Programming has become an essential skill in today's digital age, opening doors to countless opportunities and innovations. Whether you're interested in developing software, analyzing data, or creating mobile apps, understanding the fundamentals of coding can significantly boost your career prospects.
In this article, you'll find a detailed guide tailored for both beginners and those looking to polish their coding skills. By the end, you'll be equipped with the knowledge and tools to embark on or advance your programming journey.
- Introduction to Programming
- Choosing Your First Programming Language
- Basic Programming Concepts
- Writing Your First Program
- Common Pitfalls and How to Avoid Them
- Resources for Continued Learning
Introduction to Programming
At its core, programming is the art of telling a computer what to do through a set of instructions. Every time you use a smartphone app, play a video game, or browse the internet, you're interacting with the result of countless lines of code written by programmers. The ability to code isn't just for computer scientists or software developers; it's a skill that can benefit people in various fields, from finance to design and beyond.
The story of programming dates back to the early 19th century with Ada Lovelace, often considered the first computer programmer. She worked on Charles Babbage's early mechanical general-purpose computer and created an algorithm intended to be processed by a machine, making her a pioneer in the field. Jumping forward to the modern day, programming languages have evolved immensely, offering a wide range of applications and functionalities.
Learning programming might seem daunting at first, but it's a process that becomes more intuitive with practice. One of the fundamental aspects of programming is understanding the syntax and logic of a language. Syntax refers to the set of rules that define the structure of a language, while logic involves the way you arrange those rules to perform specific tasks.
Python, JavaScript, and Java are some of the most popular programming languages. Python is known for its readability and simplicity, making it a favorite among beginners. JavaScript is essential for web development, allowing dynamic content to be created on websites. Java, meanwhile, is a versatile language used in various applications, from mobile apps to large-scale enterprise systems.
Steve Jobs once said, "Everyone should learn how to program a computer because it teaches you how to think." Programming indeed sharpens problem-solving skills as you learn to break down complex problems into smaller, manageable parts. This analytical thinking is valuable not only in tech but in everyday life, helping you approach challenges more logically.
Programming also fosters creativity, enabling you to bring your ideas to life through code. If you can think of an app, game, or tool, you can probably create it with the right programming knowledge. This blend of creativity and logic is what makes programming so rewarding and exciting.
In today's job market, coding skills are highly sought after. According to a report by Burning Glass Technologies, jobs requiring coding skills pay an average of $22,000 more per year compared to non-coding positions. Moreover, coding jobs are also growing 50% faster than the job market overall, highlighting the demand for this essential skill.
Choosing Your First Programming Language
When you decide to learn programming, one of the first important decisions you'll face is selecting a language to start with. Numerous programming languages exist, each with its own unique features and use cases. Understanding which language to choose can be daunting, but it largely depends on your goals and the projects you plan to undertake.
For beginners, it's often recommended to start with a language that is both popular and relatively easy to learn. Python is an excellent choice due to its simple and readable syntax. It's widely used for web development, data analysis, artificial intelligence, and scientific computing. As a result, Python has a vast community and a wealth of resources available for learners.
Another great language for beginners is JavaScript. If you're interested in web development, JavaScript is essential, as it's the backbone of interactive websites. With JavaScript, you can manipulate web content dynamically and improve user experience. Additionally, frameworks like React and Angular are built on JavaScript, enhancing its applicability in building modern web applications.
Considering Your Career Goals
Your career aspirations can also influence your choice of a programming language. If you're aiming for a career in enterprise software development, Java might be a better fit. Java is known for its portability across different platforms, and it's the backbone of many large-scale business applications. It's taught in many computer science programs and has a strong presence in the job market.
On the other hand, if you are looking into system programming or game development, you might consider learning C++. It provides a fine level of control over system resources and memory, which is crucial for performance-critical applications. Though it comes with a steeper learning curve compared to Python, mastering C++ can open doors to opportunities in software and game development.
