Learning to program is just the beginning; becoming a successful coder involves mastering a myriad of tricks and techniques that make your code more efficient, readable, and resilient. Understanding these programming tricks isn't just about writing code that works but writing code that works well and stands the test of time.
In this article, we'll delve into some essential programming tricks that can elevate your coding skills. From mastering problem-solving techniques to effective debugging strategies, these insights will provide you with a robust toolkit for tackling any coding challenge.
So whether you're new to the programming world or looking to enhance your current skill set, these tips and tricks could be the X-factor that sets you apart in your coding journey.
- Mastering the Art of Problem-Solving
- Using Version Control like a Pro
- Code Readability and Clean Code
- Leveraging IDE and Text Editor Features
- Staying Updated with Industry Trends
- Effective Debugging Techniques
Mastering the Art of Problem-Solving
Every great programmer understands that problem-solving is at the heart of coding. Before diving into writing code, it's crucial to break down the problem you're trying to solve. Think of this as laying the groundwork before constructing a building. This means understanding the problem in depth and considering multiple ways to approach it.
One effective technique is to start with the simplest version of the task. For instance, if you're designing an algorithm to sort a list, understand how simple sorting algorithms like bubble sort work before moving on to more complex ones like quicksort. This foundational knowledge builds your confidence and skill set, laying down a strong framework for tackling harder problems later.
Brainstorming is another potent tool. Mind maps can be incredibly helpful during this phase. Draw out all potential variables and terms related to the problem. This visual representation allows you to see the entire landscape and spot connections and solutions you might have missed otherwise.
Critical thinking is essential in identifying the most efficient solutions. Always ask yourself, "Is there a better way to achieve this?" Not every solution needs to be perfect, but striving for more optimal ones ensures better performance and scalability.
Another trick is to step away for a bit if you feel stuck. Interruptions can sometimes lead to eureka moments when you're least expecting them. Jessica often tells me this: “Take a walk, clear your mind, and then come back to it.” It has saved me countless hours of frustration.
Using pseudocode can also simplify complex problems. Before writing the actual code, draft a plain-language outline of how the solution should work. This method aids in understanding and spotting potential issues early on, without getting bogged down by syntax.
Collaboration can significantly boost your problem-solving abilities. Participate in coding communities and forums. Engaging with others brings fresh perspectives and insights that you wouldn't have considered on your own. Plus, explaining your thought process to someone else can clarify your own understanding.
Many seasoned developers keep a “toolbox” of standard algorithms and data structures. Knowing these well can make problem-solving faster and more efficient. Algorithms like binary search, hash tables, or dynamic programming paradigms are essential utilities for any coder's repertoire.
A fascinating fact: Researchers found that programmers are more effective problem solvers when they take breaks and are mindful of their mental health. According to a study published in the Journal of Applied Psychology, brief diversions can significantly enhance focus and productivity.
“The important thing is not to stop questioning. Curiosity has its own reason for existing.” – Albert Einstein
Finally, remember that every problem has multiple facets and layers of complexity. Stay curious and persistent. Learning to embrace challenges makes you not just a better programmer, but a more resilient and adaptive one as well.
Using Version Control like a Pro
Understanding how to use version control systems (VCS) effectively is crucial for any programmer looking to succeed in the field. The most popular VCS today is Git, and learning to use it properly can save you countless hours and headaches. Think of version control as a safety net that not only keeps track of every change but also allows you to collaborate seamlessly with other developers. Mastering version control is like knowing how to navigate through the dense forest of code without losing your way.
One of the first steps in using Git like a pro is understanding its branching model. Branching allows you to create isolated environments for your work. This means you can work on a new feature or fix a bug in a separate 'branch' without affecting the main codebase. When you're confident with the changes, you can merge them back into the main branch. This might sound simple, but keeping your branches organized is key to avoiding merge conflicts.
Another important aspect to consider is writing meaningful commit messages. Each commit should capture a specific change or set of changes, and the message should explain what has been done. Clear, concise commit messages are invaluable when you or another developer need to understand the history of a project. It's like leaving breadcrumbs for others to follow, making it easier to track and revert changes when something goes wrong.
GitHub, GitLab, and Bitbucket are some of the popular platforms that provide additional features for Git, such as issue tracking, code review, and continuous integration. Utilizing these tools can significantly streamline your workflow. For example, code reviews not only help catch bugs early but also provide an excellent learning opportunity by exposing you to different coding styles and best practices.
