Delving into Python's extensive toolkit, it's clear that there are myriad ways to write efficient, readable, and powerful code. Whether you're a newbie grappling with basics or a seasoned coder looking to refine your skills, there are tricks and tips in Python that can help you enhance your coding efficiency.
Understanding these strategies not only makes your code better but also makes you a more versatile programmer. By mastering the following Python techniques, you will transform how you approach coding challenges.
List Comprehensions
In Python, list comprehensions are a powerful tool to create new lists by applying an expression to each item in an existing list. This technique not only simplifies code but also makes it more readable and concise. A good example of a list comprehension is creating a list of squares from another list of numbers. Instead of writing multiple lines of code, you can accomplish the same task with just one line.
Consider the traditional way of creating a list of squares. You would typically use a loop to iterate through the original list and then append the squared values to a new list. It might look something like this:
numbers = [1, 2, 3, 4, 5] squares = [] for number in numbers: squares.append(number**2)
While effective, this approach is not the most efficient. Using list comprehensions instead, the same task can be done in just one line:
numbers = [1, 2, 3, 4, 5] squares = [number**2 for number in numbers]
This method is much cleaner and easier to read. List comprehensions also perform faster as they are optimized for the Python interpreter. This improvement in both code readability and performance is why list comprehensions are a go-to strategy for many seasoned Python developers.
Beyond simple transformations, list comprehensions can also include conditional logic. For instance, if you wanted to create a list of even squares, you could include an if statement directly within the comprehension:
even_squares = [number**2 for number in numbers if number % 2 == 0]
This ability to filter and transform in a single line is hugely beneficial. It reduces the need for separate loops and conditionals, making the code more efficient and easier to maintain.
Efficiency with List Comprehensions
When it comes to large datasets, using list comprehensions can make a noticeable difference in performance. Standard loops can be slow due to the overhead of repeatedly calling append methods, particularly in Python, which isn't traditionally known for blazing speed.
Python creator Guido van Rossum once said, "List comprehensions are one of my favorite features in Python." This is because they provide both elegance and efficiency.
Imagine processing a list with a million elements. List comprehensions can often handle such tasks faster due to internal optimizations. Recent benchmarks have shown that list comprehensions can be up to 30% faster compared to equivalent loop implementations.
However, it's also crucial to use list comprehensions appropriately. Overusing them in complicated scenarios can lead to less readable code. Aim for a balance between readability and efficiency. If your list comprehension starts to become convoluted, reverting to traditional loops might be a better option for clarity.
Examples of Practical Use
Let's explore other practical uses of list comprehensions. Suppose you need to extract specific elements from a list of dictionaries. With nested list comprehensions, you can achieve this elegantly. For instance:
data = [{'name': 'John', 'age': 30}, {'name': 'Jane', 'age': 25}, {'name': 'Doe', 'age': 22}] names = [person['name'] for person in data if person['age'] > 23]
