Diving into programming can be both an exciting and daunting journey. With the right guidance, you can become proficient faster than you think. This guide is here to show you how to streamline your learning process and develop effective coding habits.
In the next sections, we'll explore some crucial concepts and techniques that will help you improve your coding speed and efficiency.
Understanding the Basics
Getting a solid grasp on the basics is where every successful journey in programming begins. It may seem like common sense, but you'd be surprised how many budding developers try to skip over these crucial steps and end up creating a shaky foundation that crumbles under the slightest pressure. So, let’s take a peek into why understanding the basics is non-negotiable and how you can effectively tackle it.
First off, learning how to write clean and efficient code starts with a solid understanding of syntax and semantics. Take JavaScript or Python, for example. Both languages have their own syntax rules that must be followed. Even a small error like a missing semicolon or an incorrect indentation can lead to bugs that are hard to track down. Therefore, you need a meticulous eye for detail.
Next up, grasping the fundamental data structures and algorithms is essential. Whether it’s arrays, linked lists, or hash tables, these are the building blocks of all complex applications. Companies like Google and Facebook often test candidates’ understanding of these in their technical interviews. According to a survey by Stack Overflow, over 40% of developers believe that understanding algorithms significantly improves problem-solving skills.
Famed computer scientist Edsger Dijkstra once said, “Simplicity is a prerequisite for reliability.” Understanding and mastering basic programming concepts contribute immensely to writing simple, reliable code.
Start with the Right Resources
Don’t underestimate the importance of quality resources. Textbooks, online courses, and tutorials can all provide valuable knowledge. For instance, CS50 by Harvard is one of the most popular introductory courses available online. It covers everything from basic C programming to SQL database management, providing a well-rounded foundation.
Practice, practice, practice. You can’t expect to become proficient without getting your hands dirty in some actual coding. Websites like LeetCode and HackerRank offer countless exercises that will test your grasp of basic concepts while also sharpening your problem-solving skills. Regular coding practice can significantly improve your speed and efficiency, which are crucial as you progress.
Understand Version Control
Lastly, learning version control is vital, especially when working in a team. Git, one of the most widely-used version control systems, allows multiple people to work on a single project without causing conflicts. Understanding how to use Git effectively from the outset can save a lot of headaches down the line.
By mastering these basic but essential elements, you'll find yourself well-equipped to tackle more complex programming challenges with confidence and ease. Remember that the key to success lies in building strong, fundamental skills that will support you throughout your coding journey.
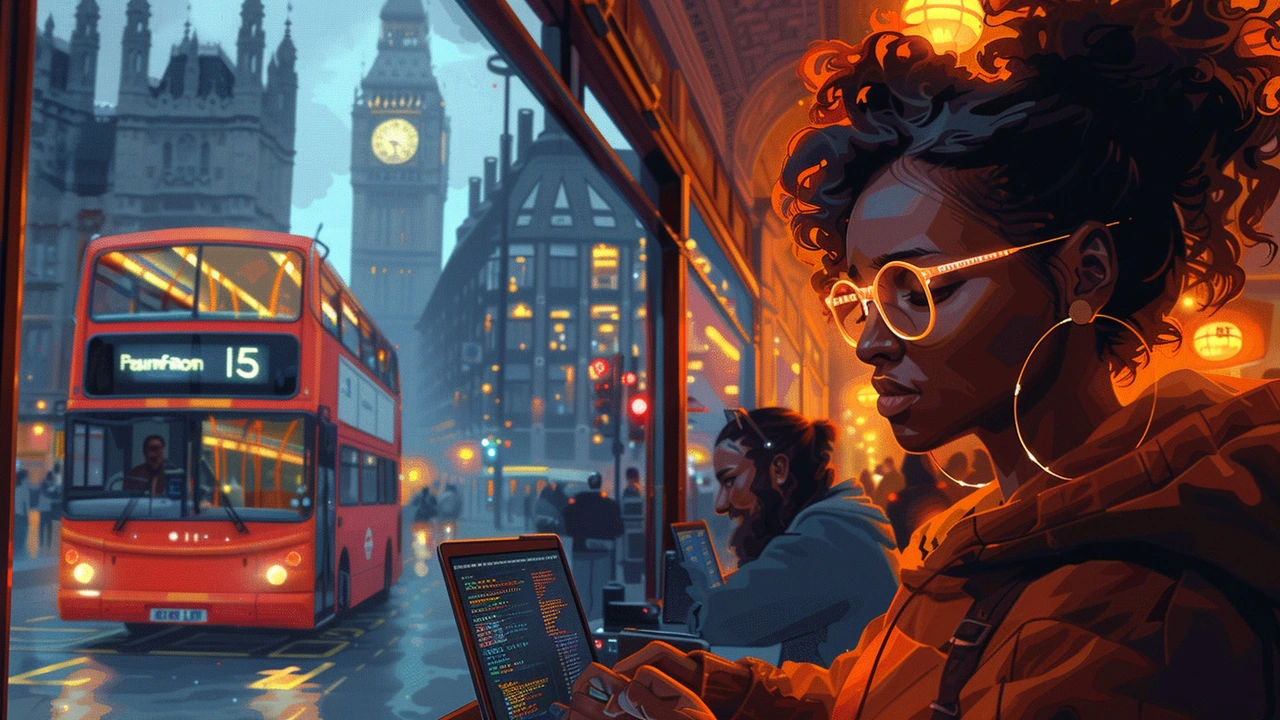
Mastering Your Tools
Being an efficient programmer is not just about knowing the syntax of a language but also about mastering the tools at your disposal. Your tools include everything from your text editor to your version control system. Knowing these tools inside out will save you a considerable amount of time and make coding a more enjoyable experience.
