Diving into the world of PHP can be both exciting and overwhelming for many developers. It's a language that powers a large portion of the web, yet mastering it requires more than just understanding syntax. The capacity to simplify your PHP code not only enhances functionality but also saves valuable time and resources.
In this article, we're going to explore several essential tricks that can help you streamline your projects. From using built-in PHP functions to fully understanding error handling, these tips are tailored to make your coding journey smoother. Whether you're a beginner or a seasoned pro, you'll find valuable insights that will take your PHP skills to the next level.
- Understanding PHP Basics
- Leveraging Built-In Functions
- Embracing Object-Oriented Programming
- Optimize for Performance
- Error Handling and Debugging
- Advanced Techniques for Experienced Coders
Understanding PHP Basics
PHP, which stands for Hypertext Preprocessor, has become a cornerstone language for web development. Since its inception in 1994 by Rasmus Lerdorf, PHP has evolved dramatically, expanding its capabilities and optimizing performance. Today, it is a server-side scripting language used to manage dynamic content, databases, session tracking, and even build entire e-commerce sites. Supporting a vast community of developers and a robust suite of tools, PHP continues to be a reliable choice for web development projects.
To begin mastering PHP, start by setting up a local development environment. This typically involves installing a web server like Apache or Nginx, a database system such as MySQL, and PHP itself. Many developers opt for packages like XAMPP or WAMP to streamline this process. Writing your first PHP script is a rite of passage—one you can execute within an HTML document using the PHP opening and closing tags. The syntax is straightforward, and its flexibility allows HTML code to be seamlessly interlaced with PHP functionalities, meaning you can embed PHP directly within a webpage.
Diving deeper into PHP requires understanding its data types. PHP supports several types, including integers, floats, booleans, strings, arrays, objects, NULL, and resource types. This versatility enhances PHP’s ability to handle a wide range of scenarios. Each data type serves specific functions; understanding how to manipulate them is key to effective programming. Interestingly, PHP automatically converts the variable data type depending on its value, demonstrating a dynamic behavior that can be both a boon and a bane for developers. It's strategic to use type coercion thoughtfully to avoid unexpected results.
"At its core, PHP is a reflection of both flexibility and simplicity in web programming," says Zeev Suraski, a key figure in PHP development. "Its design principles are what make it adaptable to various web requirements."
Writing efficient PHP code also relies on understanding its superglobal variables. These are predefined variables in PHP that start with a dollar sign and underscore (e.g., $_GET
, $_POST
, $_SESSION
). They enable access to script data regardless of the scope, effectively handling form submissions, session management, and feedback data management. Using them wisely can lead to a robust, interactive PHP application that responds well to user inputs.
PHP tricks also include the proper use of functions, loops, and conditionals, which form the foundation of any programmatic logic. Loop structures like for
, foreach
, while
, and do-while
facilitate repetitive tasks without redundancy, whereas conditional statements (if
, else
, switch
) guide the flow of logic based on specific conditions. Functions are pivotal, allowing the breakdown of code into reusable components that enhance clarity and upkeep. They can range from simple user-defined constructs to complex procedural expressions, which can succinctly manage intricate operations.
Popular PHP Development Tools
Being a popular choice for developers, PHP boasts a wide array of tools to aid in efficient development. From coding editors like Visual Studio Code and PHPStorm to debugging and profiling tools such as Xdebug, these resources significantly improve productivity. To put things into perspective, consider how Visual Studio Code provides an integrated terminal, Git control, and a vibrant extensions marketplace—all under one neat interface, making it favored among developers worldwide. Another gem, Composer, is a dependency manager essential for managing libraries and packages crucial to PHP-friendly projects. Mastering these tools can significantly ramp up your PHP development expertise, buffering speed and finesse to your workflow.
Leveraging Built-In Functions
When diving into the depths of PHP development, one of the most effective ways to enhance your coding efficiency is to make the most of built-in functions. PHP boasts a robust library of over one thousand built-in functions, each designed to handle a variety of specific tasks. These functions can save you both time and frustration by simplifying complex operations into more manageable lines of code. Practically speaking, rather than reinventing the wheel with custom functions, a PHP developer can easily streamline code with these pre-built options.
Take, for instance, something as common as string manipulation. Developers often need to find, replace, or alter strings in some way, and that's where PHP’s built-in functions like strlen(), strpos(), or str_replace() come into play. These functions are optimized for performance and can handle string operations in a fraction of the time it might take if you were to code them manually. By leveraging these, developers improve not only their code's efficiency but also its readability, making collaboration with others much smoother.