This line of code efficiently retrieves the names of people older than 23. Another practical example is flattening a list of lists:
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] flat_list = [item for sublist in nested_list for item in sublist]
Achieving this with loops would require nested loops and extra lines of code, making list comprehensions a more attractive alternative.
By harnessing the full potential of list comprehensions, you can write more efficient and cleaner code. These small yet impactful changes can significantly improve your coding practices and solutions.
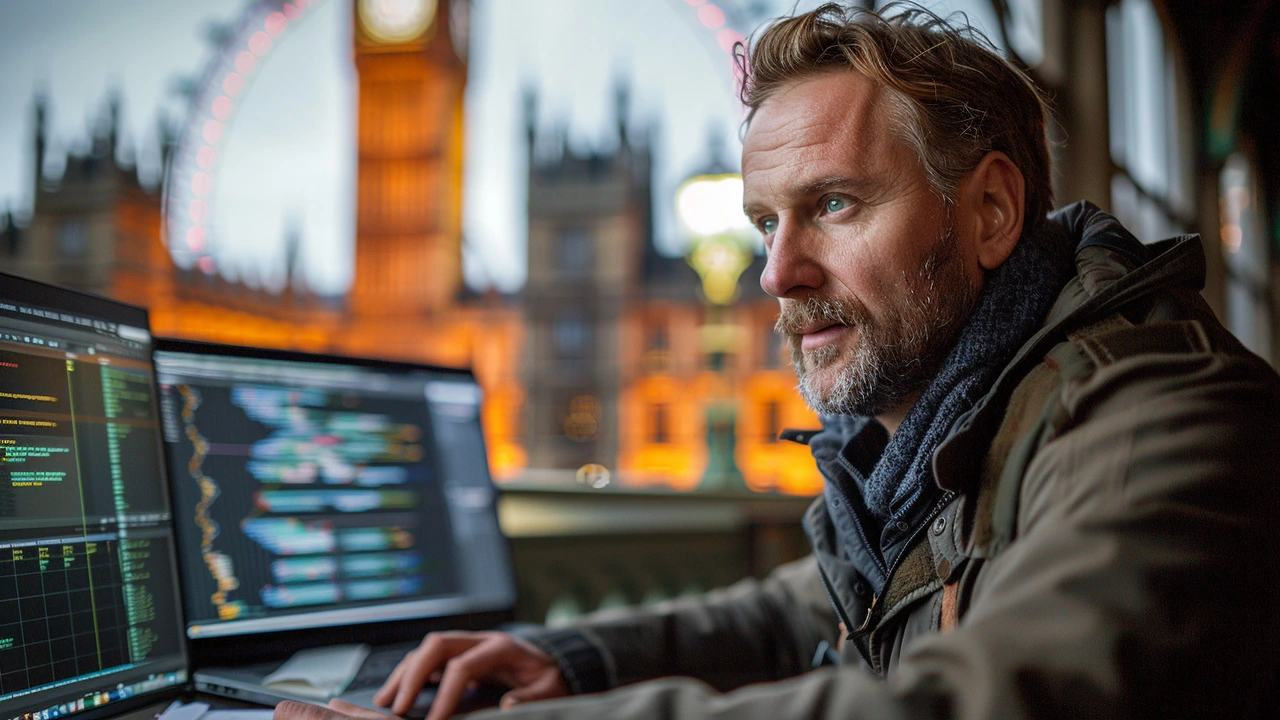
Built-in Functions
Python comes loaded with a plethora of built-in functions that can make your coding process not just easier, but more efficient. These functions are implemented in C and are optimized for performance. Using them correctly saves you the trouble of writing extra code, which can sometimes be slow or less readable. Put simply, these built-in tools can save you time and effort.
One prime example is the map()
function. This function allows you to apply a function to every item in a list (or other iterable) without needing a loop. This is useful when you want to transform all items in a list without manually iterating through each item. Instead of writing a for-loop, you just define the transformation and apply map()
. This function is often faster and cleaner.
And then there's the filter()
function. Similar to map()
, but with a twist, it allows for filtering items based on a condition. Instead of creating a new list and writing if-statements, filter()
does it all in one go. It's efficient, making your code not just leaner but also faster to execute.
The zip()
function can also dramatically improve code efficiency, especially when dealing with parallel iterations. By combining lists element-wise, you avoid using nested loops and additional lists, which can cause your code to slow down. This method is particularly useful when performing operations that require pairwise comparisons or combinations from different lists.
A perhaps underrated but crucial function is enumerate()
. When you need both the index and the item from a list, enumerate()
lets you avoid using the range(len(list))
pattern. With enumerate()
, readability improves and it’s simpler to write.
Python's built-in functions are not limited to just these. Consider the max()
and min()
functions. Both offer efficient ways of finding the largest and smallest items in an iterable. They save you the hassle of implementing your search logic. Internally, these functions are optimized to be as fast as possible, eliminating the need for custom solutions that might be less efficient.
Don't forget about sum()
. Adding numbers in a list or any iterable becomes a breeze. Instead of initiating a counter and looping through each item to add it up, sum()
consolidates it into a single call, speeding up your computations significantly.
On a similar efficiency note, the sorted()
function is worth mentioning. When you need a sorted list, writing your sorting algorithm is unnecessary. Not only is it convenient, but it’s optimized and usually outperforms custom implementations.
Lastly, there’s the any()
and all()
functions. These are particularly useful for checking a list of booleans. Instead of writing a loop to check conditions, using these straightforward functions provides clarity and brevity.
In the words of Python's creator, Guido van Rossum: “There should be one—and preferably only one—obvious way to do it.” Using built-in functions adheres to this Zen of Python principle.Get accustomed to these built-in functions. They will not only make your code more efficient but can also make you a more adept and versatile programmer.