Let’s start with text editors and Integrated Development Environments (IDEs). These are your primary workspaces where all the magic happens. Some of the most popular ones are Visual Studio Code, Sublime Text, and IntelliJ IDEA. Each of these has different features, extensions, and shortcuts that can make a significant difference in your workflow. For instance, if you’re using Visual Studio Code, mastering its keyboard shortcuts can save you hours in the long run. Examples include Ctrl+P to quickly open a file and Ctrl+Shift+L to select all occurrences of a selected word. Learning these commands can greatly speed up your coding process.
Version control systems (VCS) like Git are another crucial tool for developers. Git allows you to keep a comprehensive history of your codebase, making it easier to track changes, collaborate with other developers, and revert to previous versions when needed. Understanding commands like git commit, git push, and git merge are essential for smooth project management. A fun fact is that Linus Torvalds, the creator of Linux, also created Git, demonstrating how influential this tool is in the programming world.
Another tool worth mentioning is your terminal or command-line interface (CLI). Mastering the CLI can significantly speed up many tasks that are tediously slow to perform via GUI. Commands like ls to list directory contents, cd to change directories, and grep to search through files can be powerful when combined effectively. Consider creating bash scripts for repetitive tasks to automate and save time.
Don't ignore the power of extensions and plugins. Most text editors and IDEs support a range of extensions that can simplify many tasks. For Visual Studio Code, the Prettier extension can format your code automatically, ensuring it remains clean and consistent. Similarly, the ESLint extension can help find and fix problems in your JavaScript code. Extensions like GitLens provide a visual overview of your Git history directly within your editor, making it easier to manage your code.
By taking the time to master your development tools, you can significantly increase your productivity and coding efficiency. As the famous developer and author Martin Fowler once said, "Any fool can write code that a computer can understand. Good programmers write code that humans can understand." And mastering your tools is a significant first step to becoming a good programmer.
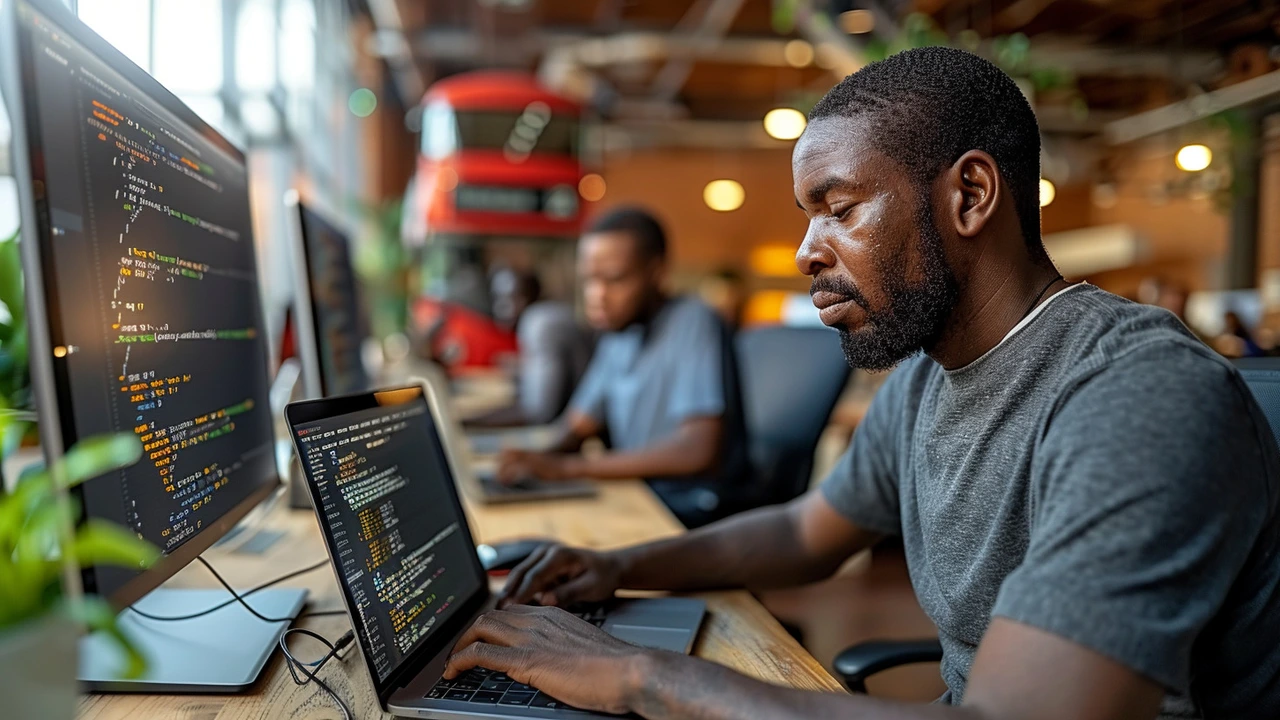
Optimizing Workflow
One of the key aspects of becoming a faster programmer is optimizing your workflow. This involves improving the way you manage your time and resources to enhance productivity. A well-optimized workflow can make complex projects feel manageable and ensure that you can write code efficiently.