“Choosing the right programming language involves some research about your own interests and the industry trends. No language is inherently better than others, it’s all about the context and your goals.” — CodeAcademy
Don't feel pressured to make a perfect choice on your first attempt. Many programmers end up learning multiple languages over their careers. The initial selection is merely a starting point; as you grow in your coding journey, you'll discover new languages and technologies that can broaden your skills. As you move forward, consider enrolling in online courses, joining coding communities, and working on projects to gain practical experience.
Practical Steps to Choose a Language
- Identify your interest area, such as web development, data science, app development, or game design.
- Research which languages are most commonly used within that field.
- Look at job postings in your desired field to see which languages employers demand the most.
- Consider starting with a language that has extensive documentation and community support.
- Try experimenting with a few languages briefly to see which one resonates with you the most.
Remember, the best way to find a suitable programming language is to start coding. Don't be afraid to get your hands dirty and explore. The insights you gain from actual practice will be invaluable and guide you towards the language that fits your needs perfectly.
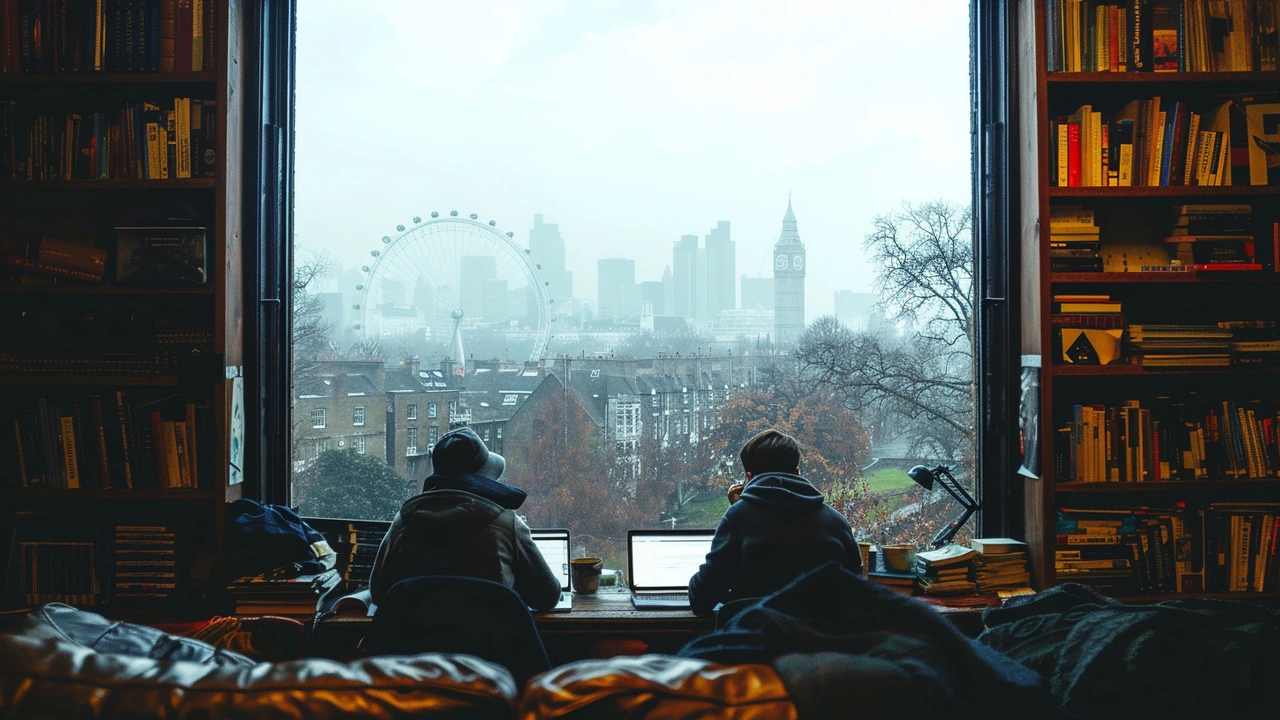
Basic Programming Concepts
When stepping into the world of coding, it's essential to grasp several core concepts that form the foundation of all programming languages. These concepts are like the ABCs of programming, providing the building blocks for creating functional and efficient code. Let's explore some of the most fundamental ideas you'll need to understand.