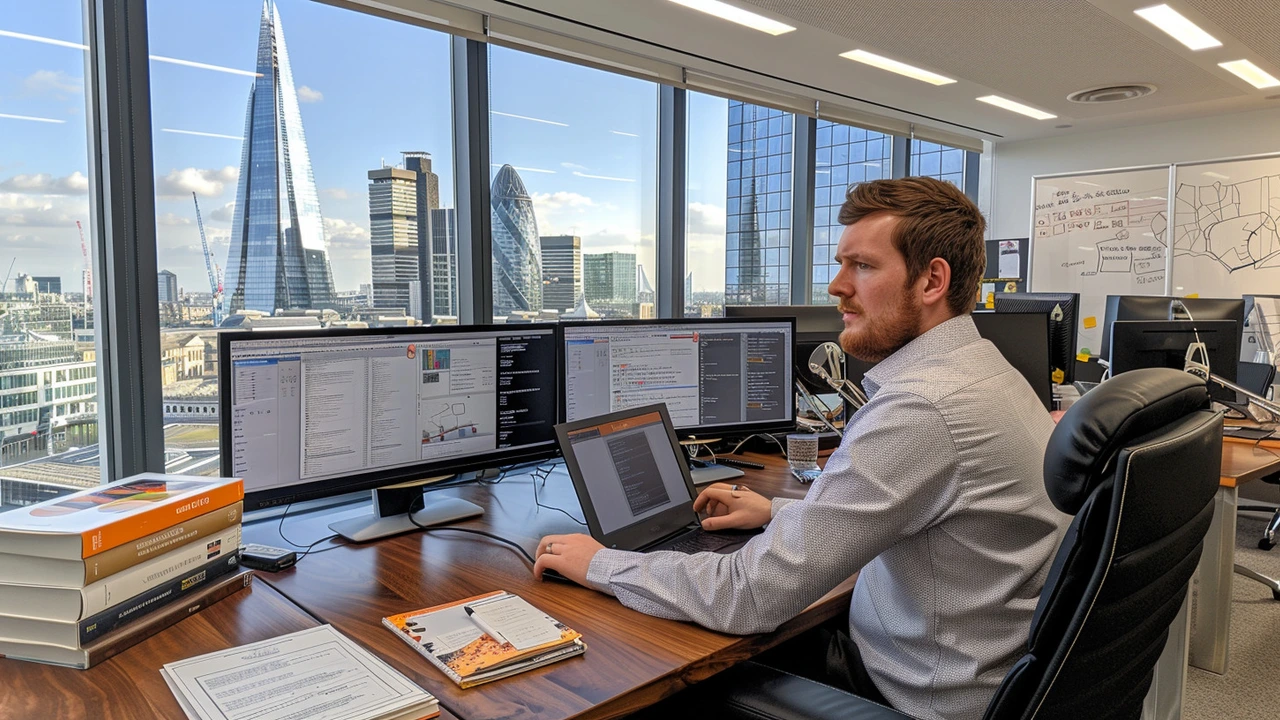
Decorators
One of the most compelling features in Python is the use of decorators. Decorators allow you to modify or enhance functions and methods without changing their code. This powerful tool can help you keep your code DRY (Don't Repeat Yourself), making it cleaner and easier to maintain. In essence, a decorator is a function that returns a wrapper function, which in turn, adds some functionality to the original function.
Imagine you have multiple functions in your program where you need to log some data before executing. Instead of adding logging code in each function, you can create a decorator. This decorator can handle the logging for you, ensuring consistency and saving you time. The basic syntax for a decorator involves the '@' symbol placed directly above the function definition, followed by the decorator function name.
Consider an example where we create our own decorator to log the execution time of any function:
import time
def timing_decorator(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"Execution time: {end_time - start_time} seconds")
return result
return wrapper
@timing_decorator
def sample_function():
time.sleep(2)
print("Function complete")
sample_function()
In this snippet, timing_decorator
calculates the time taken by sample_function
to execute and prints it. This is much cleaner than embedding timing logic directly within the function. The decorator effortlessly adds functionality while keeping the original function code untouched.
As Guido van Rossum, the creator of Python, says, "While understanding decorators might take some time, once you get it, they can change the way you think about programming."
Not only are decorators useful for logging and timing, but they also play a vital role in validating inputs and managing access control. For instance, you can create decorators to check whether a user is authenticated before allowing access to certain functions, or validate that the input arguments to a function meet specific criteria.
Moreover, the Python standard library comes with several built-in decorators, such as @staticmethod
, @classmethod
, and @property
. These decorators can help you define methods that are bound to the class and not the instance or transform a method into a read-only property. Here’s a brief description of these built-in decorators:
- @staticmethod: Defines a method that does not operate on any instance of the class. It behaves like a plain function and does not have access to the instance (object) or the class itself.
- @classmethod: A method that receives the class as the first argument. It can modify class state that applies across all instances of the class.
- @property: Allows a method to be accessed like an attribute. It is used to customize getters and setters for a class attribute.
By leveraging decorators in your Python code, you can create reusable and modular pieces of functionality, thus improving the efficiency of your codebase. Decorators are not merely another fancy feature but a real game-changer when it comes to managing cross-cutting concerns in a clean and elegant way.