Moreover, when dealing with arrays, PHP offers functions that can effortlessly sort, combine, or transform them. The use of functions like array_merge(), array_reverse(), or array_walk() effectively simplifies what would otherwise be a set of complex loop operations. Utilizing these functions is about understanding the full scope of what's available, and keeping abreast of updates as PHP evolves. For instance, PHP 7 introduced more enhancements and functions, giving developers an even broader arsenal of tools.
According to Rasmus Lerdorf, the creator of PHP, in an interview, he emphasized, "PHP is about thinking in ready-made solutions. It's written to make the most common web tasks simple, fast, and efficient."
When handling database operations, built-in PHP data objects (PDO) take care of a variety of database interactions. These methods offer a layer of security with prepared statements that protect against SQL injection attacks, crucial for applications expecting user input. This adds both performance and security, providing solutions that are both eloquent and effective. PHP functions are indeed a treasure trove for every developer.
Lastly, it's crucial to remember that PHP’s functions are frequently updated with new versions of the language. Staying current with these updates not only keeps your project optimized but also enhances its security protocols. Understanding that using built-in functions is more about staying informed than just writing code can transform incredible potential into tangible results. Thus, mastery of these functions is an essential skill in web development, turning mere lines of code into seamless, efficient solutions.
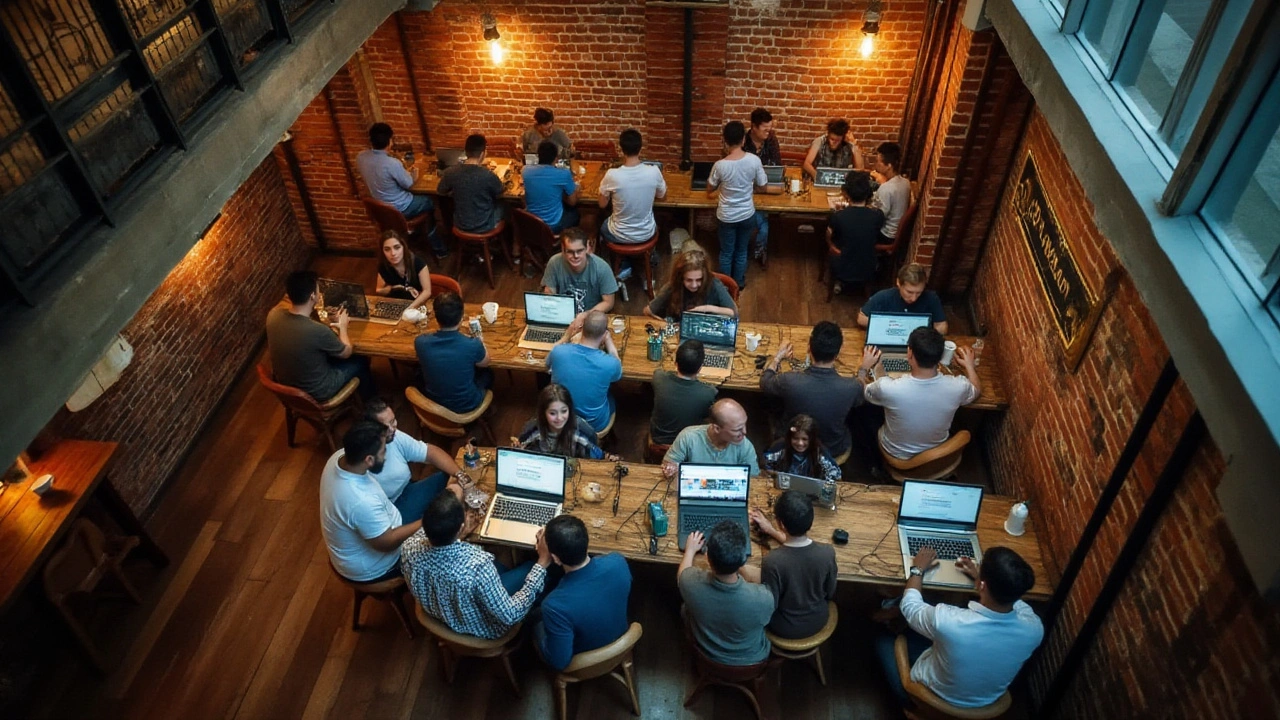
Embracing Object-Oriented Programming
Object-Oriented Programming (OOP) in PHP is more than just a paradigm; it's a way to bring clarity and structure to your projects. At its core, OOP allows developers to model concepts as objects, providing an intuitive way to organize code. This makes collaboration and maintenance significantly more manageable, as code is modular and isolated in classes. The PHP language, starting with its 5.0 version in 2004, fully embraced OOP principles, offering programmers the ability to create scalable, reusable code. By encapsulating data and behaviors into classes, developers can craft flexible applications that are more responsive to changes and easier to debug.
