder looking to polish your craft, these tricks will provide you with valuable insights to unlock Python's full potential. Prepare to transform your coding practices, one trick at a time.
- Mastering List Comprehensions
- Utilizing Python's Built-in Functions
- Optimizing Code with Generators
- Enhancing Debugging Skills
Mastering List Comprehensions
List comprehensions in Python are a brilliant way to create lists succinctly and elegantly. They bring simplicity and efficiency to your code by compressing several lines into one concise expression. Imagine having the ability to generate new lists by applying an expression to each item in an existing collection, all with the ease of a single line. That's the magic of list comprehensions. For instance, crafting a list of squares for numbers 1 through 10 can be boiled down to a compact form with this technique, all while improving code readability. Getting comfortable with list comprehensions will not only polish your coding style but also mark a significant step in your journey to mastering Python tricks. They exemplify how Python’s syntax can be exploited for premium efficiency and clarity.
The use of list comprehensions is not merely about shortening code, though. It’s about thinking in Python, embodying its philosophy of readability and elegance. Let’s say you need to filter through a list to select specific elements. Without list comprehensions, this could require a loop scattered across several lines. With them, however, you can elegantly embed conditionals right within the expression. This delightful feature turns what could have been a tedious task into an engaging one, especially when aesthetics and function go hand-in-hand. You will find list comprehensions invaluable in data manipulation tasks, which are particularly prevalent in data analysis and machine learning workflows.
There are numerous scenarios where list comprehensions shine. They are perfect for implementing mapping operations or filtering criteria. They also handle nested data structures or tackle tasks in domain-specific applications. Suppose you're working in finance and need to convert currency values stored in a list based on exchange rates. List comprehensions can handle this with ease by mapping a conversion function over your data. Statistically, they are around 30% faster than traditional loops, due to their optimized syntax. Interestingly, Guido van Rossum, Python's creator, has even remarked on their capability to transform coding tasks, saying,
“The power of list comprehensions can make Python a magical wand for data transformation and manipulation.”
Imagine a scenario where you have a matrix and you're seeking to flatten it. List comprehensions allow you to elegantly do this in a way that's easy to follow yet efficient. You'll often encounter these in niches like computer graphics or network data analysis where you’re frequently wrangling multi-dimensional datasets and need both speed and readability. Now, consider creating a new list of squared values from an existing list of numbers. You can achieve this with a simple yet powerful statement like [x**2 for x in numbers]
. This not only speaks volumes in code simplicity but also articulates clarity for anyone following your code.
Getting the hang of list comprehensions opens a door to understanding Python at a deeper level. For instance, list comprehensions are just the start; as your comfort grows, you might find yourself naturally extending these principles to other forms of comprehensions in Python, such as dictionary and set comprehensions. These patterns are foundational for writing Pythonic code, and before you know it, you'll be integrating list comprehensions effortlessly across your entire codebase, making you not just proficient, but a genuinely adept Python programmer. This shift will prepare you for even more complex challenges in your coding journey, where efficiency and performance are key.
