So, you're curious about stepping into the world of AI with Python, right? Great choice! Python is like the Swiss Army knife of programming languages, especially when it comes to AI. Why? Well, it's super easy to learn and has a ton of libraries like TensorFlow and PyTorch on its back. These libraries help you build, train, and deploy neural networks without breaking a sweat.
But before you jump into coding, let’s talk about setting up your environment. You'll need Python installed on your computer. Head to Python's official site, download the latest version, and voila—you're on your way! Don't forget to grab an Integrated Development Environment (IDE) like PyCharm or Jupyter Notebook. They’re going to be your best buddies as you work through your AI projects.
- Why Python for AI?
- Setting Up Your Python Environment
- Basic AI Concepts with Python
- Key Python Libraries for AI
- Practical Tips for Beginners
Why Python for AI?
When it comes to AI, there's Python—and then there's everything else. Curious why it's so popular? Let’s break it down. For starters, Python is famous for its simplicity and readability. You don’t need to be a coding wizard to get started, which is super appealing to beginners and experts alike.
But what really sets Python apart for AI is its ecosystem. You've got this incredible stack of libraries and frameworks, like TensorFlow and PyTorch, that make developing AI models a breeze. These tools handle the complicated math behind AI, so you can focus on building cool things.
Community Support
Then there’s the community. Python has a massive group of users and developers who consistently contribute to its growth. Need help with a bug or looking for a new feature? Chances are someone’s already got you covered on forums like Stack Overflow or GitHub. This network of support is gold when you’re diving into AI.
Integration Friendly
Also, Python plays well with others. It can easily integrate with other languages like C/C++ and Java, which is great if you need to extend your applications or add AI capabilities to existing projects. Plus, Python supports both structured data (think tables) and unstructured data (like text), which is crucial when you're dealing with varied AI applications.
Language | Usage in AI Projects (%) |
---|---|
Python | 57% |
R | 12% |
Java | 7% |
Finally, many popular tech companies like Google and Facebook use Python for their AI projects. Why does that matter for you? Well, it means there's a ton of real-world examples and resources you can draw from. If the big dogs trust Python for their AI, that’s a pretty solid endorsement.
Setting Up Your Python Environment
Getting ready to dive into Python for AI? It all starts with setting up your environment, and trust me, it's not as daunting as it sounds. Let's break it down.
Step 1: Installing Python
Start by downloading the latest version of Python from its official website. Choose the version that suits your operating system. The installation process is straightforward—just follow the prompts like you're installing any other software.
Step 2: Choosing Your IDE
An Integrated Development Environment (IDE) makes writing and testing code much easier. For beginners, I recommend PyCharm or Jupyter Notebook. PyCharm offers a great balance of power and usability. On the other hand, Jupyter Notebook is perfect if you prefer an interactive approach to coding, especially handy for data science and AI tasks.
Step 3: Setting Up Virtual Environments
Ever screwed up your system because of different package versions? That's where virtual environments come to rescue. Use venv
, a module included with Python, to create isolated environments. Here's how you set it up:
- Open your terminal or command prompt.
- Navigate to your project directory.
- Run
python -m venv myenv
. Just replace 'myenv' with your preferred environment name.
Activate your environment by running myenv\Scripts\activate
on Windows or source myenv/bin/activate
on Mac/Linux. Now install your packages without affecting the rest of your system!
Step 4: Installing Essential Libraries
Next, you'll need some libraries that are commonly used in AI. Use pip
to install them:
- NumPy
- Pandas
- Torch
- TensorFlow
Just run pip install numpy pandas torch tensorflow
to get all at once.
And there you have it! Your Python environment is all set for you to start coding away.