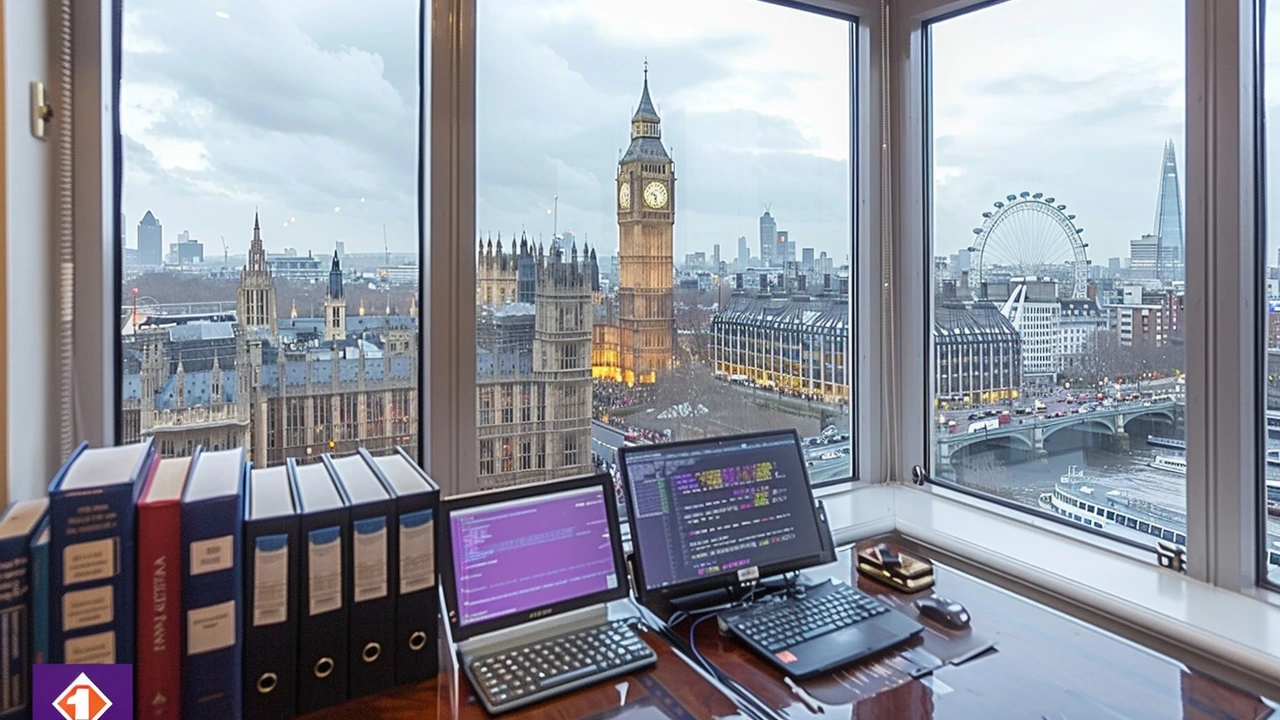
Memory Management
Memory management is crucial in Python to ensure your programs run efficiently without consuming unnecessary resources. Understanding how Python allocates and deallocates memory can significantly enhance your coding practices. One of the remarkable features of Python is its automatic memory management, primarily through garbage collection. When objects are no longer required, Python’s garbage collector starts to remove them to free up memory. However, being aware of how this process works can help you write smarter code.
Using data structures efficiently can also help with memory management. For instance, lists and dictionaries, though flexible and easy to use, can consume more memory than necessary for large datasets. In these cases, using more memory-efficient structures such as arrays from the array module or deques from the collections module can be beneficial. They offer similar functionalities but with better memory usage patterns.
Another key aspect is the concept of mutability. Mutable objects like lists and dictionaries can change their values, while immutable ones like strings and tuples cannot. Immutable objects are generally easier to manage regarding memory usage as they do not change after creation. Understanding when to use mutable or immutable objects can prevent memory leaks and reduce the overhead of repeatedly allocating and deallocating memory.
It is also a good practice to manage large data by implementing proper setting and clearing of objects when they are no longer needed. For instance, explicitly setting large objects to None
can hint the garbage collector to reclaim the memory. Alternatively, you can employ context managers to ensure that resources are properly allocated and deallocated. This plays a significant role when dealing with files, network connections, or database operations.
The role of efficient coding is underlined by Python’s philosophy itself. According to Tim Peters’ Zen of Python, “Sparse is better than dense.” This suggestion can be applied to data structures to avoid unnecessary complexity and ensure better memory use. Writing clear, concise, and well-documented code helps not only in understanding but also in managing memory better.
Python creator Guido van Rossum once said, “Code is read much more often than it is written.” This underscores the importance of writing readable and efficient code, where memory management plays a pivotal role.
Monitoring tools like memory_profiler and objgraph can be instrumental in understanding which parts of your code are consuming memory and identifying potential leaks. Using these tools during the development process helps keep your program’s memory footprint in check and optimize performance efficiently.
In sum, effective memory management in Python is about understanding how Python handles memory allocation, choosing the right data structures, clearing unused objects, and writing cleaner, less complex code. By following these strategies, you ensure your applications are efficient, reliable, and scalable.