One of the key components of OOP is the concept of inheritance. This allows you to create a new class that is based on an existing class, saving time and minimizing redundancy. For instance, if you have a class that describes a vehicle, you can create a specific class for a car that inherits properties and functions from the vehicle class. Using this technique in web development, when you'll need to adjust functionalities like adding features or changing behaviors, you only have to do so in one place. Moreover, OOP encourages the use of interfaces and abstract classes, leading to a more rigid though flexible structure, promoting a higher level of consistency throughout your codebase.
A prominent advocate for OOP, Martin Fowler, in his book ‘Refactoring,’ said,
"Any fool can write code that a computer can understand. Good programmers write code that humans can understand."This highlights the shift Object-Oriented Programming brings from machine-level efficiency to human-friendly accessibility. PHP's support for OOP is built on established principles like polymorphism, which allows objects to be treated as instances of their parent class, paving the way for more generalized and dynamic code functionality. This dynamism is particularly crucial in writing efficient code that is both adaptive and resilient, traits synonymous with high-quality programming.
Moreover, when considering coding tips, using OOP often leads to better resource management. PHP's memory is handled more efficiently through mechanisms like destructors, which are automatically invoked to free resources once an object is no longer needed. The memory optimization becomes apparent in large-scale applications where performance can quickly turn from adequate to inadequate. In addition, automated testing frameworks like PHPUnit that are designed to work seamlessly with object-oriented programming, facilitate rigorous testing environments, ensuring the integrity and reliability of the code. The art of creating tests becomes less about verifying isolated functions and more about testing interactions between objects, granting a holistic approach to software quality assurance.
In summary, as developers delve deeper into PHP, embracing the principles of Object-Oriented Programming is not just an option but a necessity in crafting modern, efficient applications. The robustness and scalability that OOP offers are unmatched. It encourages best practices, such as DRY (Don't Repeat Yourself) and SOLID principles, driving developers towards creating sustainable and maintainable codebases. The transition from procedural to object-oriented programming is aided by numerous resources and community support available in the PHP web development sphere, ensuring that learning curves are short and rewarding. Adopting OOP in PHP can significantly transform coding habits, catalyzing a shift towards cleaner and more human-friendly code alignment.
Optimize for Performance
When it comes to web development, finding ways to optimize for performance in PHP is critical. This can greatly improve user experience and reduce server load. The journey towards faster PHP applications starts with understanding the importance of writing efficient code. First, remember that PHP scripts are compiled into bytecode, which is then interpreted by the Zend engine. Reducing the number of operations this engine performs is key. Start by revisiting every loop in your code; ensure that they're doing precisely what they need to without any extra calls. It's important to remember that each function or method call in PHP introduces additional overhead, so look for opportunities where caching or memoization might save some of this repeated work.
One significant aspect of optimization is database interaction. Whenever you're dealing with databases, using prepared statements not only enhances security but also can increase performance. This is because prepared statements allow the database to compile and optimize queries beforehand, which can be reused multiple times with different inputs. Another vital tip is to avoid querying the database inside a loop — it's much more efficient to retrieve all needed data in one go using a singular query. If you're dealing with large datasets, considering pagination is a great strategy. By limiting the number of results returned to the user, you ensure that your application remains swift and responsive.
Moreover, PHP's built-in Opcache is an underutilized gem. By storing precompiled script bytecode in memory, Opcache reduces the overhead of parsing and compiling PHP files every request. Activating Opcache is straightforward and has an immediate impact on performance. For those who use frameworks, understanding how they dispatch, route, and handle requests can be beneficial. Performance often depends on how efficiently you structure these processes. Minimizing autoloading and ensuring unnecessary components aren't loaded can result in substantially faster runtimes.
"Premature optimization is the root of all evil." - Donald Knuth. This quote reminds developers to focus on clear, functional code first. Once this is achieved, performance tweaks can follow.
