Python has taken the programming world by storm with its versatility and simplicity. But even the most experienced programmers can find new tricks to make their coding more efficient and powerful. This essential handbook is designed to share a mix of basic and advanced tips that will boost your Python programming skills.
From understanding common practices to uncovering advanced techniques, this guide has something for everyone. Whether you're just starting out or looking to refine your skills, you'll find valuable insights here to help you become a better Python programmer. Let's dive in and explore the world of Python programming together.
- Introduction to Python
- Common Python Tips
- Advanced Python Techniques
- Debugging and Optimization
- Hidden Gems in the Standard Library
Introduction to Python
Python, invented by Guido van Rossum and first released in 1991, stands as one of the most popular and versatile programming languages available today. It’s known for its simplicity and readability, which makes it an excellent choice for people new to coding and for seasoned developers alike. Python’s syntax is straightforward and mimics the English language, allowing for easier learning curves and efficient code writing. As a high-level language, it abstracts away many of the complex details of the computer’s hardware, letting programmers focus on solving problems rather than getting bogged down by intricate technical details.
Python is used in a wide array of applications, from web development and data analysis to artificial intelligence and scientific computing. Its rich ecosystem, full of libraries like NumPy for numerical operations, pandas for data manipulation, and TensorFlow for machine learning, empowers developers to tackle various challenges efficiently. The Python Package Index (PyPI) offers a treasure trove of additional modules and packages to extend Python’s functionality even further.
The strong community support and comprehensive documentation also contribute significantly to Python’s popularity. Whether you’re troubleshooting an error or looking for guidance on implementing a new feature, it’s easy to find answers thanks to the active community forums and expansive resources available online. This spirit of collaboration lends itself to continuous improvements and innovations within the language.
“Python's simple, easy to learn syntax emphasizes readability and therefore reduces the cost of program maintenance” — Python Software Foundation.
Another notable feature of Python is its cross-platform compatibility. Code written in Python can run seamlessly on various operating systems, such as Windows, macOS, and Linux, without requiring modification. This makes Python incredibly versatile and valuable for developers working in diverse environments. Additionally, the language’s interpreted nature means that you can run Python code as soon as it's written, enabling rapid testing and debugging.
While Python is lauded for its simplicity, it’s also powerful enough to handle complex tasks. The language supports multiple programming paradigms, including procedural, object-oriented, and functional programming. This versatility means that you can adapt Python to suit the needs of virtually any project, whether you’re building a quick script or developing a full-scale application.
Year | Python Version |
---|---|
1991 | Python 0.9.0 |
2000 | Python 2.0 |
2008 | Python 3.0 |
It’s worth noting that Python excels not only in its technical capabilities but also in its philosophy. The language's development is guided by the principles laid out in PEP 20, known as the Zen of Python. This philosophy emphasizes simplicity, readability, and the importance of community, which helps ensure that Python remains easy to use and collaborative throughout its evolution.
Common Python Tips
Python is loved by many for its simplicity, readability, and the vast ecosystem of libraries and frameworks that make development easier and more efficient. However, there are some common tips and tricks that can significantly enhance the way you write and understand Python code. For instance, using list comprehensions is a go-to method for creating new lists based on existing ones. It's a more concise and readable way compared to using traditional for-loops. List comprehensions can be more efficient and less prone to errors.
Another useful tip is to take advantage of the Python built-in functions. Functions like len(), sum(), min(),
and max()
can simplify your code. By using these built-in options, not only do you make your code cleaner, but also you tap into the optimized implementations provided by Python. This can lead to more efficient execution. Keeping your code clean and using the DRY (Don't Repeat Yourself) principle is another aspect every programmer should follow. Avoiding redundancy in your codebase makes it easier to maintain and understand.
Understanding the importance of exception handling is another critical aspect of Python programming. Using try
and except
blocks allows your program to handle unexpected errors gracefully. Rather than letting your application crash, you can manage exceptions and provide meaningful feedback to the users. Additionally, it's essential to understand the differences between shallow and deep copies. This knowledge helps avoid unintended side effects when modifying objects in your code.
Another invaluable tip is leveraging Python's powerful standard libraries. Libraries like itertools
for efficient looping, collections
for specialized container data types, and functools
for higher-order functions can dramatically simplify various tasks. As Alex Martelli, a prominent Pythonista, once said:
"To describe something as clever is not considered a compliment in the Python culture."
This quote highlights the Python philosophy of simplicity and readability over unnecessary complexity.
Consistent naming conventions are also essential. Adhering to PEP 8 guidelines ensures that your code is readable and standardized, making collaboration with other developers smoother. Choosing descriptive names for your variables and functions further enhances code readability. Lastly, using virtual environments when managing dependencies in your projects can save you a lot of trouble. This isolates your project’s dependencies, preventing conflicts between different projects using different versions of the same package.