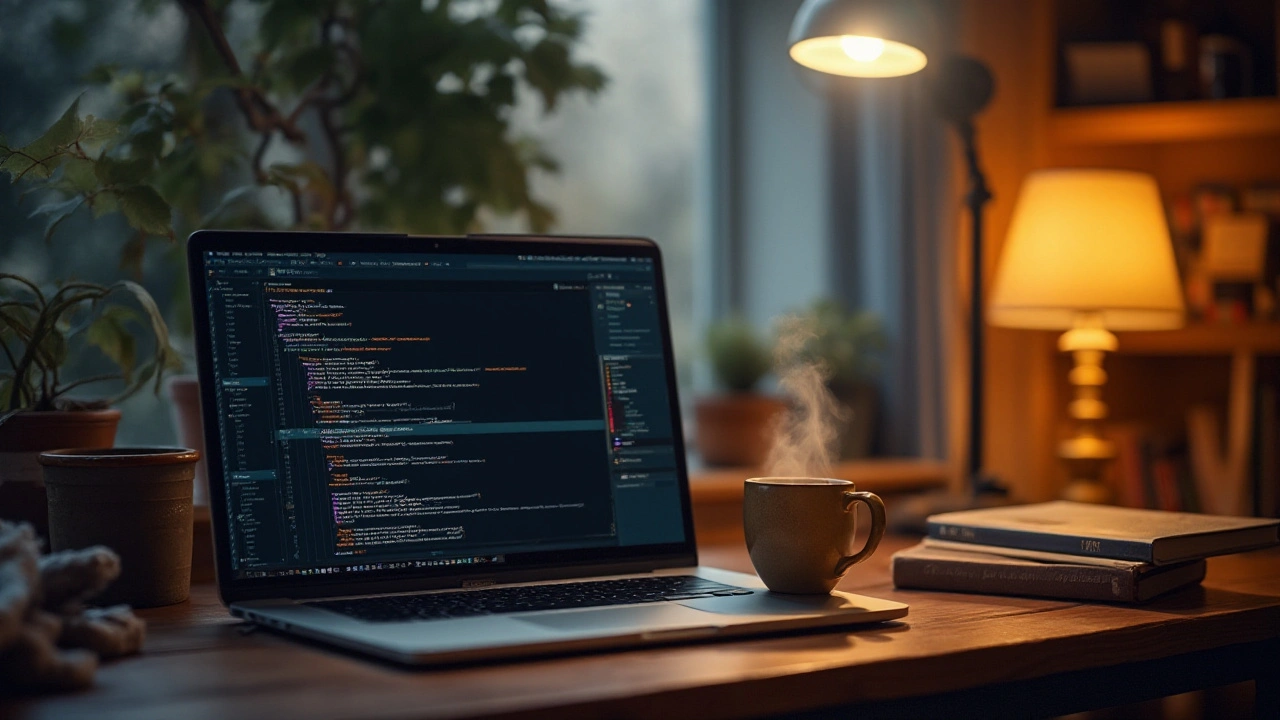
Utilizing Python's Built-in Functions
Delving into Python's rich ecosystem of built-in functions is akin to opening a treasure chest filled with tools ready to make your coding life simpler and more efficient. These functions, ready at your disposal, allow you to carry out common tasks without the hassle of writing extensive code. When you're looking to carry out operations like mathematical computations, data manipulation, or even type conversions, there's likely a built-in function available to make the job easier. Python tricks often hinge on understanding how to effectively leverage these ready-made solutions. Using these functions wisely can drastically cut down coding time while also minimizing error possibilities.
Let's take one of the most fundamental functions - len()
. This function is incredibly powerful in its simplicity. With it, you can find the length of elements in a string, list, tuple, or even dictionaries with ease. For instance, if you're dealing with multiple data structures and need to ascertain how many entries each contains, len()
is your best friend. Its usability is highlighted in data-driven projects where precise measurements are crucial. It's used in data validation, checks, and even for dynamic management of code that responds to dataset size. Talking about precision, the function round()
reigns supreme when it comes to numerical tweaking. Remember, the key with these functions is not just knowing they exist, but knowing when and how to use them effectively to unleash their full potential.
The beauty of Python is in its ability to let developers focus on solving complex problems by abstracting away mundane details. When you need to apply a function iteratively in a neat and clean way, functions like map()
and filter()
do wonders. These are applicable in scenarios where conditions or transformations need to be repeatedly applied across collections. Coding smartly begins with recognizing these repetitive patterns and applying the right function to streamline your logic. As a step further into this smarter coding universe, Python presents you with list comprehensions. It's not exactly a built-in function, but a nifty feature that simplifies making lists. With it, for-loops and conditionals in list generation become obsolete, reducing verbosity while maintaining readability.