To begin with, it's essential to choose the right tools for the job. Integrated Development Environments (IDEs) like Visual Studio Code or JetBrains' IntelliJ IDEA are designed to make coding easier. They come equipped with auto-completion, error checking, and a host of other features that save time. While these tools have a learning curve, investing time in mastering them pays off significantly down the line. Prioritize learning the shortcuts and custom features unique to your chosen IDE.
Next, consider setting up a version control system, such as Git. It is invaluable for managing changes in your code, especially when working in a team. Implementing good practices with Git, like writing meaningful commit messages and using branches effectively, can prevent a lot of headaches. According to a Stack Overflow survey, around 87% of developers use Git, highlighting its importance in modern programming.
Besides tools, it's crucial to establish a coding routine. Setting aside specific times of the day for focused coding can help create a rhythm. Distractions are the enemy of productivity, so turning off notifications and finding a quiet workspace can significantly boost your efficiency. The Pomodoro Technique—working for 25 minutes, then taking a 5-minute break—can also be very effective for maintaining focus and preventing burnout.
As Bill Gates famously said, "I choose a lazy person to do a hard job. Because a lazy person will find an easy way to do it." Optimizing your workflow is about finding that easy way to tackle tasks.
Automation is another vital component of an optimized workflow. Writing scripts to automate repetitive tasks can save considerable time. For instance, automating the deployment process prevents the need for manual updates and reduces the risk of errors. Tools like Jenkins or GitHub Actions can integrate seamlessly into your workflow to automate everything from testing to deployment.
Next, embracing the concept of Continuous Integration (CI) and Continuous Deployment (CD) can take your workflow to the next level. CI/CD practices help in frequently merging code changes into a shared repository, minimizing integration issues. Frequent, automated deployments ensure that the product is always in a ready-to-release state. According to a survey by CircleCI, teams practicing CI/CD deploy 208 times more frequently than those who don't.
If you are working in a team, communication and transparency are paramount. Utilize collaborative tools like Slack, JIRA, or Trello to keep everyone aligned. Regular stand-up meetings can ensure that the team stays on the same page and hurdles are addressed promptly. According to a State of Agile report, teams that communicate frequently complete projects 50% faster.
Finally, remember to constantly review and refine your workflow. Regularly assess which parts of your process are working and which aren't. Feedback from peers can provide new insights and help you make necessary adjustments. Continuously optimizing your workflow can enhance your productivity and make you a more effective programmer.
