Programming can feel a bit like being a detective sometimes, don't you think? You're on the hunt, searching for those pesky bugs that hide in the shadows of your code, waiting to trip you up. While coding is all about creativity and logic, debugging is where the real magic happens—that's where problems are solved.
A good place to start with debugging is understanding why bugs occur. Computers follow instructions very literally, so even the tiniest mistake can cause unexpected results. That typo or forgotten semicolon can create a ripple effect. But don't worry! Debugging is your toolkit to sleuth out these issues and smooth them over.
- Understanding Debugging
- Common Tools and Techniques
- Debugging Best Practices
- Debugging in Team Environments
Understanding Debugging
Debugging is often the unsung hero in the world of software development. It's the process of identifying and removing errors or bugs from code, making sure that the programs run as intended. Think of it like editing a book to ensure there are no typos or confusing sentences that might lead readers astray.
So, where do bugs come from? They're mostly accidental, caused by human error. When writing thousands of lines of code, it's easy for something small to slip through the cracks. That's why debugging is crucial—it helps maintain the quality and reliability of software.
Types of Bugs
Understanding different kinds of bugs can make the debugging process easier:
- Syntax errors: These occur when the code is not written correctly. The computer can't understand what you've written, much like misspelling a word.
- Logic errors: The code runs, but it doesn't do what you expect. Think of it as following a map but ending up at the wrong destination.
- Runtime errors: These happen while the program is running. It's like a sudden breakdown in the middle of a car journey.
Each type of bug requires a different approach, but knowing what you're up against is already half the battle won.
Popular Debugging Techniques
There are several techniques developers use to track down bugs:
- Print statements: This old-school method involves printing out variable values to track where things go wrong.
- Breakpoints: Set these in your code to pause execution at specific points. You can then examine variable values and see what the program is doing.
- Unit testing: Automated tests are written for small parts of the code to make sure each piece functions correctly.
Incorporating these techniques into your debugging process can help streamline bug fixes.
Common Tools and Techniques
Diving into the world of debugging without your toolkit is like rock climbing without any ropes. Thankfully, there's no shortage of code debugging tools that can turn you from a frustrated programmer into a seasoned hunter of bugs.
Debugging Tools
Let's chat about some of the big players. First up, we have debuggers. They're your best friend when you're trying to figure out where things are going south in your software development process.
- GDB (GNU Debugger): This one's a classic among C and C++ developers. It lets you see what happens when your program runs or when it crashes. A bit old-school, but super effective.
- Chrome DevTools: If you're in the web development game, this is a must-have. You can inspect HTML, view messages, and even edit CSS live.
- PyCharm Debugger: For all you Python fans, PyCharm offers an amazing built-in debugger that makes catching bugs feel almost too easy.
Techniques to Master
Highly effective debuggers also pack some neat tricks up their sleeves. Here are a few techniques that can take your bug fixing skills to the next level:
- Breakpoints: Instead of reading your code a hundred times, use breakpoints to pause execution and check the state at that moment. Simple and powerful.
- Logging: Insert print statements to see how your program flows and what values are being processed. It's the debugging equivalent of a spy camera.
- Binary Search: This isn't just for algorithms! Narrow down the source of your bug by dividing and conquering your code to pinpoint where the issue lies.
Stats You Should Know
Tool | Popularity |
---|---|
Chrome DevTools | 85% of web developers use this regularly |
Visual Studio Debugger | Ranked top for integrated development environments (IDEs) |
Keep these tools and techniques in your back pocket, and you'll find yourself navigating the debugging world with a lot more ease. It’s all about finding what works best for you and turning that bug-fixing session from a headache into an adventure.