Consider a scenario where you're dealing with a dataset that requires every string element to be turned uppercase, and only those elements which start with 'a'. With a few simple strokes, Python's built-in upper()
and a blend of filter()
can have your solution nailed precisely and succinctly. This is the hallmark of skilled Python development - conjuring elegant solutions from seemingly complex tasks with minimal effort. The result is code that is not only functional but efficient, following Python's philosophy of running with elegance and simplicity.
A noteworthy mention within Python's built-in selection is the zip()
function, which proves beneficial in scenarios where parallel iteration is necessary. This function combines elements from multiple iterables, producing a compact set of tuples. This is especially useful in data analysis and manipulation, where simultaneous iteration over dataset columns is routine. For example, consider you have two lists, one of names and the other of corresponding scores, in need of being combined into a dictionary. zip()
offers an efficient path forward, automating what would otherwise be a manual and tedious process.
Delving Deeper
To fully appreciate these built-in helpers, one must step back and recognize Python's philosophy. Python encourages writing code that is not only efficient but also readable and accessible to those who will maintain it after you. In many cases, what gives rise to a powerful block of Python code is the judicious application of these programming tips and functions. Used artfully, they bring elegance and clarity to what is otherwise a cluttered mess of loops and conditional statements. A potent built-in function can often stand in for an entire block of code, capturing its utility and gaining efficiency targets immediately.
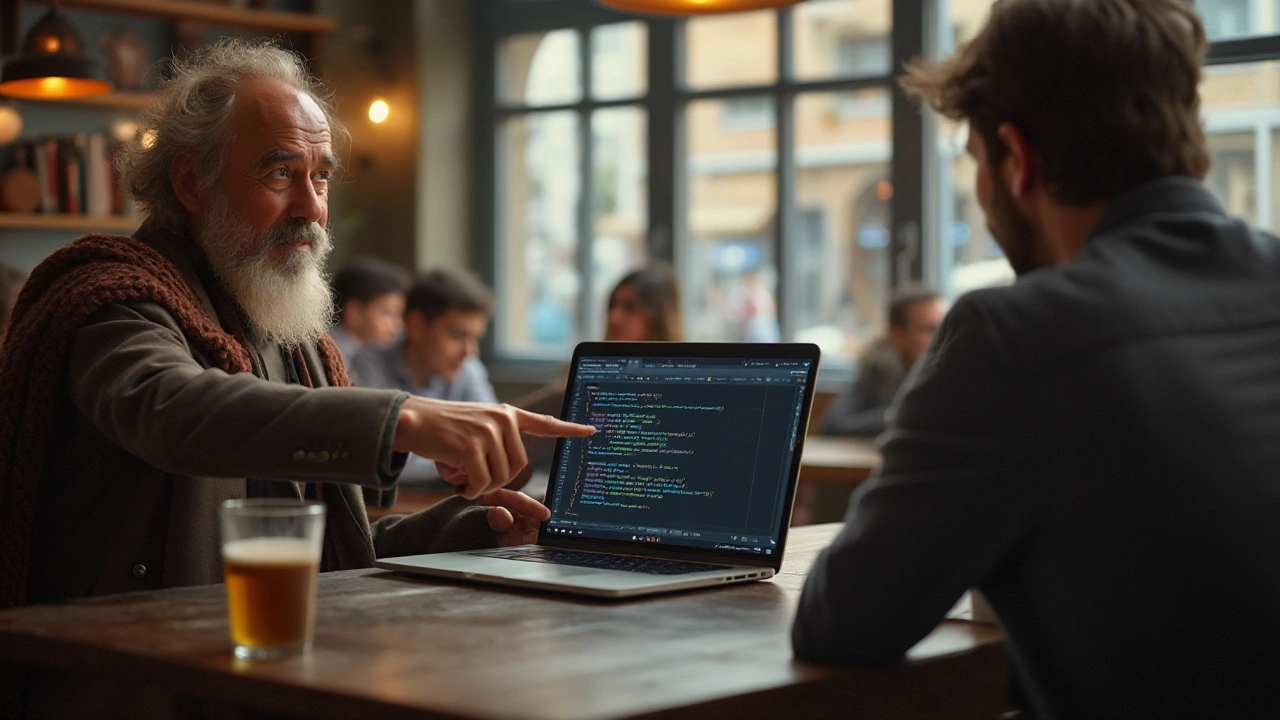
Optimizing Code with Generators
When striving for efficient and clean code, Python generators emerge as an essential tool in a programmer’s arsenal. They offer an elegant way to write iterators without the overhead of creating entire data structures in memory. When used wisely, generators enable you to improve your code’s performance and readability. Rather than generating and storing all the values in one go, generators produce items on the fly, which in essence is memory-efficient. This means you can loop through a large dataset even on devices with limited RAM capacity, providing the dual benefit of speed and resource management.