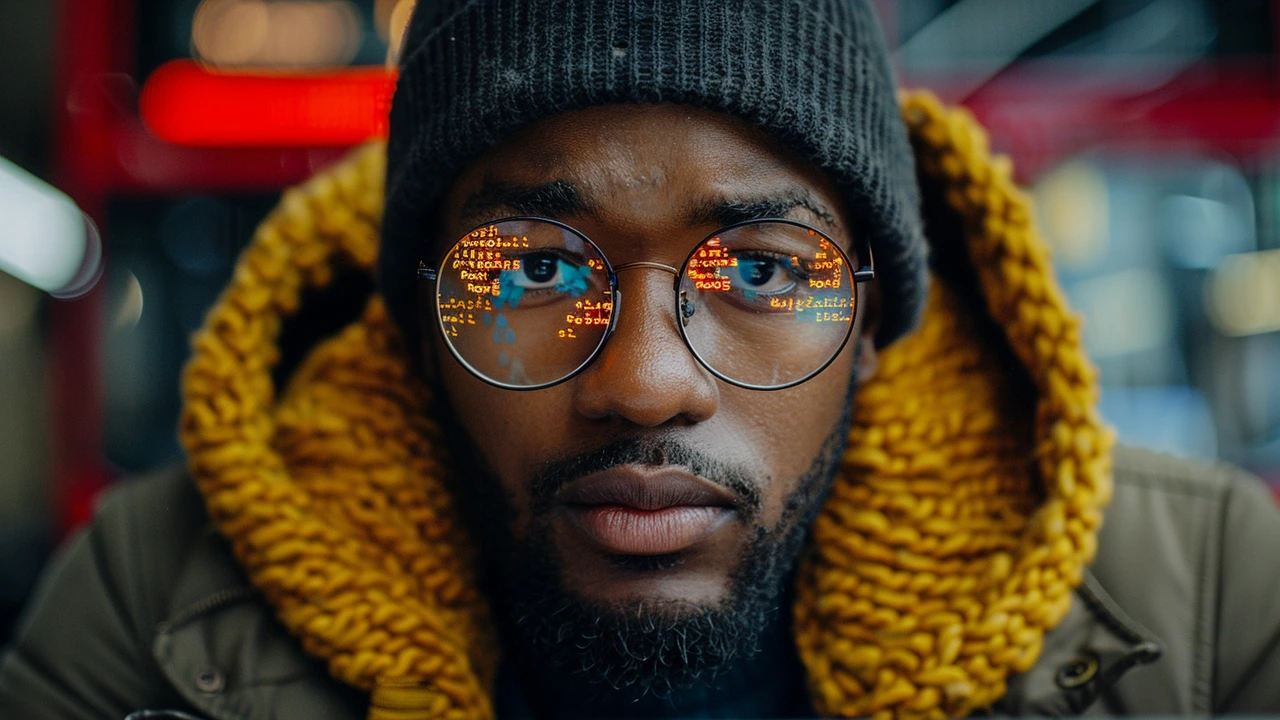
Learning and Growing
When it comes to becoming a proficient programmer, the journey never truly ends. The tech world evolves rapidly, and staying updated is essential. First off, continuous learning should be a key part of your routine. You can do this by subscribing to tech forums, reading blogs, and participating in online courses. Websites like Coursera, Udacity, and freeCodeCamp offer fantastic resources. Additionally, following influential developers on Twitter or LinkedIn can provide insights into new trends and tools.
One of the best ways to learn is by doing. Building projects, no matter how small, can help you apply what you've learned and solidify your understanding. Websites like GitHub are excellent for sharing your work and getting feedback from other developers. Open source contributions are particularly beneficial because they expose you to real-world coding practices and collaborative work environments.
Don't underestimate the power of a mentor. Having someone experienced guide you can significantly accelerate your learning curve. Whether it's a colleague, a friend, or someone from a coding bootcamp, mentors provide valuable feedback and can offer insights that books and online courses can't.
"The more I learn, the more I realize how much I don't know." - Albert Einstein
It's essential to cultivate a growth mindset. Understand that making mistakes is part of the learning process. Each bug you encounter and solve adds to your knowledge base. Celebrate those 'aha' moments as they indicate real progress. Practice problem-solving regularly. Platforms like LeetCode and HackerRank are great for honing your coding skills and preparing for technical interviews.
Networking is another crucial aspect of growth. Attend meetups, webinars, and local tech events. Networking can open doors to job opportunities and collaborations that you might not find otherwise. Communities like Stack Overflow are not only great for getting your questions answered but also for networking with industry professionals.
Finally, keep track of your progress and celebrate your milestones. Whether it's a new language you've learned, a project you've completed, or a problem you've solved, acknowledging your achievements can keep you motivated and focused on your goals. Remember, every expert was once a beginner. Your path to becoming an expert developer is paved with continuous learning and growth.