First, let's talk about **variables**. In programming, variables are used to store data that can change. Think of a variable as a container for information. It can hold numbers, text, or more complex data. Variables are crucial because they make your code more flexible and dynamic. For example, in Python, you can create a variable by simply writing: x = 5
.
Next up, we have **data types**. Every piece of data in a program has a type, such as an integer, float (which refers to decimal numbers), string (which refers to text), or boolean (which represents true or false). Knowing data types helps the program understand how to handle different kinds of data. For instance, adding two strings together will concatenate them, while adding two integers will perform arithmetic addition.
**Control structures** are another vital concept. These include loops (like for
and while
loops) and conditional statements (like if
, else
, and elif
in Python). Control structures enable you to dictate the flow of your program, making decisions based on conditions and repeating actions. For example, you might use a loop to process items in a list or use an if statement to execute certain code only if a condition is met.
Another essential concept is **functions**. Functions are reusable pieces of code that perform a specific task. They help break down programs into manageable parts, making them easier to read, test, and maintain. You can think of functions like recipes; you define the steps once and use them whenever needed. In Python, you define a function using the def
keyword, followed by the function name and parameters.
Error handling is a critical part of programming that ensures your code can handle unexpected situations gracefully. Using **try-except blocks** in Python, for example, allows you to manage errors and keep your program running smoothly. This is particularly important in larger programs where errors are more likely to occur.
Lastly, understanding **arrays** or **lists** is crucial. These structures allow you to store multiple values in a single variable, which is incredibly useful for handling collections of data. For example, a list in Python can be created using square brackets: my_list = [1, 2, 3, 4]
. Lists make it easier to manipulate groups of related data, such as performing operations on each element or sorting the entire collection.
With a basic understanding of these concepts, you're well on your way to becoming proficient in coding. Remember, the key to mastering programming is practice and experimentation. Don't be afraid to make mistakes and learn from them.
Writing Your First Program
Starting your programming journey can be quite exciting. It’s like learning how to express yourself in a new language but with precise rules and infinite creativity. Let's dive into what it takes to write your first program. You often hear about how coding can make you look at problems differently, and that's because the fundamental step of writing your first program compels you to think logically and step-by-step.
To start, choose a simple problem to solve. For instance, many begin with a classic "Hello, World!" program, a tradition in the coding community. This involves writing a program that outputs the text “Hello, World!” to the screen. It might sound trivial, but it teaches the crucial steps from writing code to executing it successfully. Programming languages like Python, known for its simplicity and readability, offers a great starting point.
Here's how you can write the "Hello, World!" program in Python:
- Open your text editor or Integrated Development Environment (IDE) - tools like Visual Studio Code or PyCharm are popular choices.
- Type in the line:
print("Hello, World!")
. - Save the file with a .py extension, such as hello.py.
- Run the program by opening your command line, navigating to the file's location, and typing
python hello.py
.
Running this code will display the text “Hello, World!” in your command line. Congratulations! You've written and executed your first program. It’s a small step but a crucial one in understanding the cycle of coding, from writing to executing and seeing the results.
Creating more complex programs follows the same basic principles. Start simple, experiment, and learn from each program you write. Keep in mind, debugging is an essential part of coding. As you start seeing errors, don't get frustrated. Debugging helps you understand what went wrong and why.
"Programs must be written for people to read, and only incidentally for machines to execute." — Harold Abelson
Understanding the mistakes you make and fixing them sharpens your logical thinking. It’s recommended to comment your code, which involves adding notes within your code to explain what each part does. This makes it easier for you or anyone else reading your code to understand the logic behind it. Commenting is a good habit to cultivate early on.