Take, for example, the time-honored technique of lazy evaluation, which is a fundamental concept underpinning generators. With lazy evaluation, Python generators produce data as it’s required, not all at once. This on-demand generation is one reason why they’re so memory-friendly, especially when deploying code across environments where system resources are constrained. Just imagine processing a gigantic log file or sifting through extensive real-time data streams. Generators make these complex tasks manageable by harnessing Python’s efficient built-in iterator support.
To fully appreciate the potency of generators, it’s helpful to understand their uniqueness compared to traditional functions. A typical function runs from start to finish, whereas a generator remembers its state between iterations. It kicks off execution when next() is called and stops at the yield command, saving its state and resuming from that point upon subsequent calls. This feature gives developers a flexible way to pause and resume execution, making generators perfect for managing pipelines of tasks where the workload varies over time or depends on unpredictable inputs. As developer Brad Miller once observed, “Generators are all about producing the values you need without cluttering up memory with ones you don’t.”
"Generators are all about producing the values you need without cluttering up memory with ones you don’t." – Brad Miller
Utilizing Python’s generator expressions can further streamline your code. Forged in the same spirit as list comprehensions, generator expressions allow concise yet powerful loop structures, but only return one item at a time. This small shift in how data is processed can vastly improve efficiency in scripts where speed and scalability are paramount. Many developers laud this feature as a game-changer because it bridges the gap between simplicity and power. You can often replace complex loops with a single line of readable code, effectively reducing potential bugs and saving on debugging time, a double win for any software project.
The true strength of Python generators often shines through in their lesser-known uses. For example, they are indispensable during infinite sequences or working with potentially infinite data. Not to mention, they harmonize beautifully with other Python features like functools’ reduce or itertools functions which are designed to handle large volumes of data efficiently. Moreover, generators play well with pipelines where you can chain logical operations without preemptively consuming data, ensuring that your code always executes with optimal resource allocation.