Incorporating version control into your daily routine also involves some essential commands and techniques. Knowing how to use `git stash` can be a lifesaver when you need to switch tasks quickly. This command saves your current work and lets you revert to a clean state. Once you're ready to resume, you can retrieve your stashed changes and continue working. Similarly, understanding the difference between `git pull` and `git fetch` can help you manage your project better. `Git pull` integrates your local repository with remote changes, while `git fetch` simply updates the local metadata, giving you a chance to review changes before merging.
“Mastering Git is not only about knowing the commands but understanding the underlying model and being able to collaborate effectively with your team,” says Scott Chacon, the co-author of 'Pro Git.'
Collaboration is where version control truly shines. When working in a team, it's important to establish a branching strategy that fits your workflow. Some teams prefer the Git Flow model, which distinguishes between feature, release, and hotfix branches. Others might opt for a simpler workflow with just a `main` and `develop` branch. The key is to be consistent and communicate your approach clearly within your team.
Lastly, don't overlook the importance of backups. Even though version control provides a form of backup, it's wise to periodically back up your repositories to an external location. This adds an extra layer of security and ensures that you don't lose your precious code to unforeseen circumstances. Inset automation into this task can relieve the burden significantly, using scripts or CI/CD pipelines to handle regular backups.
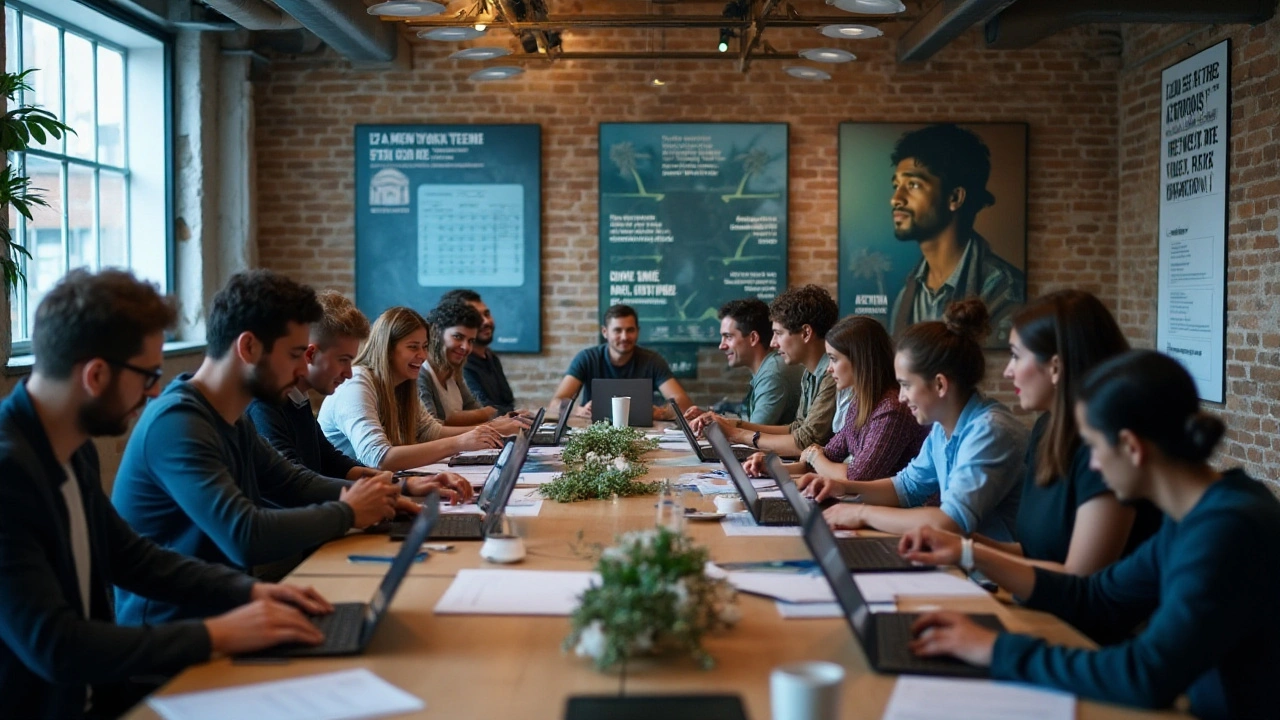
Code Readability and Clean Code
Code readability isn't just a fancy term thrown around by senior developers; it is a critical aspect of software development that significantly impacts your project's longevity and maintainability. Writing clean code means creating something that others (or even your future self) can read and understand without a plethora of comments explaining each line. One might think writing code that works is enough, but readable and clean code can save a team countless hours in the long run.