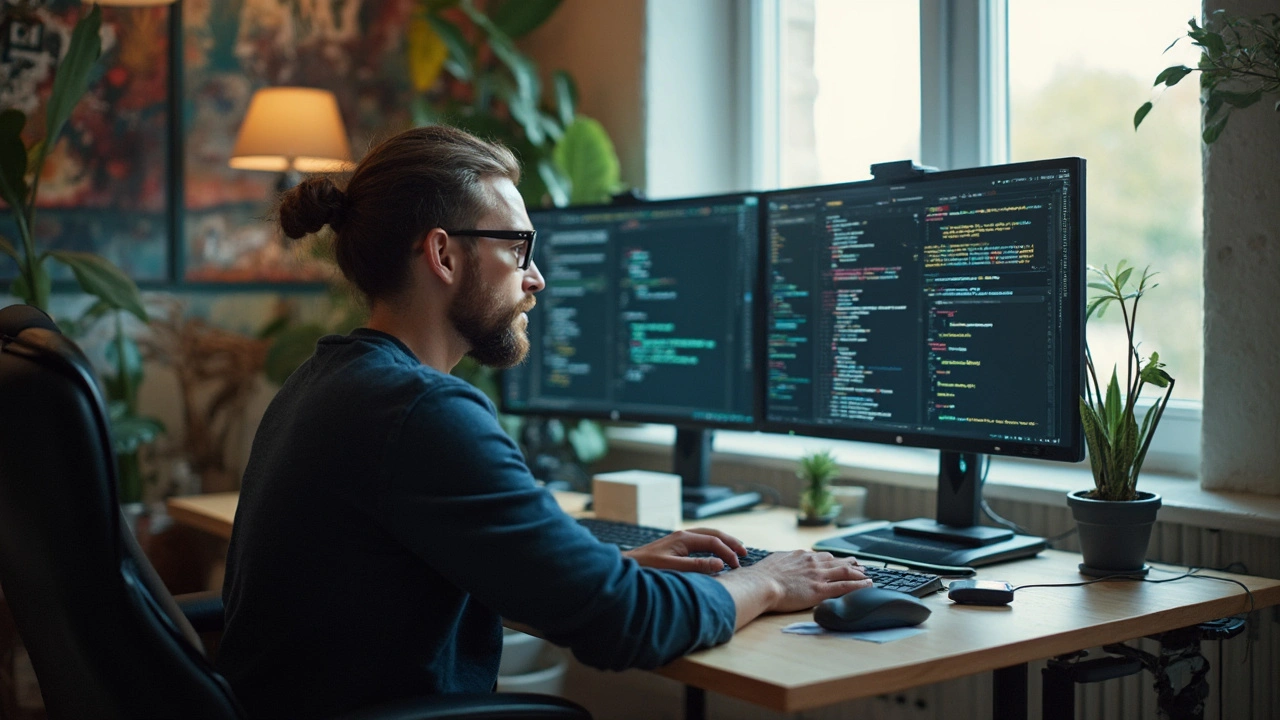
Basic AI Concepts with Python
Alright, so you're ready to dig into some basic AI concepts with Python? Awesome! Python makes it super accessible to understand and implement fundamental AI ideas. Let's break down some basic concepts you’ll often encounter in your AI journey.
Understanding Algorithms
Algorithms are basically the secret sauce of AI. In Python, these are used to teach computers how to decide things and solve problems. Think of them as detailed recipes for your computer to follow. From simple sorting algorithms to complex neural networks, Python has your back.
Machine Learning with Python
Machine learning (ML) is a core aspect of AI where computers learn from data. With Python, you can utilize libraries like scikit-learn to build ML models that, say, predict whether it’s going to rain tomorrow or suggest which movie to watch on Netflix. Fancy, right? Understanding datasets, training models, and evaluating their performance becomes a breeze with these tools.
Deep Learning Basics
Deep learning takes things up a notch. It's all about neural networks that mimic our brains. Using libraries like TensorFlow and Keras, Python helps in setting up these intricate models. Whether it’s recognizing images or understanding speech, Python does the heavy lifting with these libraries.
Using Libraries Effectively
Python shines here with its impressive list of libraries. For data manipulation, you have Pandas and NumPy. When it comes to visualization, Matplotlib and Seaborn are your go-to options. Knowing when and where to use these libraries is key to making your AI projects efficient and effective.
By leveraging Python’s simplicity and robust libraries, you're already halfway into the world of AI magic. Just keep experimenting and learning—it’s all about gaining hands-on experience.
Key Python Libraries for AI
Diving into AI without taking advantage of Python's libraries is like riding a bike with one wheel—you’re not going to get far! These libraries do the heavy lifting, letting you focus on solving problems, not reinventing the wheel.
TensorFlow
First up is TensorFlow. Developed by Google, it’s one of the top libraries used to create deep learning models. Its flexibility allows you to build and train models for everything from image recognition to natural language processing. Plus, it's got an active community, so you're never too far from help when you hit a snag.
PyTorch
Then there's PyTorch. It’s popular in research circles primarily because of its dynamic computation graph, which means you can change things on the fly as you go. It’s like a sandbox for testing AI model ideas. Most of the cutting-edge research you’ve heard of likely used PyTorch at some point!
Keras
If you’re only starting out and need a gentle introduction, Keras is your friend. It provides a high-level interface for TensorFlow, helping you design models with only a few lines of code. Super helpful if you want to prototype quickly and focus on the bigger picture without getting bogged down by technical details.
Scikit-learn
For traditional machine learning algorithms, there’s Scikit-learn. Whether you’re working with regression, classification, or clustering, Scikit-learn's got you covered. It integrates well with other libraries and makes your data science pipeline seamless.
Library | Main Uses |
---|---|
TensorFlow | Deep learning, flexibility in model building |
PyTorch | Dynamic graphs, research |
Keras | High-level neural networks API |
Scikit-learn | Traditional machine learning algorithms |
In short, mastering these libraries can open up a whole world of possibilities. They're constantly updated and improved, so staying on top of their developments is a good idea. So go ahead, install these, and start exploring!