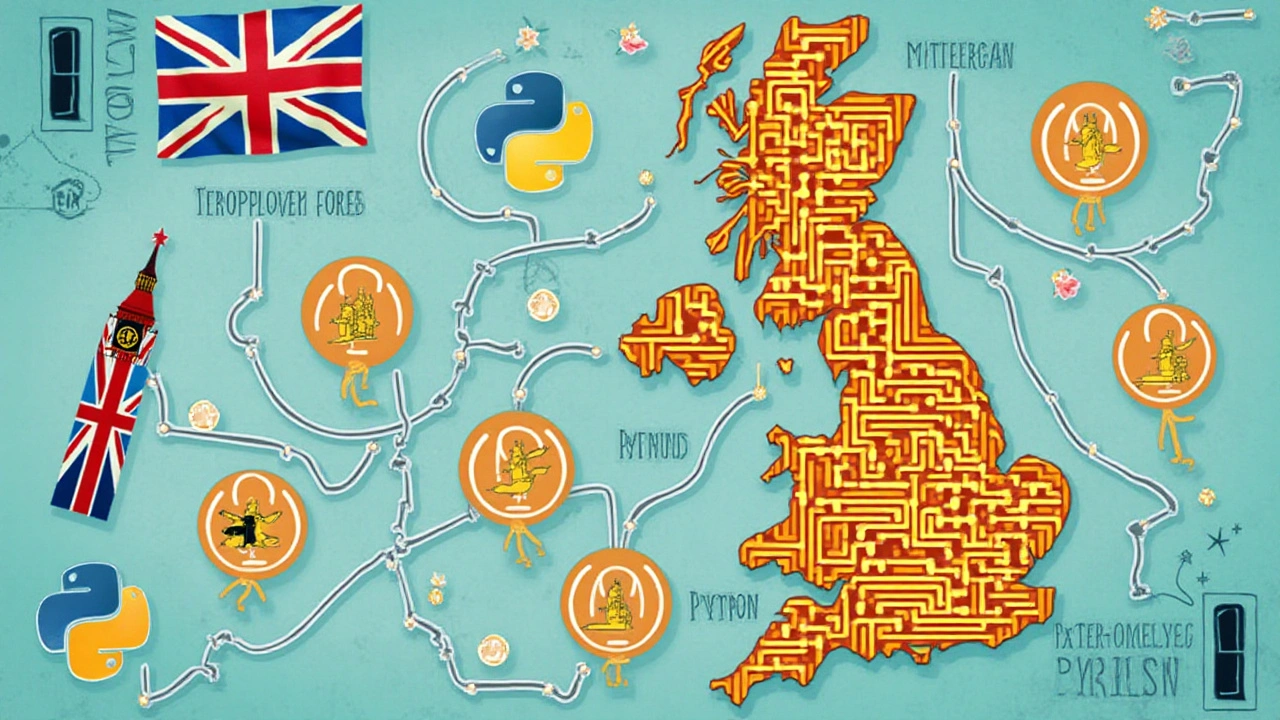
Enhancing Debugging Skills
Debugging is often seen as the rather less glamorous side of coding, yet it remains an essential skill that distinguishes good developers from the great ones. When working with Python tricks, a nuanced understanding of debugging can save time and prevent headaches. One of the strengths of Python is its comprehensive error messages, which provide detailed insights into what's gone wrong and where. Learning to dissect these messages effectively can be your first line of defense in tackling bugs.
It's vital to adopt a systematic approach to debugging. Begin by replicating the issue consistently. This isn't always straightforward, especially with unpredictable bugs, but consistent replication is the cornerstone for thorough problem-solving. Once you've nailed down the errant behavior, zero in on the specific section of your code that's raising the red flags. Utilizing comments can be beneficial here; by systematically commenting out code blocks, you can isolate and identify the problematic sections.
An often underutilized tool is Python's in-built pdb
debugger. It's a powerful ally, providing an interactive debugging environment. With pdb
, you can set breakpoints, inspect variables, and step through your code line by line. By embracing pdb
, developers can gain a deeper understanding of their script's flow and identify logic errors more efficiently. Remember, practice and familiarity with such tools can often be the difference between superficial fixes and definitive solutions.
"Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it." - Brian W. KernighanSome developers find it beneficial to pair their debugging efforts with automation tests. Such tests can catch bugs early and support ongoing maintenance by verifying functionality against expected outcomes. Implementing unit tests helps confirm that each module of your code behaves as intended under various conditions.
Keeping an eye on performance metrics can also be enlightening, especially when debugging code tied to real-world applications. Understanding how efficiently your code runs, how much memory it uses, and where the bottlenecks are can provide significant clues during the debugging process. In some cases, rewriting portions of your code to align with coding best practices can lead to more maintainable, bug-free applications in the long run.
For collaborative projects, code reviews play a crucial role in the debugging process. Having another set of eyes on the code often brings a fresh perspective. Experienced teammates might spot subtle errors that escape your notice during late nights over the keyboard. Incorporating such feedback allows you to learn and expand your repertoire of programming tips while enhancing your debugging prowess.