Think of it this way: when someone else reads your code, they should be able to understand its purpose, logic, and flow without needing to dissect every detail. A well-known principle in the software development world is the 'KISS' principle – Keep It Simple, Stupid. Simplicity doesn't mean reducing the functionality of your code; rather, it involves removing unnecessary complexities.
Use Meaningful Names
One easy and impactful way to improve code readability is by using meaningful variable and function names. A variable name like `userAge` is infinitely more understandable than `x23`. Readers can grasp the significance and usage of `userAge` immediately. This simple trick can make a huge difference, especially in larger projects where hundreds of variables and functions exist.
Comment Sparingly, but Wisely
While some might advocate for heavy commenting, it's important to strike a balance. Over-commented code can be just as troublesome as under-commented code. Instead, aim to write self-explanatory code and use comments to clarify complex logic or to explain why a particular approach was chosen over another. According to a study by Cambridge University, “Code with proper, concise comments helps in reducing the cognitive load on developers”. Yet, excessive commenting can lead to clutter and even misinformation if the comments are not updated with the code.
Consistent Code Formatting
No one enjoys working on a project where every file has different indentation, line spacing, and bracket placement. Establishing a consistent coding style helps streamline the development process. Many integrated development environments (IDEs) allow you to set formatting rules for your codebase, autocompleting spaces or adjusting indents as you type. This minimizes errors and discrepancies in code formatting, making it easier for teams to collaborate efficiently.
Apply the DRY Principle
The DRY (Don't Repeat Yourself) principle is a cornerstone of clean code. Repeating the same code in multiple places makes it harder to update and maintain. Instead, aim to write reusable functions or modules that can be called whenever necessary. This not only reduces redundancy but also simplifies debugging and testing, as changes are contained within a single function instead of scattered throughout the code.
“Programs must be written for people to read, and only incidentally for machines to execute.” – Harold Abelson, Computer Scientist
Utilize Linters and Code Analysis Tools
Tools like ESLint for JavaScript or Pylint for Python help in identifying potential errors and ensuring that your code adheres to predefined style guidelines. These tools scan your codebase and flag deviations from your coding standards, making it easier to maintain consistency and catch errors before they make it into production. Especially for large projects, incorporating a linter into your workflow can significantly boost code quality.
Readable Code: An Investment
In essence, investing time in writing readable and clean code can pay dividends by making your work more maintainable, easier to debug, and pleasant to read. Remember, your code is not just for today; it’s for tomorrow, next week, and potentially for years to come. By adopting these practices, you'll not only become a more proficient programmer but also a more valuable team member in any software development project.
Leveraging IDE and Text Editor Features
Every programmer has their favorite Integrated Development Environment (IDE) or text editor. These tools offer a wide range of features that can significantly enhance your coding routine. Understanding and utilizing these features effectively is crucial for becoming a more efficient and productive coder.
One of the most essential features is the code autocomplete. This functionality suggests possible completions for partial code snippets, saving time and reducing typos. It can even suggest entire blocks of code or common idioms, which can be particularly helpful for beginners. For example, in Visual Studio Code, extensions like IntelliSense provide smart completions based on variable types, function definitions, and imported modules.
Another powerful feature is syntax highlighting. This makes your code easier to read and understand by displaying different categories of terms in different colors and fonts. Syntax highlighting helps spot errors early, making it easier to debug and review your code. Most text editors, such as Sublime Text and Atom, offer customizable syntax highlighting to suit different programming languages and developer preferences.
The integrated terminal in IDEs like PyCharm or VS Code can be a game-changer. It allows developers to run scripts, manage version control, and install packages without leaving the coding environment. This seamless integration saves a considerable amount of time by reducing the need to switch between different applications.
Version control integration is another feature that can significantly boost your productivity. With tools like Git integrated directly into your IDE, you can manage your repositories, commit changes, and review history without ever leaving your coding environment. This integrated approach ensures that version control becomes a natural part of your workflow rather than an extra chore.
Most modern IDEs and text editors also come with built-in debugging tools that let you set breakpoints, inspect variables, and step through your code line by line. These features are invaluable for identifying and fixing bugs. For example, JetBrains' ReSharper offers advanced debugging features that allow you to step through code, even when it spans multiple threads.
According to a study from JetBrains, developers who use IDEs with advanced features like code refactoring and in-built testing are 40% more productive than those who do not. This productivity boost underscores the importance of selecting a powerful and feature-rich IDE.
Refactoring tools are incredibly helpful for keeping your code clean and maintainable. These tools can automatically rename variables, extract methods, and perform other syntax transformations, ensuring your codebase remains organized. For example, Eclipse offers a variety of refactoring tools that make it easier to manage and update your code.