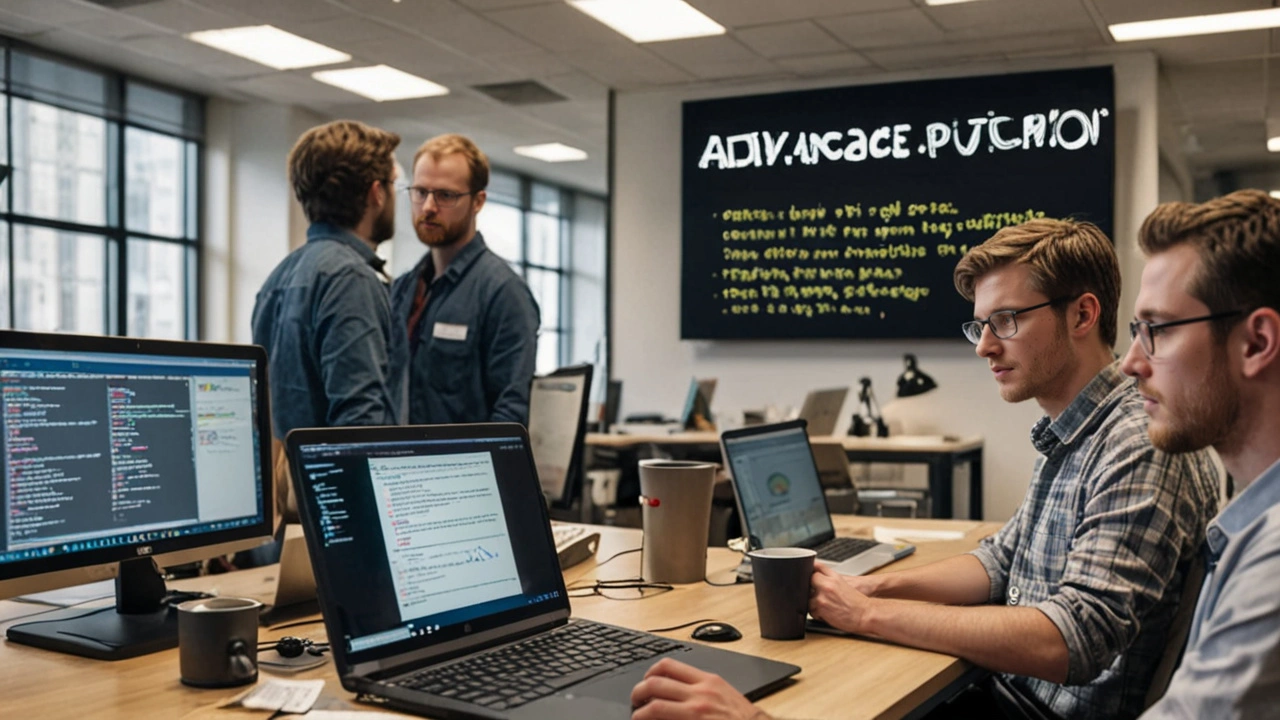
Advanced Python Techniques
As you grow more comfortable with Python programming, you'll want to explore advanced techniques that can help you streamline your workflow and craft more efficient code. One of the first steps in advancing your Python skills is understanding the power of list comprehensions. These allow you to create new lists by applying an expression to each item in an existing list, with an optional condition. Instead of creating an empty list and using a for loop to append items, you can accomplish the same task in a single, concise line.
Another crucial technique is mastering decorators. Decorators are a powerful tool that allows you to modify the behavior of a function or class method. A decorator is a function that takes another function and extends its behavior without explicitly modifying it. This can be particularly useful for logging, enforcing access control, or memoization. By understanding and utilizing decorators, you can keep your code DRY (Don't Repeat Yourself) and maintain a clean, readable structure.
Generators are another advanced feature you should familiarize yourself with. They allow you to iterate through data without loading the entire dataset into memory, which can be a game-changer when working with large datasets. Generators are created using functions with the yield statement. Each time the generator's __next__() method is called, the function resumes where it left off, producing the next value in the sequence. This makes them highly efficient for scenarios where lazy evaluation is beneficial.
Utilizing the context manager is an advanced trick that can save you from many headaches, especially when handling resources like file streams. A context manager enables you to allocate and release resources precisely when you need to. This is managed using the with statement in Python, which ensures that resources are cleaned up promptly after their use. It's particularly useful for managing memory and other resources efficiently.