Additionally, there are platforms like GitHub where you can share your code, collaborate with others, and even get feedback. It's widely used in the industry and can be a valuable resource for improvement. Keep practicing, explore different problems, and try writing programs that solve real-world issues. Gaining proficiency in programming is a gradual journey but every small program you write is a step forward.
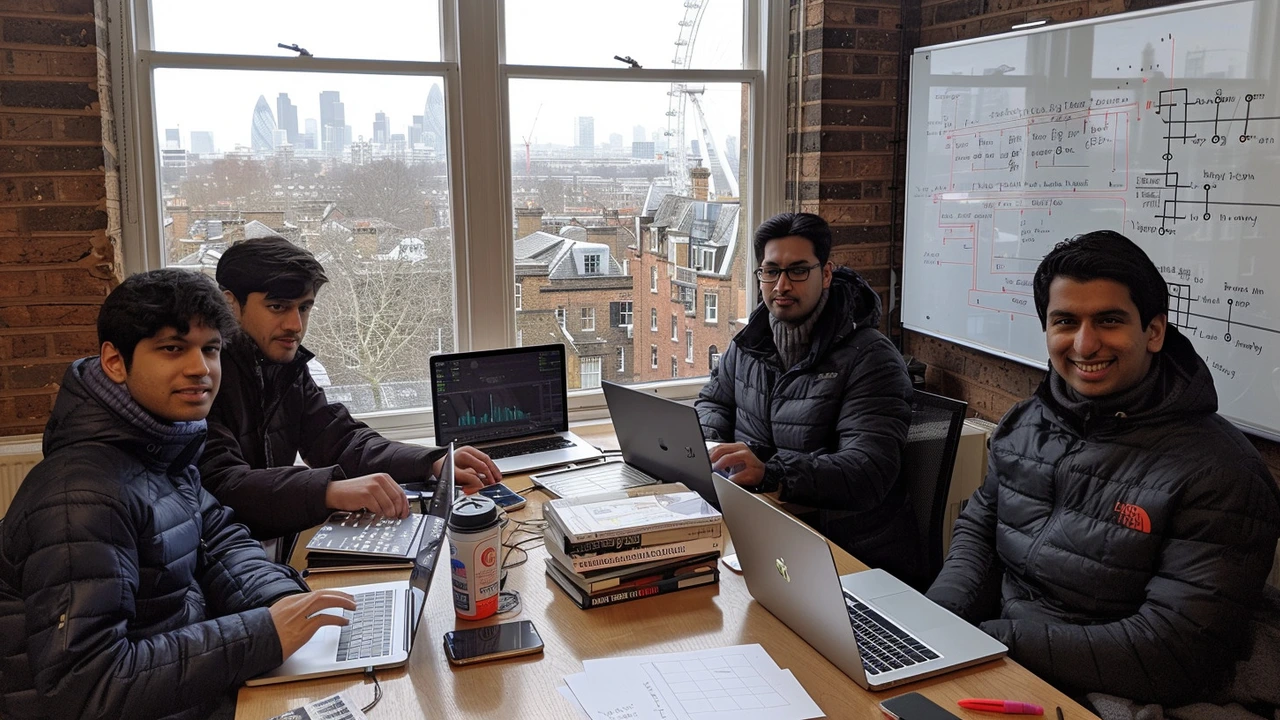
Common Pitfalls and How to Avoid Them
Starting on your programming journey can be both exciting and daunting. Many new coders encounter various obstacles that can slow their progress or even discourage them from continuing. Recognizing these common pitfalls and knowing how to navigate them can make a world of difference in your learning experience.
One common mistake that beginners make is not fully understanding the problem they are trying to solve before jumping into coding. This often leads to incomplete or incorrect solutions. To avoid this, take your time to thoroughly analyze and break down the problem. Write a clear plan or pseudocode outlining the steps you need to take. This approach can significantly streamline the coding process.
Another frequent issue is syntax errors. These are mistakes in the way code is written, which can often cause programs to fail. Beginners should pay close attention to detail, ensuring that every bracket, semicolon, and indentation is in the right place. Using an Integrated Development Environment (IDE) can be extremely helpful, as it often highlights syntax errors, making them easier to identify and fix.