Project management features in IDEs like NetBeans can help you organize your code files, track tasks, and manage dependencies. These features ensure that everything is in its right place, making it easier to navigate and understand your project.
Personal customization options can also play a significant role in improving your workflow. Many text editors support various themes, plugins, and extensions that you can tailor to fit your specific needs. For example, Atom's wide range of community plugins can be used to add features like live previews, advanced search, and even collaborative coding.
In addition to these features, consider exploring the potential of keyboard shortcuts. Most IDEs and text editors allow you to perform common tasks with simple keyboard combinations, speeding up your workflow. For example, keyboard shortcuts for commenting out lines, duplicating code, or navigating between files can save you considerable time.
Leveraging these powerful features can make the act of coding more of a breeze. By understanding and properly using the features available in your chosen IDE or text editor, you can code more efficiently, debug more effectively, and manage your projects with much greater ease. So take the time to explore and master your tools; they really can make all the difference in your coding journey.
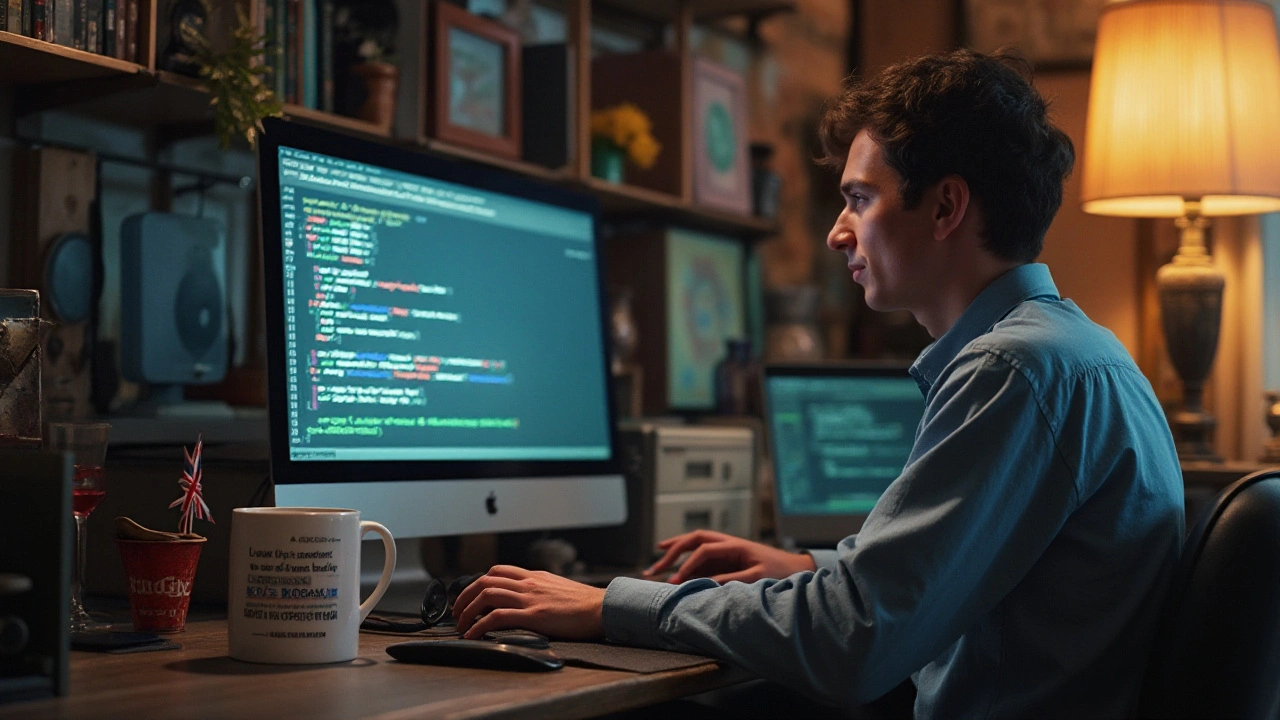
Staying Updated with Industry Trends
In the ever-evolving world of technology, keeping up with industry trends is crucial for any programmer. New languages, libraries, and frameworks are constantly emerging, and staying current is not just about knowing the latest buzzwords. It's about understanding the landscape so you can make informed decisions in your work.
The speed at which the field changes can be overwhelming. Blogs, podcasts, newsletters, and social media are valuable resources. Blogs by industry leaders such as Martin Fowler or Kent Beck can provide in-depth analysis and practical insights. Podcasts like 'Coding Blocks' or 'Syntax' offer discussions on various topics, from best practices to new technologies. Following key figures on Twitter or LinkedIn can also help you stay in the loop.