Metaprogramming
Metaprogramming is another powerful tool in the advanced Python programmer’s toolkit. This technique involves writing programs that can manipulate other programs or themselves at runtime. In Python, you can leverage functions like getattr(), setattr(), hasattr() and delattr() to modify attributes dynamically. You can also use the type() function to create new classes dynamically. This offers a high degree of flexibility and can enable you to write more abstract and reusable code.
“Metaprogramming is a must-learn for any Python developer looking to leverage the language to its fullest potential,” says Guido van Rossum, the creator of Python.
Concurrency and Parallelism
Concurrency and parallelism are topics that any advanced Python developer should master. Python offers several libraries and frameworks, such as threading, multiprocessing, and asyncio, to handle these tasks. Concurrency entails executing multiple tasks concurrently, while parallelism involves executing multiple tasks simultaneously. Understanding the difference and knowing when to use each is crucial for writing efficient and responsive applications. The asyncio library is particularly useful for writing asynchronous programs and can greatly enhance performance, especially in I/O-bound applications.
Finally, understanding the intricacies of Python’s memory management, including garbage collection and memory allocation, can help you write more efficient code. Python’s memory manager handles allocation and deallocation of memory automatically, but knowing how it works can help you optimize your programs to run faster and use less memory. By tracking reference counts and clearing unused objects, you can manage memory more effectively, avoiding potential memory leaks and sluggish performance.
Debugging and Optimization
Debugging and optimization are crucial components of programming. When you're dealing with complex Python code, it's easy to overlook minor mistakes that can lead to bigger issues down the line. Debugging helps identify and fix these errors early, saving time and effort. Python provides several built-in tools to make this process more efficient, such as the pdb module.
The pdb (Python Debugger) module is a powerful tool for letting you inspect code execution step-by-step. Imagine you've written a piece of code and it doesn’t seem to work as expected. With pdb, you can set breakpoints, step through each line, and view the values of variables at any given moment. This granular control makes it easier to identify where things go wrong and how to correct them.
While debugging is essential for identifying errors, code optimization helps improve the performance of your program. Optimizing Python code can make it run faster and use less memory. One straightforward way to optimize is by using list comprehensions instead of standard loops. For instance, consider a scenario where you need to create a new list based on an existing one. Traditional loops can be slow, but a list comprehension can achieve the same result in a more efficient and concise manner.
Another effective optimization technique is leveraging the built-in functions that Python offers. Functions like map, reduce, and filter can help process data faster compared to manually looping through elements. These functions are implemented in C, making them significantly faster than their Python-loop counterparts. According to Python's official documentation, using built-in functions can speed up operations by up to 20%, depending on the task.
Understanding memory management is also vital for optimizing Python code. The gc (garbage collector) module helps manage the memory usage automatically, but sometimes manual intervention is required. Using tools like objgraph can help you visualize memory usage and identify memory leaks. Memory leaks can lead to significant slowdowns, especially in long-running applications.
Profiling your code is another important part of the optimization process. Profiling helps identify which parts of your code are consuming the most resources. The cProfile module in Python is useful for this purpose. By running your script with cProfile, you can get a detailed report showing how much time each function takes. This information can be crucial for pinpointing bottlenecks in your code.
Here are some practical tips for debugging and optimization:
- Set clear and intentional breakpoints in your code.
- Utilize list comprehensions for cleaner and faster loops.
- Leverage Python's built-in functions where possible.
- Use the gc module to handle garbage collection efficiently.
- Profile your code with cProfile to identify bottlenecks.
Additionally, keeping your codebase clean and well-documented can make the debugging and optimization process much easier. Clean code is easier to read, understand, and modify, which is particularly important when working in a team environment. Well-documented code gives insights into the logic behind different sections, making it easier to identify potential issues.
"Code is like humor. When you have to explain it, it’s bad." – Cory House
Combining these debugging and optimization strategies can significantly improve your Python programming efficiency and performance, making you a more effective developer.