Optimization isn’t only about code; it's about choices across the stack. The environment in which your PHP runs is just as crucial. Using lightweight servers like Nginx over Apache can provide speed benefits in many cases. PHP versions also make a difference; always aim to use the latest stable release, as improvements and bug fixes continually evolve PHP’s performance capabilities. Monitoring tools like New Relic or built-in PHP functions can help keep an eye on bottlenecks. By watching real-world usage data, you can make informed decisions about where to apply optimizations.
Coding tips in PHP should not just be about shortcuts and tricks. It's about understanding the language's inner workings and applying best practices consistently. Remember though, no optimization is truly beneficial if it makes the codebase harder to maintain or understand. Balance is key. Efficient code is sustainable only when it remains accessible to future developers, including your future self. By focusing on clear logic and optimizing where it really counts, you ensure that your applications don't just work – they thrive under any load.
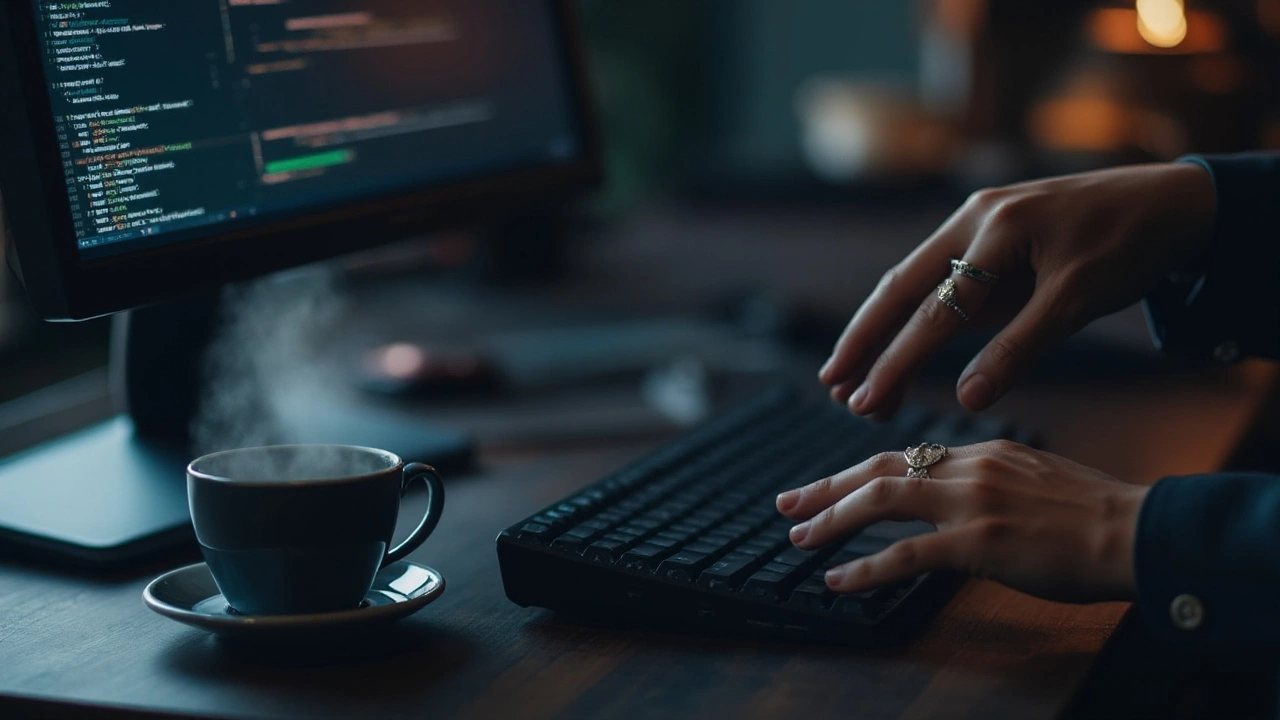
Error Handling and Debugging
Every developer, regardless of seniority, encounters errors in their code. It's a natural part of the development process, especially when working with PHP, a language that many developers rely on for building dynamic web applications. To effectively manage these errors, it's crucial to understand PHP's error handling capabilities. The language provides a robust error reporting mechanism that can be customized to suit the needs of your project. This includes using the error_reporting() function to control which errors are reported and tailoring the display_errors directive to determine whether errors should be presented in the output or logged for later review. Mastering this level of control can significantly reduce development time by allowing you to concentrate on resolving the root cause of issues without distraction from less critical warnings.