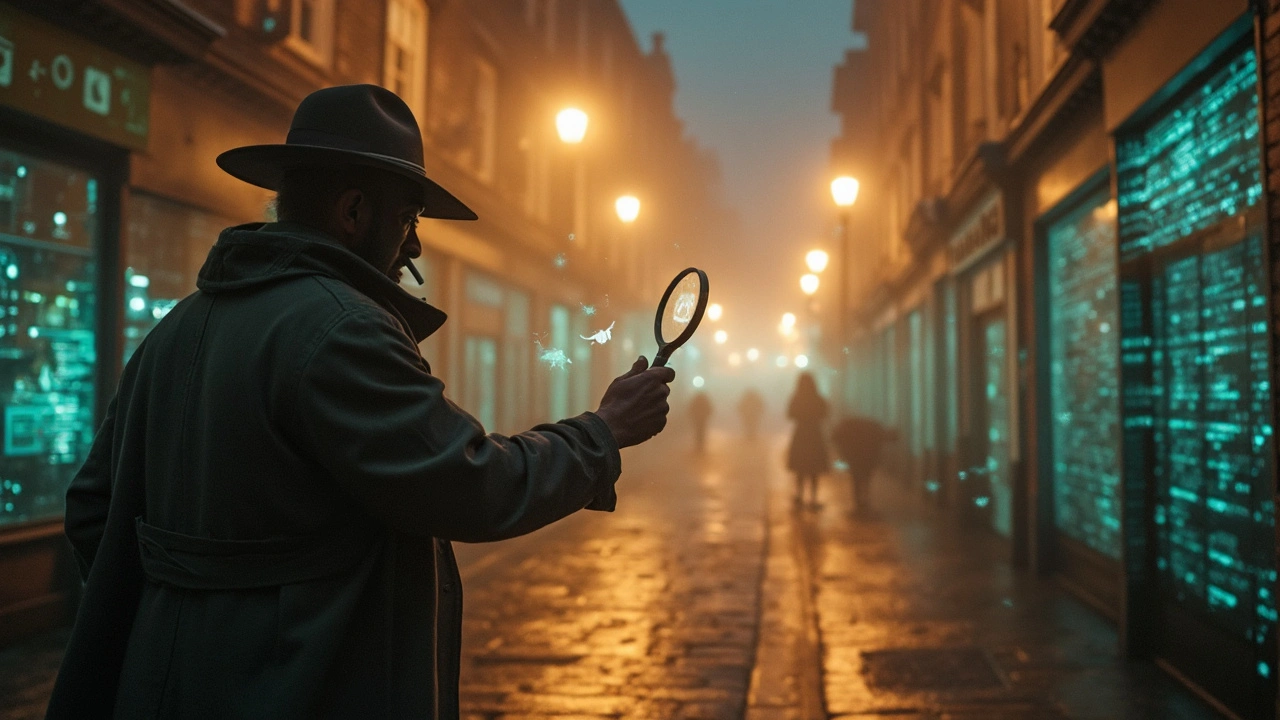
Debugging Best Practices
Getting comfortable with code debugging is essential for any developer. It can save hours of frustration and help you write better software. Let's talk about some best practices that can make debugging a breeze.
1. Understand the Problem Thoroughly
Before diving in to fix a bug, take a moment to understand what's really going wrong. Reproduce the bug yourself and note down the exact conditions under which it occurs. Often, understanding half the problem means you're halfway to the solution.
2. Use Version Control Wisely
Some find themselves in hot water by not using version control judiciously. Tools like Git allow you to see what changes have been made, making it easier to backtrack if a recent change broke something. Commit often and use clear messages; your future self will thank you!
3. Break the Problem into Smaller Parts
Tackle the issue by breaking it down into smaller, manageable pieces. This technique, often referred to as 'divide and conquer,' allows you to isolate the part of the code that is misbehaving.
4. Employ Print Statements or Logging
Adding logging is a timeless debugging trick. Print statements help you trace program execution and understand where things might be going astray. However, be sure to clean up any extraneous logs after resolving the bug.
5. Keep Calm and Debug On
Stress and frustration can cloud judgment and lead to more mistakes. Step back, relax, and come back to the problem with a fresh set of eyes if you're feeling stuck. Sometimes a break is the most effective strategy.
6. Learn and Share
Every bug you fix is a learning opportunity. Document it somewhere for future reference, and share your experiences with teammates. This way, the whole team grows together.
Practicing these habits regularly will greatly improve your debugging game. By mastering these techniques, you ensure that no bug is too big to squash!
Debugging in Team Environments
Working on a software project with a team is no easy feat. Apart from merging code, integrating different modules, and ensuring everything runs smoothly, you have to get everyone on the same page when it comes to finding and fixing bugs. Debugging in this environment brings its own set of challenges, but it can be incredibly rewarding when done right.
Collaboration is key when it comes to team-based debugging. Clear communication can save hours of time and prevent misunderstanding. Sharing a common understanding of the software development goals helps everyone aim in the same direction.
Using Debugging Tools Efficiently
There are plenty of tools out there to help teams squash bugs together. Version control systems like Git can be lifesavers when working in teams. They let you track changes, resolve conflicts, and most importantly, roll back any changes that unleashed a storm of errors. Make sure everyone on the team knows the basics at least.
Another handy tool is project management software that includes bug-tracking features, like Jira or Trello. They help keep everyone updated on what needs fixing and who's tackling which issue. This way, efforts aren't duplicated, and progress is visible to all.
Code Review Culture
Code reviews are an excellent way to catch errors early on. Having other team members look at your code can provide fresh perspectives and new ideas on how to solve issues. Frequent reviews mean that bugs are caught sooner rather than after a significant chunk of work is done. Encourage a culture where it’s safe to give and receive constructive feedback and suggestions.
Table of Commonly Used Debugging Tools in Team Environments
Tool | Purpose | Key Feature |
---|---|---|
Git | Version Control | Branching and Merging |
Jira | Project Management | Issue Tracking |
Trello | Project Management | Visual Workflow |
Managing Tensions and Conflicts
Working in a team often means different opinions on how to tackle a problem. It’s crucial to have regular meetings to discuss challenges and priorities. Encourage team members to share their problem-solving strategies and consider setting up a 'bug-fix day' where the entire focus is on bug fixing.
Ultimately, a good debugging practice in a team isn't just about technical skills; it's about teamwork, effective communication, and a shared commitment to creating quality software. The stronger the team culture around debugging, the smoother the code debugging process will be.