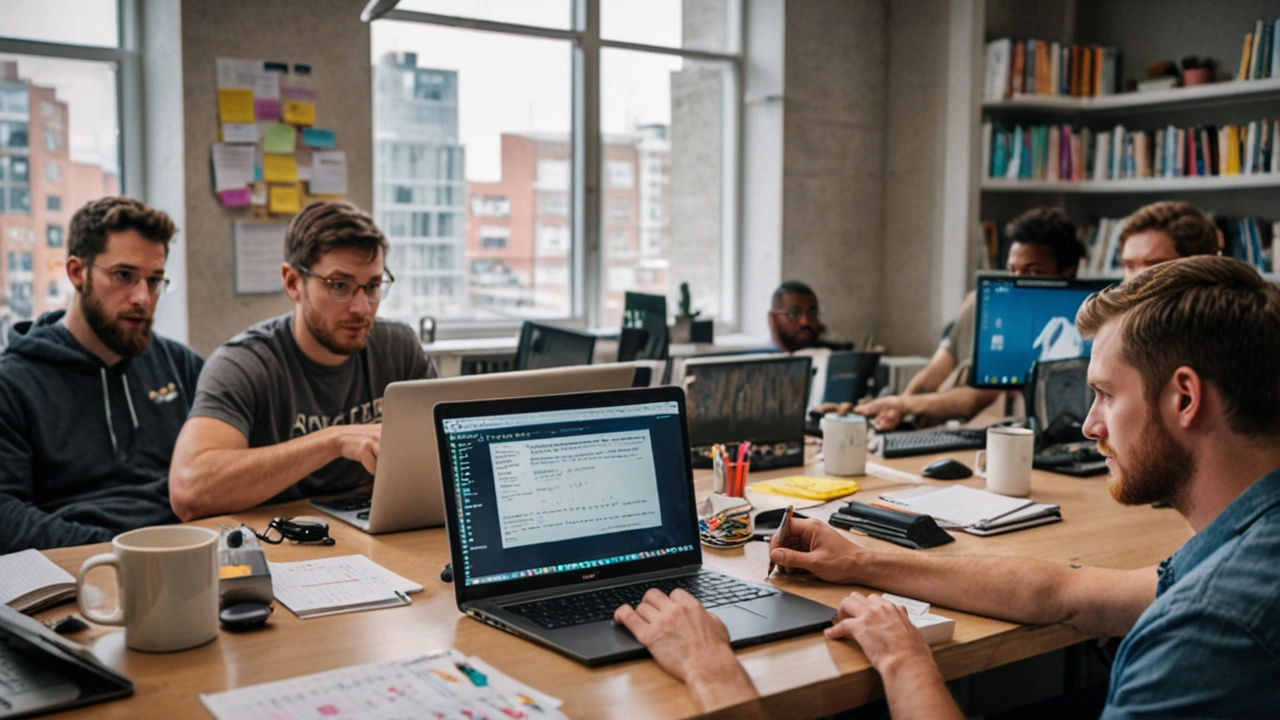
Hidden Gems in the Standard Library
The Python Standard Library is like a treasure chest waiting to be unlocked. There are many useful modules that might be overlooked but can significantly improve the efficiency and quality of your code. Exploring these hidden gems can provide surprising solutions to common problems, and sometimes break new ground in what you can achieve with Python.
One often overlooked module is itertools, which is perfect for handling iterators. It provides a collection of tools for creating iterators for efficient looping. With functions like product
, permutations
, and combinations
, it allows you to solve complex mathematical problems with ease. For example, using itertools.combinations
, you can generate all possible groups or combinations of a list without having to write complex nested loops.
Another gem is the collections module, which provides alternative container datatypes. A few notables include defaultdict
, OrderedDict
, Counter
, and deque
. These can significantly simplify tasks that would otherwise require more verbose code. For instance, defaultdict
can automatically initialize dictionary values, which can save you the hassle of checking for the existence of a key.
The functools module is extremely useful for higher-order functions and operations on callable objects. The lru_cache
decorator within this module can be a real game-changer for optimizing your functions. By caching the results of expensive function calls, it can shorten execution times considerably. This is extremely useful for recursive algorithms or functions that process large amounts of data or perform complex calculations.
Python’s pathlib is another powerful module that often goes under the radar. It offers a simple and unified way to deal with filesystem paths. While the os
module can handle file paths, pathlib
provides a more readable and object-oriented approach. For example, it allows you to perform straightforward manipulations like renaming files or iterating over directory contents with minimal code.
“The standard library has a richer set of useful tools than many developers realize. Mastering these modules can enhance your productivity and broaden your coding horizons.” - Guido van Rossum, Creator of Python
Don't forget about the datetime module, which is indispensable for anything related to date and time. Whether you are formatting dates, calculating time differences, or scheduling events, datetime
has all the tools you need. From understanding time zones to generating timestamps, it can prevent a lot of the headaches associated with date manipulation.
Lastly, consider the contextlib module, specifically the contextmanager
decorator. This might change how you handle resources like file streams or network connections. By making it easier to create context managers, contextlib
can help ensure that resources are properly managed, thus preventing potential issues such as file corruption or memory leaks.
Exploring and mastering these modules can make a significant difference in your daily coding tasks. The Python Standard Library is comprehensive and versatile, often making third-party libraries unnecessary for many tasks. So next time you face a challenging problem, it might be worth checking if there’s already a solution hidden within the standard library.