Attending conferences and meetups can be another excellent way to stay updated. Events like PyCon, JavaOne, or local meetups offer the chance to hear firsthand from experts. Networking with peers can provide insights that you might not get from just reading articles or listening to podcasts. Sometimes, these interactions can lead to job opportunities or collaborations on exciting projects.
Training and certification programs are other methods of keeping your skills up-to-date. Websites like Coursera, Udemy, and edX offer courses taught by industry experts. Certifications from recognized organizations can add a stamp of credibility to your resume. They can demonstrate your commitment to your career and your ability to stay current.
Reading research papers and academic journals, like those from ACM or IEEE, can help you understand the theoretical foundations of new technologies. This can be especially useful if you are working on cutting-edge projects or considering a career in research or academia. The Rigorous Reading approach can also help you read and digest complex material more effectively.
Sometimes, it’s the little things that can make a big difference. For instance, setting up Google Alerts for topics you're interested in can help you stay updated without too much effort. Using tools like Feedly to organize your reading material can make your information intake more manageable. By dedicating some time each week to read or listen to new material, you can ensure you’re not falling behind.
Participating in online communities such as Stack Overflow, Reddit's r/programming, or specialized Slack groups can provide practical insights and peer support. These platforms allow you to ask questions, share your knowledge, and learn from others' experiences. The collaborative nature of these communities can be incredibly beneficial in staying updated and solving real-world problems.
"Staying current isn't just about knowing the new terminology; it's about understanding how the landscape is shifting so you can adapt and make informed decisions," says Martin Fowler.
Lastly, contributing to open-source projects can be a fantastic way to apply what you've learned and stay updated. Open-source contributions often require you to dive deep into new technologies, face real-world challenges, and collaborate with other experts. This hands-on experience can be invaluable and can also help you build a robust portfolio.
Effective Debugging Techniques
Debugging is an essential skill in programming, often overlooked until a bug rears its ugly head. It's not just about finding and fixing errors; it's about understanding why they occurred in the first place. To debug effectively, you need a mix of patience, logic, and a methodical mindset. Let’s dive into some techniques that can help you become more proficient at debugging.
First off, always keep your code readable. Clean code isn't just for show; it makes tracing issues much easier. Implementing consistent naming conventions and organizing your code into smaller, manageable functions can save you a lot of trouble. When variables and functions are named clearly, it’s easier to follow the logic and identify where things might be going wrong.
Next, use logging and print statements wisely. While inserting print statements may seem old school, it’s an effective way to understand what’s happening in your code at different stages. Modern IDEs come equipped with robust logging tools that can help you keep track of your application's state without cluttering your codebase.
Effective coding also involves using a debugger tool. Integrated Development Environments (IDEs) like PyCharm, Visual Studio Code, and Eclipse come with powerful debugging capabilities. You can set breakpoints, inspect variables, and step through your code line by line. This helps you pinpoint where an error might be occurring, making your debugging process more efficient.
Divide and conquer is another useful method. If you're dealing with a complex codebase, isolating the problematic section can make the debugging process less overwhelming. Break down your large functions or modules into smaller, testable units. This way, you can test each unit individually, which makes it easier to identify where the bug might be lurking.
Leverage version control systems like Git. Version control allows you to keep track of changes made to your codebase. If a bug is introduced, you can easily trace back to the commit that caused the issue. This feature is particularly useful when collaborating in a team, as it provides a historical record of code changes and who made them.
Check for common mistakes. Sometimes, the bug is something very basic, like a typo or an off-by-one error. Before diving deep, make sure to cross-check simple issues. It can save you time and mental energy, allowing you to reserve your critical thinking for more complicated problems.
An interesting fact: studies have shown that developers spend as much as 50% of their time debugging. Given this considerable investment, it’s worth honing your debugging skills. The better you become at debugging, the faster you can return to writing new feature code. Chris Pine, an author and programming advocate, aptly said,
“The best method for debugging is to write code in the first place that is easy to debug.”
Finally, don’t underestimate the value of a fresh perspective. When stuck on a stubborn bug, stepping away for a short time can be surprisingly effective. Alternatively, having a colleague look at the code can provide new insights. Sometimes, another set of eyes is all you need to uncover the logical oversight.
To summarize, making your code readable, using logging and debugger tools, isolating problematic sections, leveraging version control systems, checking for common mistakes, and seeking a fresh perspective are fundamental programming tips for effective debugging. Mastering these techniques will make you a more efficient and capable developer, ready to tackle any coding challenge that comes your way.