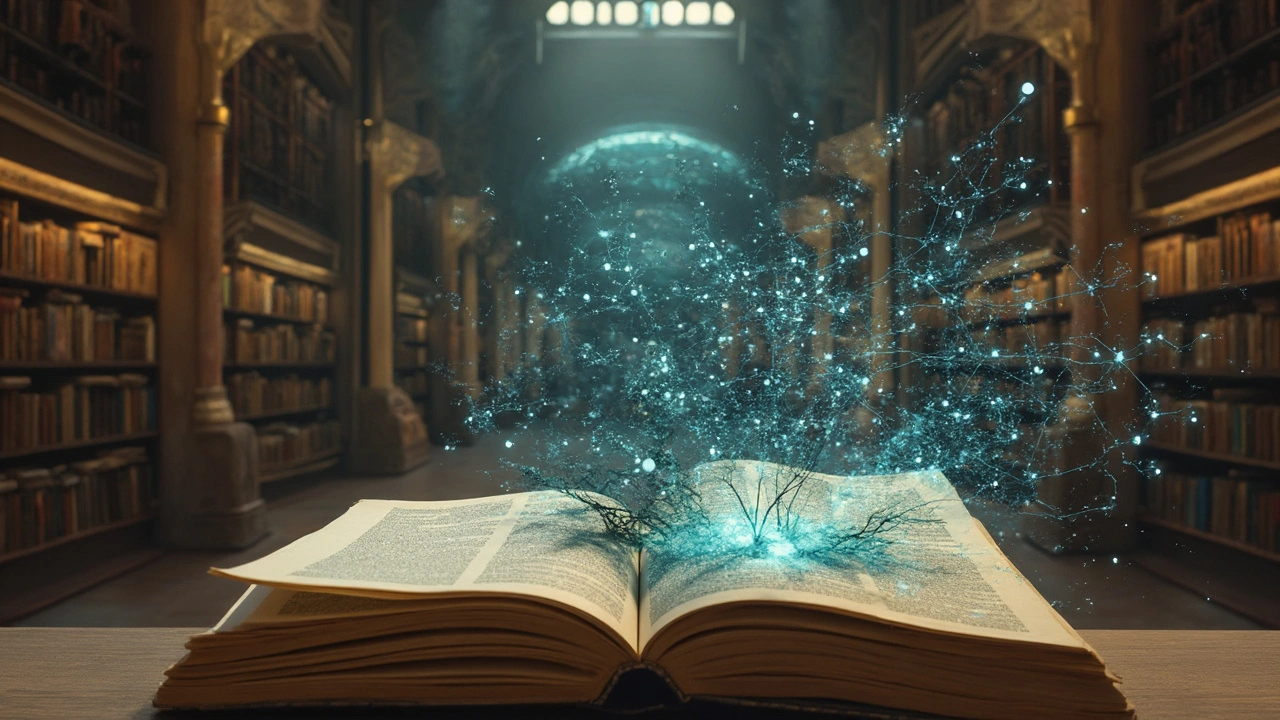
Practical Tips for Beginners
Alright, let’s get into the nitty-gritty of starting out with Python for AI. Taking those first steps can feel overwhelming, but trust me, it’s more about understanding the concepts than memorizing code.
Start Small, Think Big
It’s tempting to hop straight into developing complex AI models, but begin with the basics. Simple projects help you understand Python’s syntax and core programming principles. Consider starting with a basic weather app or a text-based calculator. These can be your playground for experimenting with code.
Learn by Doing
Forget boring theory-heavy tutorials as much as possible. Dive into coding exercises. Websites like Codecademy or LeetCode offer Python challenges that improve your skills in a hands-on way. Remember, the best way to learn programming is to write lots of code and break stuff—it’s okay to make mistakes!
Use Libraries to Your Advantage
Python is famous for its extensive range of libraries tailored for AI. Don't try reinventing the wheel. Familiarize yourself with libraries like NumPy for numerical data handling, and TensorFlow or PyTorch for building machine learning models. Explore sample projects on GitHub to see how these libraries are utilized in real-world applications.
Join Communities
Programming can feel lonely, but you’re never really alone. Python has a large and welcoming community. Join forums like Reddit’s r/learnpython, Stack Overflow, or even local meetups. There's always someone willing to help you with code issues or to share insights.
Track Your Progress
Keeping track of your learning path is key. Document the projects you work on, the problems you encounter, and how you solve them. It’s a great way to see how far you’ve come. Plus, this habit helps when you get stuck on new challenges—often, you've already solved something similar.
In summary, patience and persistence are essential when learning Python for AI. It’s a journey, not a race. Every bug fixed and every line of code written brings you one step closer to mastering AI.