Trying to learn too many languages at once can also be overwhelming. It's crucial to master one programming language before moving on to others. This ensures a solid foundation of programming concepts, which are often transferable across languages. Python, for example, is a great starting point due to its readability and extensive libraries.
Debugging can be a source of frustration for new coders. Many give up too soon when they encounter bugs in their code. However, debugging is a vital skill. Developing a methodical approach to finding and fixing errors is essential. Tools like print statements, logging, and debugging features in IDEs can make this process more manageable.
Not utilizing available resources is another common pitfall. Books, online tutorials, forums, and coding communities are rich sources of knowledge and support. Engaging with these resources can provide new insights and help overcome challenges. Platforms like Stack Overflow and GitHub are excellent places to seek help and find code examples or libraries that can simplify your work.
Skipping comments and documentation is tempting, but it can cause problems in the long run. Properly commenting your code makes it easier to understand and maintain, especially if you return to it after some time. Good documentation becomes beneficial when collaborating with others or even for your future self.
Many beginners also underestimate the importance of testing. Writing tests for your code ensures that it works as expected and helps catch problems early. Automated testing frameworks can be handy and are worth learning. Regularly testing small parts of your code can save considerable time and effort later.
Finally, patience is key. Mastery of programming does not happen overnight. It's important to practice regularly and not be discouraged by setbacks. Consistent effort and a positive mindset are your best allies on this journey.
Resources for Continued Learning
Embarking on your programming journey is just the beginning. Continued learning is crucial for staying relevant in the fast-evolving field of computer programming. With a plethora of resources available, it can be overwhelming to determine where to start. Here's a helpful guide to some of the best resources to keep learning and improving.
Books remain a goldmine of information and are ideal for deep dives into specific programming languages and concepts. Titles like "Clean Code" by Robert C. Martin offer valuable insights into writing efficient code. Meanwhile, books like "The Pragmatic Programmer" by Andrew Hunt and David Thomas teach essential tricks and best practices that can elevate your coding skills.
Online courses have revolutionized the way we learn, providing flexibility and a wide range of topics at our fingertips. Platforms such as Coursera, Udemy, and edX offer courses from top universities and experts in the field. Whether you're looking to pick up Python or dive into machine learning, these courses provide both beginner and advanced content.
Practical experience is another cornerstone of becoming a proficient programmer. Websites like GitHub not only allow you to store and share code but also let you collaborate on projects. Participating in open-source projects can significantly enhance your understanding and expose you to real-world coding challenges.
Community forums and discussion boards are invaluable for troubleshooting and learning from others' experiences. Sites like Stack Overflow provide a platform where you can ask questions and receive answers from seasoned developers. Engaging in discussions helps you learn diverse approaches to problem-solving.
Coding bootcamps are intensive training programs designed to turn novices into job-ready developers. Programs from organizations like General Assembly and Le Wagon focus on hands-on learning and often include career services that assist with job placement. These bootcamps are an excellent way to fast-track your programming skills.
"Learning to code not only means acquiring the skill of writing code but also understanding the conceptual framework that underpins software development." - Douglas Rushkoff
Coding challenges and competitions such as those offered by HackerRank and LeetCode provide an engaging way to practice and refine your skills. These platforms offer a variety of challenges that can help you improve your problem-solving abilities and prepare you for technical interviews.
Lastly, staying updated with the latest trends and technologies is vital. Following tech blogs, subscribing to newsletters, and listening to podcasts can keep you informed. Websites like TechCrunch and Medium offer articles on emerging technologies and industry trends. Podcasts such as "CodeNewbie" and "Software Engineering Daily" are excellent for gaining insights from experts.
Whether you're just starting or you're an experienced coder looking to stay ahead, these resources provide a comprehensive path towards continuous improvement in the world of programming. With dedication and the right tools, mastering coding is an achievable and rewarding goal.