Debugging is equally important and often perceived as an art form by veteran developers. A solid approach to debugging in PHP often begins with leveraging built-in functions like var_dump() and print_r() to inspect variables and data structures in real-time. These tools provide invaluable insights into what your code is doing at specific points in execution, making it easier to pinpoint errors. To enhance this process, many developers advocate for using a dedicated debugging tool, such as Xdebug. This powerful extension integrates seamlessly with many IDEs, providing step-by-step debugging capabilities that allow you to meticulously track the flow of your program and examine the state of your variables at each step.
"Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it." – Brian Kernighan
In addition to leveraging tools and functions, establishing a well-structured error logging practice is crucial. A structured log helps to identify patterns and recurring issues that may indicate deeper problems in the codebase. Depending on the scale of your application, implementing a logging library such as Monolog can greatly enhance the efficiency and readability of your logs, allowing developers to quickly diagnose issues and initiate resolution processes.
Modern development environments also offer automated solutions for error reporting and logging. Services like Sentry and Rollbar provide real-time error tracking and reporting, often with insights that are difficult to gather manually. These tools can automatically capture and analyze the errors that occur in your application, offering detailed reports that help you get to the heart of an issue without delay. Integrating such services can give your team a significant advantage by minimizing the time spent on error tracking and allowing more focus on write quality, impactful code.
Finally, the importance of thorough testing cannot be overstated. Incorporating unit tests and integration tests within your PHP projects can prevent many errors from ever reaching production. PHPUnit is a popular choice among PHP developers, providing a framework to automate testing and ensure code behaves as expected. By including these tests as part of your development cycle, you not only mitigate the occurrence of errors but also establish a level of confidence in your code’s functionality and reliability. This proactive approach in error handling saves time and resources, paving the way for more efficient and effective web development.
Advanced Techniques for Experienced Coders
For coders who have moved beyond the basics and are ready to tackle more advanced PHP techniques, the opportunities to optimize and refine code are vast. Leveraging object-oriented programming (OOP) is one of the pathways that significantly enhance code structure and reusability. Embracing OOP entails understanding and applying principles like inheritance, encapsulation, and polymorphism. These concepts allow for creating more scalable and maintainable applications by organizing code into classes and objects, which model real-world interactions.
An important aspect of efficiency in PHP is understanding and utilizing design patterns. These are proven solutions to common problems in software design. Familiarity with patterns like MVC (Model-View-Controller), Singleton, and Factory can drastically enhance your coding architecture. The Singleton pattern, for instance, ensures a class has only one instance while providing a global point of access. Meanwhile, the Factory pattern aids in creating objects without specifying the exact class of object that will be instantiated, ensuring flexibility and dynamism in code.
Martin Fowler, a renowned software engineer, once said, "Design patterns help you build better software systems." Recognizing these patterns and implementing them effectively can be a game-changer for developers looking to optimize their PHP applications.
Optimizing Query Performance
Experienced developers understand that unsophisticated database interactions can be bottlenecks for application performance. Optimizing SQL queries is therefore crucial. Using joins effectively, indexing tables, and structuring complex queries cautiously can cut down redundant data fetching and boost the application's speed. Moreover, understanding and applying PHP's PDO (PHP Data Objects) provides an additional layer of flexibility and security in managing databases. PDO allows the use of prepare statements that both reduce parsing times and safeguard against SQL injections. Combining these techniques makes your database connections both faster and safer.
Enhanced Security Practices
Security is paramount, and only increasingly becomes so as your application scales. Incorporating security best practices in your PHP code is non-negotiable. Input validation, for example, is fundamental in protecting against attacks such as cross-site scripting (XSS) and SQL injection. By using functions like filter_var() or custom validation functions, you can ensure that user inputs are sanitized properly. Additionally, managing session data securely is another crucial aspect. Using HTTPS ensures that data transmitted between client and server remains encrypted. Moreover, employing more secure password hashing algorithms, such as Bcrypt or Argon2, for storing user credentials can prevent unauthorized access.
Asynchronous PHP
Another fascinating area for experienced PHP developers is asynchronous programming. Traditionally, PHP is synchronous, meaning each line of code is executed sequentially. However, using libraries like ReactPHP allows developers to break free from this limitation. Asynchronous PHP can handle multiple requests simultaneously resulting in increased application performance, especially under heavy load conditions. This technique is particularly beneficial for applications requiring real-time data processing, such as chat applications or live notifications.
The flexibility and power of PHP are limitless when wielded by skilled hands. By integrating these advanced techniques, experienced coders can develop applications that are not just functional, but robust, efficient, and secure. Through constant learning and adaptation, one can unlock the full potential of PHP programming, crafting software that meets modern demands with precision and creativity.